Using Go for Network Programming: Socket Communication and Protocols
In the rapidly evolving landscape of software development, the need for robust network communication has become more critical than ever. Whether it’s building web servers, microservices, or distributed systems, understanding network programming is a crucial skill. One language that shines in this domain is Go, also known as Golang. With its simplicity, performance, and built-in support for concurrency, Go is an excellent choice for creating efficient and reliable network applications. In this blog post, we will delve into the world of network programming using Go, exploring socket communication and protocols.
1. Introduction to Network Programming with Go
1.1. Understanding Network Programming
Network programming involves creating software that communicates over computer networks, enabling data exchange between devices. This is the foundation of applications like web servers, instant messaging, online gaming, and more.
1.2. Why Go for Network Programming?
Go’s simplicity and performance make it an ideal choice for network programming. Its standard library provides powerful tools for building network applications, and its concurrency features allow you to handle multiple connections efficiently.
2. Basics of Socket Communication
2.1. What are Sockets?
Sockets are endpoints for sending or receiving data across a computer network. They provide a standard interface for network communication. Sockets can be of two types: stream sockets (TCP) and datagram sockets (UDP).
2.2. Socket Types: Stream vs. Datagram
Stream sockets provide a reliable, ordered, and error-checked connection suitable for applications like file transfers and email. Datagram sockets, on the other hand, offer connectionless communication, making them suitable for tasks like streaming media or real-time gaming.
2.3. Go’s net Package
Go’s standard library includes the net package, which provides essential functions and types for networking. It offers interfaces for creating network listeners, establishing connections, and working with IP addresses and ports.
3. Building a Simple TCP Server
3.1. Importing the Required Packages
To start building a TCP server in Go, import the necessary packages:
go import ( "fmt" "net" )
3.2. Creating a Listener
A TCP server listens for incoming connections on a specific port. Here’s how you can create a listener:
go func main() { listener, err := net.Listen("tcp", ":8080") if err != nil { fmt.Println("Error:", err) return } defer listener.Close() fmt.Println("Server listening on port 8080") }
3.3. Handling Connections
Once a connection is established, the server can accept it and start handling requests:
go for { conn, err := listener.Accept() if err != nil { fmt.Println("Error:", err) continue } go handleConnection(conn) }
3.4. Sending and Receiving Data
In the handleConnection function, you can read and write data to the client:
go func handleConnection(conn net.Conn) { defer conn.Close() // Read data from the client buffer := make([]byte, 1024) n, err := conn.Read(buffer) if err != nil { fmt.Println("Error:", err) return } // Process the received data data := buffer[:n] fmt.Printf("Received: %s\n", data) // Send a response to the client response := []byte("Hello from the server") _, err = conn.Write(response) if err != nil { fmt.Println("Error:", err) } }
4. UDP Communication in Go
4.1. Understanding UDP
User Datagram Protocol (UDP) offers connectionless communication, suitable for scenarios where speed is crucial and data reliability is less critical.
4.2. Creating a UDP Server
Creating a UDP server in Go is straightforward:
go func main() { addr := net.UDPAddr{ Port: 8080, IP: net.ParseIP("127.0.0.1"), } conn, err := net.ListenUDP("udp", &addr) if err != nil { fmt.Println("Error:", err) return } defer conn.Close() fmt.Println("UDP Server listening on port 8080") }
4.3. Sending and Receiving UDP Datagrams
Handling UDP communication involves sending and receiving datagrams:
go buffer := make([]byte, 1024) n, addr, err := conn.ReadFromUDP(buffer) if err != nil { fmt.Println("Error:", err) return } fmt.Printf("Received from %s: %s\n", addr, buffer[:n]) response := []byte("Hello from UDP server") _, err = conn.WriteToUDP(response, addr) if err != nil { fmt.Println("Error:", err) }
5. Working with Protocols
5.1. What are Protocols?
Protocols define rules for communication between devices. Go supports various protocols, including HTTP, HTTPS, JSON-RPC, and more.
5.2. HTTP and HTTPS in Go
Go’s net/http package enables you to create HTTP servers and clients easily. Example of a simple HTTP server:
go func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) { fmt.Fprint(w, "Hello, World!") }) http.ListenAndServe(":8080", nil) }
5.3. JSON-RPC Protocol
JSON-RPC is a remote procedure call protocol encoded in JSON. You can implement JSON-RPC servers and clients in Go using libraries like github.com/gorilla/rpc/jsonrpc.
6. Error Handling and Concurrency
Dealing with Errors in Network Programming
Network programming comes with various potential errors, such as connection failures or data corruption. Proper error handling ensures your application remains robust.
6.1. Leveraging Goroutines for Concurrency
Go’s goroutines allow you to handle multiple connections concurrently, enhancing the efficiency of your network applications. Use the sync package to manage synchronization.
6.2. Managing Multiple Connections
When dealing with multiple clients, consider using a connection pool or a similar strategy to efficiently manage resources and connections.
7. Case Study: Building a Chat Application
Designing the Application
Imagine creating a simple chat application. Clients connect to a server, send messages, and receive messages from other clients.
7.1. Implementing the Server
The server needs to manage connections, handle incoming messages, and broadcast messages to all connected clients. Goroutines can help achieve this efficiently.
7.2. Developing the Client Side
Clients establish a connection to the server and interact with it using the command-line interface. The client can send messages and display received messages.
7.3. Handling Messages and Concurrency
To ensure messages are delivered to all clients, the server maintains a list of active connections and broadcasts messages to each one.
8. Security Considerations in Network Programming
8.1. Encryption and TLS
When transmitting sensitive data over networks, encryption is vital. Go’s crypto/tls package provides support for TLS encryption.
8.2. Input Validation and Sanitization
Ensure that user inputs are validated and sanitized to prevent security vulnerabilities like SQL injection or cross-site scripting (XSS).
8.3. Protecting Against Common Attacks
Network applications are susceptible to attacks like Distributed Denial of Service (DDoS) and Man-in-the-Middle (MitM). Implement security measures to safeguard your application.
Conclusion
In conclusion, Go offers a versatile and powerful platform for network programming, making it an excellent choice for developing efficient and reliable network applications. From socket communication to handling protocols and ensuring security, Go’s standard library and concurrency support provide all the tools you need to create robust network applications that can scale and perform under various conditions. By mastering the concepts and techniques outlined in this blog post, you’ll be well-equipped to venture into the exciting world of network programming using Go.
Table of Contents
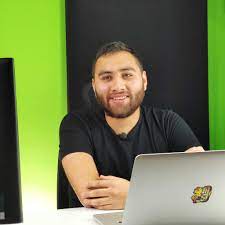
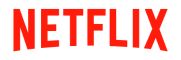