Introduction to Go’s Net/HTTP Package: HTTP Client and Server
When it comes to building web applications and services in the Go programming language, the Net/HTTP package is a fundamental tool in your arsenal. This package provides everything you need to create robust HTTP clients and servers, making it a core component of Go’s standard library.
In this comprehensive guide, we will explore the Net/HTTP package in depth, covering the basics, key concepts, and practical examples. Whether you’re a seasoned Go developer or just getting started, this introduction will equip you with the knowledge and skills to harness the full potential of Go’s Net/HTTP package.
1. Understanding HTTP in Go
1.1. HTTP Basics
Before delving into Go’s Net/HTTP package, let’s briefly review the basics of HTTP (Hypertext Transfer Protocol). HTTP is the foundation of data communication on the World Wide Web. It is a protocol that enables the transfer of data between a client (typically a web browser) and a server.
HTTP is inherently a stateless protocol, which means that each request from a client to a server is independent and doesn’t retain any information about previous requests. This statelessness is a fundamental principle of HTTP.
1.2. Go’s Approach to HTTP
Go’s standard library includes the Net/HTTP package, which provides a comprehensive set of tools for working with HTTP. This package is designed with simplicity and flexibility in mind, making it easy to build both HTTP clients and servers.
One of Go’s strengths is its focus on clean and efficient code, and the Net/HTTP package is no exception. It encourages developers to write clear and concise code while providing powerful features for handling HTTP requests and responses.
2. Building an HTTP Server
2.1. Creating a Simple HTTP Server
To get started with Go’s Net/HTTP package, let’s build a basic HTTP server that responds to incoming requests. Here’s a minimal example:
go package main import ( "fmt" "net/http" ) func helloHandler(w http.ResponseWriter, r *http.Request) { fmt.Fprintln(w, "Hello, World!") } func main() { http.HandleFunc("/hello", helloHandler) http.ListenAndServe(":8080", nil) }
In this example, we define an http.HandlerFunc called helloHandler that writes “Hello, World!” as the response. We then use http.HandleFunc to map the /hello URL path to this handler. Finally, we start the HTTP server on port 8080 using http.ListenAndServe.
2.2. Handling Requests and Responses
HTTP handlers in Go are functions that take two arguments: an http.ResponseWriter and an *http.Request. The http.ResponseWriter is used to construct the HTTP response, while the *http.Request contains information about the incoming HTTP request.
You can access various properties of the request, such as the HTTP method, headers, query parameters, and request body, via the *http.Request object. Likewise, you can write the response headers and body using the http.ResponseWriter.
Let’s enhance our previous example to handle different HTTP methods:
go func handleRequest(w http.ResponseWriter, r *http.Request) { switch r.Method { case http.MethodGet: fmt.Fprintln(w, "GET Request") case http.MethodPost: fmt.Fprintln(w, "POST Request") default: http.Error(w, "Method not allowed", http.StatusMethodNotAllowed) } }
In this updated code, the handleRequest function checks the HTTP method of the incoming request and responds accordingly. If it’s a GET request, it prints “GET Request”; if it’s a POST request, it prints “POST Request.” For other methods, it returns a “Method not allowed” error with a 405 status code.
We can map this handler to a specific URL path just like before.
2.3. Routing Requests
In real-world applications, you’ll often need more advanced routing to handle different routes and URL patterns. The gorilla/mux package is a popular choice for handling routing in Go.
Here’s an example of using gorilla/mux for routing:
go package main import ( "fmt" "net/http" "github.com/gorilla/mux" ) func main() { r := mux.NewRouter() r.HandleFunc("/hello", helloHandler).Methods(http.MethodGet) r.HandleFunc("/greet/{name}", greetHandler).Methods(http.MethodGet) http.Handle("/", r) http.ListenAndServe(":8080", nil) }
In this example, we use mux.NewRouter() to create a router, and then we define routes using r.HandleFunc. The second route includes a URL parameter ({name}) that can be accessed within the handler function. http.Handle(“/”, r) sets the router as the request handler.
2.4. Middleware
Middleware is a powerful concept in Go’s Net/HTTP package. Middleware functions can intercept and process requests and responses before they reach the final handler. This allows you to implement common functionality, such as logging, authentication, and authorization, in a modular way.
Here’s an example of a simple logging middleware:
go func loggingMiddleware(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { // Log the incoming request log.Printf("Request from %s: %s %s", r.RemoteAddr, r.Method, r.URL) // Call the next handler in the chain next.ServeHTTP(w, r) }) }
You can use this middleware to log requests by wrapping your handlers like this:
go http.Handle("/", loggingMiddleware(r))
This is just one example of what you can achieve with middleware in Go’s Net/HTTP package. You can create middleware functions for various purposes, making your HTTP server code more organized and maintainable.
3. Making HTTP Requests with Go
3.1. Creating an HTTP Client
In addition to building HTTP servers, Go’s Net/HTTP package is excellent for creating HTTP clients. Whether you need to consume external APIs or make requests to other services, Go provides a straightforward way to do so.
Here’s how you can create a basic HTTP client:
go package main import ( "fmt" "net/http" "io/ioutil" ) func main() { // Create an HTTP client client := &http.Client{} // Define the URL to request url := "https://jsonplaceholder.typicode.com/posts/1" // Send an HTTP GET request resp, err := client.Get(url) if err != nil { fmt.Println("Error:", err) return } defer resp.Body.Close() // Read the response body body, err := ioutil.ReadAll(resp.Body) if err != nil { fmt.Println("Error:", err) return } // Print the response fmt.Println("Response:", string(body)) }
In this example, we create an http.Client and use it to send an HTTP GET request to a specified URL. We then read the response body and print it to the console.
3.2. Sending GET and POST Requests
Go’s Net/HTTP package supports various HTTP methods, including GET, POST, PUT, DELETE, and more. Here’s how you can send both GET and POST requests:
GET Request
go func sendGetRequest() { // ... resp, err := client.Get(url) // ... }
POST Request
go func sendPostRequest() { // Create a JSON payload payload := []byte(`{"title": "New Post", "body": "This is a new post."}`) // Send an HTTP POST request resp, err := client.Post(url, "application/json", bytes.NewBuffer(payload)) // ... }
When sending a POST request, you can specify the request body and set the content type. In the example above, we send a JSON payload with the “application/json” content type.
3.3. Handling HTTP Responses
Once you’ve sent an HTTP request, you need to handle the HTTP response. In the previous examples, we used ioutil.ReadAll to read the response body. However, the http.Response struct provides more information about the response, such as headers and status codes.
go // ... // Check the response status code if resp.StatusCode == http.StatusOK { // Request was successful // ... } else { // Request failed // ... }
You can also access response headers using resp.Header.
3.4. Error Handling
When making HTTP requests, it’s essential to handle errors gracefully. Network issues, server problems, or incorrect URLs can lead to errors. Always check for errors in your code and handle them appropriately.
4. Concurrency and Goroutines
Go is well-known for its built-in support for concurrency through Goroutines. You can harness Goroutines to perform multiple HTTP requests concurrently, improving the performance of your applications.
4.1. Leveraging Goroutines for Concurrent Requests
Let’s say you need to make several HTTP requests to different URLs concurrently. You can use Goroutines to achieve this efficiently:
go package main import ( "fmt" "net/http" "sync" ) func fetchURL(url string, wg *sync.WaitGroup) { defer wg.Done() resp, err := http.Get(url) if err != nil { fmt.Printf("Error fetching %s: %s\n", url, err) return } defer resp.Body.Close() // Process the response here // ... } func main() { urls := []string{"https://example.com", "https://example.org", "https://example.net"} var wg sync.WaitGroup for _, url := range urls { wg.Add(1) go fetchURL(url, &wg) } // Wait for all Goroutines to finish wg.Wait() }
In this example, we define a fetchURL function to make HTTP requests concurrently using Goroutines. The sync.WaitGroup helps us wait for all Goroutines to finish before exiting the main function.
4.2. Managing Concurrency
While Goroutines are a powerful tool, it’s crucial to manage concurrency effectively to avoid issues like resource contention and excessive resource usage. Here are some tips for managing concurrency in Go’s Net/HTTP applications:
- Use a pool of Goroutines to limit the number of concurrent requests.
- Implement rate limiting to control the rate at which requests are made.
- Be mindful of shared resources and use appropriate synchronization mechanisms when needed.
- Monitor resource usage and performance to identify bottlenecks.
4.3. Best Practices
When working with Go’s Net/HTTP package and concurrency, consider the following best practices:
- Always handle errors returned by HTTP requests.
- Use Goroutines and channels for concurrent tasks.
- Implement timeouts for HTTP requests to prevent blocking indefinitely.
- Profile and optimize your code to ensure efficient resource utilization.
- Write tests to validate the correctness of your HTTP handlers and clients.
5. Advanced Topics
As you become more proficient with Go’s Net/HTTP package, you may encounter advanced topics and scenarios. Here are a few areas to explore:
5.1. TLS/SSL and HTTPS
Securing your HTTP communication is vital, especially when dealing with sensitive data. Go provides excellent support for TLS/SSL and HTTPS, allowing you to encrypt your HTTP traffic.
To enable HTTPS in your Go HTTP server, you’ll need an SSL/TLS certificate and private key. You can configure your server to use them like this:
go http.ListenAndServeTLS(":443", "cert.pem", "key.pem", nil)
5.2. Authentication and Authorization
Implementing authentication and authorization in your HTTP applications is essential for securing your resources. Go provides libraries and packages for handling authentication, including JWT (JSON Web Tokens) and OAuth2.
5.3. WebSockets
For real-time communication between clients and servers, consider using WebSockets. Go’s gorilla/websocket package simplifies WebSocket handling and allows you to build interactive applications.
5.4. Testing HTTP Handlers
Writing tests for your HTTP handlers is crucial to ensure that your code behaves as expected. Go’s testing framework makes it easy to write unit tests for your HTTP server and client code.
Conclusion
In this comprehensive introduction to Go’s Net/HTTP package, we’ve covered the basics of building HTTP servers and clients, handling requests and responses, leveraging concurrency with Goroutines, and delved into advanced topics like TLS/SSL, authentication, and WebSockets. Armed with this knowledge, you’re well-equipped to create robust and performant web applications and services in the Go programming language.
The Net/HTTP package’s simplicity and flexibility, combined with Go’s built-in support for concurrency, make it a powerful tool for building web applications that can handle high traffic loads efficiently. As you continue your journey with Go, don’t hesitate to explore further and experiment with different patterns and libraries to unlock the full potential of Go’s HTTP capabilities. Happy coding!
Table of Contents
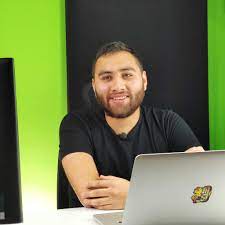
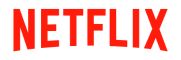