Email Automation Made Easy: Go’s net/smtp Unleashed
In the fast-paced world of tech startups and venture capital, effective communication is key. One essential aspect of communication is sending emails, and in this article, we’ll explore how to do just that using Go’s `net/smtp` package. Whether you’re a startup founder, a VC investor, or a tech leader, knowing how to send emails programmatically can be a valuable skill.
Table of Contents
1. Why Use Go for Sending Emails?
Before we dive into the specifics of the `net/smtp` package, let’s briefly discuss why Go is a great choice for sending emails in the first place:
- Concurrency: Go’s built-in support for concurrency makes it efficient for handling multiple email sending tasks simultaneously.
- Simplicity: Go’s clean and minimalistic syntax makes it easy to write and maintain email-sending code.
- Performance: Go is known for its excellent performance, which is crucial when you need to send a large number of emails quickly.
Now, let’s get into the nitty-gritty of using Go’s `net/smtp` package.
2. Importing the `net/smtp` Package
To start sending emails with Go, you’ll need to import the `net/smtp` package. Add the following import statement to your code:
```go import ( "net/smtp" ) ```
3. Sending an Email
Sending an email with Go involves several steps, including establishing a connection to your SMTP server, authenticating, and composing the email message. Here’s an example of how to do it:
```go package main import ( "net/smtp" "log" ) func main() { // Define SMTP server and authentication details smtpServer := "smtp.example.com" smtpPort := "587" smtpUsername := "your_username" smtpPassword := "your_password" // Compose the email message from := "your_email@example.com" to := []string{"recipient@example.com"} subject := "Hello, Go!" message := "This is a test email sent from Go." // Connect to the SMTP server auth := smtp.PlainAuth("", smtpUsername, smtpPassword, smtpServer) err := smtp.SendMail(smtpServer+":"+smtpPort, auth, from, to, []byte(message)) if err != nil { log.Fatal(err) } log.Println("Email sent successfully!") } ```
In this example, we specify the SMTP server details, authenticate using your credentials, and then use the `smtp.SendMail` function to send the email.
4. External Resources
Here are some external resources that can provide further insights into using Go’s `net/smtp` package:
- Official Go Documentation on `net/smtp` – https://pkg.go.dev/net/smtp
- Sending Email with Go by Todd McLeod – https://www.youtube.com/watch?v=4NgkI4y9XMw– A video tutorial covering the basics of sending email in Go.
- GitHub Repository: Gomail – https://github.com/go-gomail/gomail – A popular third-party package for sending emails in Go, built on top of `net/smtp`.
Conclusion
Sending emails programmatically in Go using the `net/smtp` package is a powerful capability, especially for startup founders, VC investors, and tech leaders who want to automate communication tasks. With Go’s simplicity and performance, you can ensure efficient email delivery for your important messages.
Start experimenting with the `net/smtp` package, and you’ll soon discover how Go can streamline your email-sending process.
Table of Contents
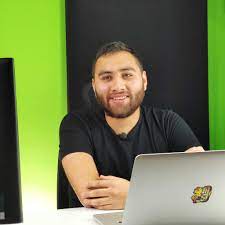
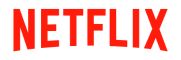