From Code to Creativity: The Power of Go in Language Generation
We’re diving deep into the world of Go (also known as Golang) and exploring how this powerful language can be harnessed for Natural Language Generation (NLG) and working with Language Models. Whether you’re an early-stage startup founder, a VC investor, or a tech leader, this one’s for you.
Table of Contents
Before we delve into the exciting realm of NLG and language models, let’s do a quick intro to Go. Go is an open-source programming language known for its simplicity, efficiency, and versatility. Developed by Google, it’s gained popularity for its ability to build robust and high-performance applications.
1. Natural Language Generation with Go
1.1. Generating Text with Templates
Go makes it a breeze to generate text using templates. The `text/template` and `html/template` packages allow you to define templates with placeholders and then populate them with data. This approach is ideal for generating structured text like emails, reports, or even parts of web pages.
For example, you can create a template for a marketing email:
```go package main import ( "fmt" "text/template" "os" ) func main() { templateText := "Hey {{.Name}}, check out our latest {{.Product}}!" data := struct { Name string Product string }{ Name: "John", Product: "Gizmo X", } t := template.Must(template.New("emailTemplate").Parse(templateText)) if err := t.Execute(os.Stdout, data); err != nil { fmt.Println("Error:", err) } } ```
This simple example demonstrates how Go’s templating engine can dynamically generate personalized content.
1.2. Leveraging External Libraries
When it comes to NLG and language models, Go has some great libraries at your disposal. One standout is the `gpt-3.5-turbo` library, which interfaces with GPT-3, a state-of-the-art language model. You can use it to generate human-like text with just a few lines of code.
Here’s an example of how you can use the `gpt-3.5-turbo` library:
```go package main import ( "fmt" "github.com/openai/gpt-3.5-turbo" ) func main() { apiKey := "YOUR_API_KEY" client := gpt.NewClient(apiKey) prompt := "Translate the following English text to French: 'Hello, how are you?'" response, err := client.Completions.Create(prompt, gpt.WithTemperature(0.7), gpt.WithMaxTokens(50)) if err != nil { fmt.Println("Error:", err) return } fmt.Println("Translation:", response.Choices[0].Text) } ```
By integrating external libraries like `gpt-3.5-turbo`, you can tap into advanced NLG capabilities.
2. Examples in the Wild
Let’s take a look at some real-world examples of companies and projects that have successfully used Go for NLG and language models:
- GitHub Copilot GitHub Copilot: GitHub’s AI-powered coding assistant relies on NLG and language models, including GPT-3. While GitHub Copilot’s core is built in Rust, it leverages Golang for various tasks and integrations.
- WriteSonic:WriteSonic: WriteSonic is an AI content generation tool that uses GPT-3 under the hood. Their backend, responsible for generating text, is implemented in Go, ensuring speed and efficiency.
- Face’s Transformers Transformers: Although primarily Python-based, Hugging Face’s Transformers library has a Go wrapper that allows developers to interact with pre-trained models for text generation, translation, and more.
Conclusion
So there you have it, fellow tech enthusiasts! Go is not just a language for building high-performance systems; it’s also a fantastic choice for diving into the exciting world of Natural Language Generation and language models.
Feel free to explore the external links for more in-depth insights into these real-world applications of Go in NLG. As always, stay curious, keep coding, and let Go empower your language generation adventures!
Table of Contents
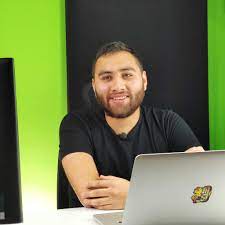
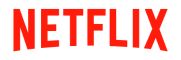