Using Go for Natural Language Processing: Text Analysis and Classification
Natural Language Processing (NLP) is a field of artificial intelligence that focuses on enabling computers to understand, interpret, and generate human language. It plays a pivotal role in various applications such as sentiment analysis, chatbots, language translation, and more. While Python is often the go-to language for NLP tasks, other programming languages like Go can also be powerful tools for text analysis and classification.
In this comprehensive guide, we’ll delve into the world of NLP using Go. We’ll explore the essential libraries, techniques, and code samples to perform text analysis and classification effectively. Whether you’re a seasoned Go developer or a newcomer, this guide will help you harness the power of Go for NLP tasks.
1. Why Use Go for NLP?
1.1. Performance and Efficiency
Go is renowned for its excellent performance and efficiency. It compiles to native machine code, which results in faster execution times compared to interpreted languages like Python. When working with large datasets or complex NLP tasks, Go’s speed can be a game-changer.
1.2. Concurrency and Parallelism
Go was designed with concurrency in mind. Its goroutines and channels make it easy to write concurrent programs, which is crucial for handling NLP tasks efficiently. You can easily parallelize tasks like text preprocessing, making Go a great choice for high-performance NLP applications.
1.3. Strong Typing
Go’s strong typing system helps catch errors at compile time, reducing the likelihood of runtime errors. This feature is particularly valuable when working with text data, as it can help ensure data integrity and consistency.
1.4. Scalability
Scalability is a critical factor in many NLP applications. Go’s simplicity and efficiency make it easier to scale your NLP projects as they grow, whether you’re building a simple sentiment analysis tool or a complex chatbot.
2. Getting Started with Go for NLP
Now that we’ve highlighted the advantages of using Go for NLP, let’s dive into the practical aspects. To get started, you’ll need a basic understanding of Go programming. If you’re new to Go, there are plenty of online resources and tutorials to help you grasp the fundamentals.
3. Setting Up Your Go Environment
Before we can start with NLP, you need to set up a Go environment. Follow these steps to get up and running:
- Install Go: Download and install Go from the official website. Make sure to add Go’s bin directory to your system’s PATH variable.
- Verify Installation: Open a terminal and run the following command to ensure Go is installed correctly:
shell go version
You should see the installed Go version displayed.
4. Essential Libraries for NLP in Go
Go has a growing ecosystem of libraries for NLP tasks. Here are some essential libraries that you’ll find incredibly useful:
4.1. GoNLP
GoNLP is a popular library for machine learning and NLP tasks in Go. It provides a wide range of tools for text analysis, classification, and feature engineering. To install GoNLP, use the following command:
shell go get github.com/sjwhitworth/golearn
4.2. Golang-NLP
Golang-NLP is a library that focuses on text processing and NLP in Go. It includes functionalities for tokenization, part-of-speech tagging, named entity recognition, and more. Install Golang-NLP using:
shell go get github.com/jdkato/prose
4.3. Gorgonia
Gorgonia is a machine learning library for Go. While it’s not exclusive to NLP, it can be immensely helpful for implementing neural networks and deep learning models for text analysis. To install Gorgonia, use:
shell go get gorgonia.org/gorgonia
Now that you have the essential libraries in place, let’s explore some fundamental NLP tasks.
5. Text Preprocessing in Go
Text preprocessing is a crucial step in NLP. It involves cleaning and transforming raw text data into a format suitable for analysis. Here’s how you can perform common text preprocessing tasks in Go:
5.1. Tokenization
Tokenization involves splitting text into individual words or tokens. GoNLP provides a simple way to tokenize text:
go package main import ( "fmt" "github.com/sjwhitworth/golearn/base" "github.com/sjwhitworth/golearn/feature_extraction" ) func main() { text := "Tokenization is an essential NLP task." tokens := feature_extraction.NewWordExtractor() tokens.Tokenize(text) fmt.Println(tokens.GetTokens()) }
This code snippet tokenizes the input text and prints the tokens.
5.2. Removing Stop Words
Stop words are common words like “the,” “and,” “is,” etc., that don’t carry much meaning and are often removed during text analysis. You can use GoNLP to remove stop words:
go package main import ( "fmt" "github.com/sjwhitworth/golearn/base" "github.com/sjwhitworth/golearn/feature_extraction" ) func main() { text := "This is an example sentence with some stop words." tokens := feature_extraction.NewWordExtractor() tokens.Tokenize(text) filteredTokens := feature_extraction.RemoveStopWords(tokens.GetTokens()) fmt.Println(filteredTokens) }
This code snippet removes stop words from the input text.
5.3. Stemming and Lemmatization
Stemming and lemmatization are techniques used to reduce words to their root forms. GoNLP provides support for stemming and lemmatization:
go package main import ( "fmt" "github.com/jdkato/prose" ) func main() { text := "Running and ran are both forms of run." doc, _ := prose.NewDocument(text) for _, token := range doc.Tokens() { fmt.Printf("Token: %s, Lemma: %s\n", token.Text, token.Lemma) } }
This code snippet demonstrates how to perform stemming and lemmatization using Golang-NLP.
6. Text Classification with Go
Text classification is a common NLP task where you categorize text documents into predefined classes or categories. Here’s how you can perform text classification in Go using GoNLP:
6.1. Data Preparation
First, you need a dataset for text classification. Suppose you have a CSV file with text data and corresponding labels. You can load this data using GoNLP:
go package main import ( "github.com/sjwhitworth/golearn/base" "github.com/sjwhitworth/golearn/linear_models" ) func main() { // Load your dataset from a CSV file rawData, err := base.ParseCSVToInstances("dataset.csv", true) if err != nil { panic(err) } // Define the feature and label columns rawData.SpecifyAttributes([]base.Attribute{ // Define your features here }) // Initialize a classifier (e.g., logistic regression) classifier := linear_models.NewLogisticRegression("l2", 1.0, 1000) // Train the classifier classifier.Fit(rawData) }
In this code, you load your dataset, specify the features and labels, and initialize a classifier.
6.2. Feature Extraction
To perform text classification, you need to convert text data into numerical features. You can use techniques like TF-IDF (Term Frequency-Inverse Document Frequency) for feature extraction:
go // Convert text data to TF-IDF features tfidf := feature_extraction.NewTfidfVectorizer() tfidf.Fit(rawData) tfidf.Transform(rawData)
6.3. Training and Evaluation
Once you have prepared your data and extracted features, you can train and evaluate your classifier:
go package main import ( "github.com/sjwhitworth/golearn/evaluation" ) func main() { // Split data into training and testing sets trainData, testData := base.InstancesTrainTestSplit(rawData, 0.8) // Train the classifier on the training data classifier.Fit(trainData) // Make predictions on the test data predictions := classifier.Predict(testData) // Evaluate the classifier's performance confusionMat := evaluation.GetConfusionMatrix(testData, predictions) accuracy := evaluation.GetAccuracy(confusionMat) fmt.Printf("Accuracy: %.2f%%\n", accuracy*100) }
This code splits your data into training and testing sets, trains the classifier, makes predictions, and evaluates its accuracy.
Conclusion
In this guide, we’ve explored how to use Go for Natural Language Processing, focusing on text analysis and classification. Go’s performance, concurrency support, strong typing, and scalability make it a valuable choice for NLP tasks.
We covered essential libraries for NLP in Go, including GoNLP, Golang-NLP, and Gorgonia. You learned about text preprocessing techniques such as tokenization, stop word removal, and stemming/lemmatization. Additionally, we walked through the process of text classification, from data preparation to feature extraction, training, and evaluation.
With this foundation, you can leverage Go to tackle a wide range of NLP projects, from sentiment analysis to chatbots and beyond. As you continue to explore the capabilities of Go in NLP, you’ll discover how it can empower you to build efficient and scalable language-processing applications. So, roll up your sleeves, fire up your Go environment, and start transforming text data into valuable insights with Go and NLP. Happy coding!
Table of Contents
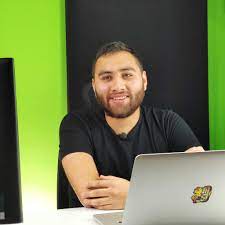
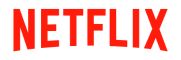