Introduction to Go’s Net/http Package: Creating Web Servers and Clients
Go, often referred to as Golang, is a powerful and efficient programming language developed by Google. It has gained significant popularity in recent years due to its simplicity, performance, and robust standard library. One of the key strengths of Go is its ability to handle web-related tasks efficiently, thanks in no small part to the net/http package.
In this blog, we will explore the fundamentals of Go’s net/http package, demonstrating how to create web servers and clients using this package. Whether you are a beginner or an experienced developer, this guide will provide you with the knowledge and examples you need to get started with web development in Go.
1. What is the net/http Package?
The net/http package is a part of Go’s standard library and is designed to handle HTTP requests and responses. It provides a set of powerful tools and abstractions for building both HTTP servers and clients, making it a cornerstone for web development in Go.
2. Importing the net/http Package
Before we dive into creating web servers and clients, we need to import the net/http package. You can do this with the following import statement:
go import ( "net/http" )
This import statement makes all the functions and types from the net/http package available for use in your Go program.
3. Creating a Simple HTTP Server
Now that we have imported the net/http package, let’s start by creating a simple HTTP server. We will create an HTTP server that responds with a “Hello, World!” message when accessed via a web browser.
3.1. Creating a Basic HTTP Handler Function
In Go, HTTP servers are configured by defining handler functions. A handler function is an ordinary Go function that conforms to the http.Handler interface. The http.Handler interface requires the implementation of a single method: ServeHTTP. Here’s a basic example of an HTTP handler function:
go func helloHandler(w http.ResponseWriter, r *http.Request) { // Write a response to the client w.Write([]byte("Hello, World!")) }
In the helloHandler function, we receive two parameters:
- w of type http.ResponseWriter: This is used to write the response that will be sent back to the client.
- r of type *http.Request: This represents the incoming HTTP request and contains information about the request, such as the HTTP method, headers, and URL.
3.2. Registering the Handler Function
To make our helloHandler function respond to incoming HTTP requests, we need to register it with the HTTP server. We do this using the http.HandleFunc function, which associates a path (in this case, “/hello”) with our handler function:
go
func main() {
http.HandleFunc(“/hello”, helloHandler)
http.ListenAndServe(“:8080”, nil)
}
go func main() { http.HandleFunc("/hello", helloHandler) http.ListenAndServe(":8080", nil) }
In the main function, we use http.HandleFunc to associate the “/hello” path with our helloHandler function. We then use http.ListenAndServe to start the HTTP server, specifying that it should listen on port 8080. The second parameter (nil) means that we’re using the default HTTP server settings.
3.3. Running the HTTP Server
To run the HTTP server, simply execute your Go program. When you open a web browser and navigate to http://localhost:8080/hello, you should see the “Hello, World!” message displayed in your browser.
Congratulations! You’ve just created a basic HTTP server in Go. Now, let’s take a closer look at some important concepts and features of the net/http package.
4. HTTP Server Configuration
In the previous example, we created a basic HTTP server with minimal configuration. However, the net/http package provides extensive customization options to tailor your server’s behavior according to your needs.
4.1. Handling Different HTTP Methods
By default, the http.HandleFunc function responds to all HTTP methods (GET, POST, PUT, DELETE, etc.). If you want to handle specific HTTP methods, you can use http.HandlerFunc instead. Here’s an example of handling a POST request:
go func postHandler(w http.ResponseWriter, r *http.Request) { if r.Method == http.MethodPost { // Handle the POST request // ... } else { w.WriteHeader(http.StatusMethodNotAllowed) w.Write([]byte("Method not allowed")) } } func main() { http.HandleFunc("/post", postHandler) http.ListenAndServe(":8080", nil) }
In this example, we check the HTTP method using r.Method and respond accordingly.
4.2. Customizing the HTTP Response
The http.ResponseWriter interface allows you to customize the HTTP response sent to the client. You can set custom headers, status codes, and more. Here’s an example of setting a custom header and status code:
go func customResponseHandler(w http.ResponseWriter, r *http.Request) { w.Header().Set("Content-Type", "application/json") w.WriteHeader(http.StatusOK) w.Write([]byte(`{"message": "Custom response"}`)) } func main() { http.HandleFunc("/custom", customResponseHandler) http.ListenAndServe(":8080", nil) }
In this example, we set the “Content-Type” header to “application/json” and the status code to 200 (OK) before writing the response body.
4.3. Serving Static Files
The http.FileServer function is a handy tool for serving static files, such as HTML, CSS, JavaScript, and images. Here’s how you can serve static files from a “static” directory:
go func main() { http.Handle("/static/", http.StripPrefix("/static/", http.FileServer(http.Dir("static")))) http.ListenAndServe(":8080", nil) }
In this example, we use http.StripPrefix to remove the “/static/” prefix from URLs, making it transparent to the client. Files from the “static” directory will be served when requested.
5. Creating an HTTP Client
In addition to creating HTTP servers, the net/http package also provides tools for creating HTTP clients. You can use these tools to make HTTP requests to external servers and consume APIs. Let’s explore how to create a basic HTTP client in Go.
5.1. Making a GET Request
To make a simple GET request, you can use the http.Get function:
go package main import ( "fmt" "net/http" "io/ioutil" ) func main() { response, err := http.Get("https://jsonplaceholder.typicode.com/posts/1") if err != nil { fmt.Println("Error:", err) return } defer response.Body.Close() body, err := ioutil.ReadAll(response.Body) if err != nil { fmt.Println("Error:", err) return } fmt.Println(string(body)) }
In this example, we use http.Get to send a GET request to the specified URL (“https://jsonplaceholder.typicode.com/posts/1”). We then read and print the response body.
5.2. Making a POST Request
To make a POST request with a JSON payload, you can use the http.Post function:
go package main import ( "fmt" "net/http" "bytes" "encoding/json" ) func main() { payload := map[string]interface{}{ "title": "foo", "body": "bar", "userId": 1, } payloadBytes, _ := json.Marshal(payload) response, err := http.Post("https://jsonplaceholder.typicode.com/posts", "application/json", bytes.NewBuffer(payloadBytes)) if err != nil { fmt.Println("Error:", err) return } defer response.Body.Close() // Handle the response as needed }
In this example, we create a JSON payload, marshal it into bytes, and send it as the request body in a POST request.
6. Middleware in net/http
Middleware is a powerful concept in web development that allows you to intercept and manipulate HTTP requests and responses before they reach your handlers or after they leave them. Go’s net/http package provides a straightforward way to implement middleware.
6.1. Creating Middleware Functions
Middleware functions are functions that wrap HTTP handlers, allowing you to perform actions before and after the handler executes. A middleware function takes an http.Handler as a parameter and returns a new http.Handler. Here’s a simple logging middleware:
go func loggingMiddleware(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { // Log the incoming request fmt.Println("Request received:", r.Method, r.URL.Path) // Call the next handler in the chain next.ServeHTTP(w, r) // Log the response status code fmt.Println("Response status:", w.Status()) }) }
In this example, loggingMiddleware wraps the next handler, logging the incoming request and the response status code.
6.2. Using Middleware
To use middleware in your HTTP server, simply wrap your handlers with the middleware functions. Here’s an example that uses the logging middleware:
go func main() { // Create a new router router := http.NewServeMux() // Attach middleware to the router router.Handle("/", loggingMiddleware(http.HandlerFunc(helloHandler))) // Start the server http.ListenAndServe(":8080", router) }
In this example, the helloHandler is wrapped with the loggingMiddleware using http.HandlerFunc. Now, every incoming request will be logged before and after the handler is executed.
Conclusion
Go’s net/http package is a versatile and powerful tool for building web servers and clients. In this blog, we’ve covered the basics of creating an HTTP server, configuring it, serving static files, and creating an HTTP client. Additionally, we explored the concept of middleware and how it can be used to enhance your web applications.
With the knowledge and examples provided in this guide, you are well-equipped to start developing web applications and APIs in Go. As you delve deeper into web development with Go, you’ll discover even more features and techniques offered by the net/http package, allowing you to build robust and efficient web applications. Happy coding!
Table of Contents
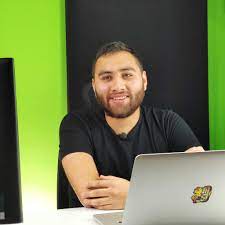
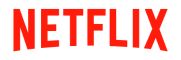