Optimizing Go Applications: Profiling and Performance Tuning
Go, also known as Golang, is a versatile and powerful programming language that has gained popularity for its simplicity, performance, and concurrency support. While Go is known for being fast “out of the box,” there are always opportunities to further optimize your Go applications for even better performance. In this blog, we’ll explore profiling techniques and performance tuning strategies to help you make your Go code lightning-fast.
1. Why Optimize Your Go Applications?
Before we dive into the nitty-gritty of profiling and performance tuning, let’s discuss why it’s essential to optimize your Go applications.
1.1. Improved User Experience
Optimizing your Go application means faster response times, smoother interactions, and an overall better user experience. Whether you’re building a web server, a command-line tool, or a backend service, speed matters.
1.2. Resource Efficiency
Efficient Go code consumes fewer system resources, such as CPU and memory. This not only reduces operational costs but also allows your application to handle more concurrent requests without slowing down.
1.3. Competitive Advantage
In today’s competitive software landscape, performance can be a key differentiator. An optimized Go application can outperform competitors and attract more users.
Now that you understand the importance of optimization let’s move on to the practical aspects of profiling and performance tuning.
2. Profiling Your Go Application
Profiling is the process of analyzing your application’s runtime behavior to identify performance bottlenecks. Go provides powerful built-in tools for profiling, making it relatively easy to spot areas that need improvement.
2.1. CPU Profiling
CPU profiling helps you identify functions or code blocks that consume the most CPU time. To enable CPU profiling in your Go application, import the net/http/pprof package and add the following code:
go import _ "net/http/pprof"
Then, expose the profiling endpoints in your HTTP server:
go go func() { log.Println(http.ListenAndServe("localhost:6060", nil)) }()
With the profiling server running, you can access CPU profiling information by visiting http://localhost:6060/debug/pprof/profile in your web browser. This will generate a CPU profile that you can analyze using tools like go tool pprof.
2.2. Memory Profiling
Memory profiling helps you identify memory leaks and excessive memory usage. To enable memory profiling, add the following code to your Go application:
go import _ "net/http/pprof"
Then, expose the profiling endpoints as shown earlier. You can access memory profiling information at http://localhost:6060/debug/pprof/heap and http://localhost:6060/debug/pprof/goroutine.
2.3. Goroutine Profiling
Goroutine profiling allows you to identify the number of goroutines in your application and their states. To enable goroutine profiling, import the net/http/pprof package and expose the profiling endpoints as described earlier. Access goroutine profiling information at http://localhost:6060/debug/pprof/goroutine.
2.4. Block Profiling
Block profiling helps you identify blocks of code where goroutines are frequently waiting. To enable block profiling, import the net/http/pprof package and expose the profiling endpoints as shown earlier. You can access block profiling information at http://localhost:6060/debug/pprof/block.
3. Analyzing Profiling Data
Once you’ve collected profiling data, it’s time to analyze it and identify performance bottlenecks. The go tool pprof command-line tool is your primary tool for this task. Here’s how you can use it:
bash go tool pprof http://localhost:6060/debug/pprof/profile
Replace the URL with the specific profiling endpoint you want to analyze (e.g., /debug/pprof/heap for memory profiling). This command will open an interactive shell where you can run various commands to inspect the data.
Some useful commands include:
- top: Displays the top functions consuming CPU time or memory.
- list <function>: Shows the source code for a specific function.
- web: Generates a graphical representation of function calls.
By using these commands and analyzing the generated reports, you can pinpoint performance bottlenecks in your Go application and take steps to optimize them.
4. Performance Tuning Strategies
Now that you’ve identified performance bottlenecks using profiling, it’s time to implement performance tuning strategies to improve your Go application’s speed and efficiency.
4.1. Algorithm Optimization
One of the most effective ways to improve performance is to optimize your algorithms. Look for opportunities to replace slow algorithms with more efficient ones. For example, consider using a hash map instead of linear search for data retrieval operations.
4.2. Data Structure Selection
Choosing the right data structures can significantly impact performance. Use data structures that are tailored to your specific use case. For instance, if you need to perform frequent insertions and deletions, consider using a linked list or a skip list instead of an array.
4.3. Concurrency
Go is known for its excellent support for concurrency with goroutines and channels. Leverage these features to parallelize tasks and take full advantage of multi-core processors. However, be mindful of synchronization overhead, and use channels and mutexes judiciously.
4.4. Profiling-Driven Optimization
Profiling should not be a one-time activity. Continuously monitor and profile your Go application in production to catch performance regressions early. Set up alerts based on critical performance metrics to notify you of issues as they arise.
4.5. Caching
Implement caching mechanisms to reduce the workload on critical components of your application. Caching can significantly improve response times and reduce the load on databases or external APIs.
4.6. Database Optimization
If your Go application interacts with a database, optimize your database queries. Indexing, query optimization, and connection pooling can all contribute to better database performance.
4.7. Code Review and Refactoring
Regularly review your codebase for performance bottlenecks. Refactor code that is difficult to maintain or contains redundant operations. Keep an eye out for memory leaks and fix them promptly.
5. Measuring the Impact
After implementing performance tuning strategies, it’s crucial to measure the impact of your optimizations. Use profiling tools to collect new data and compare it to the baseline data you collected earlier. This will help you quantify the improvements and ensure that your optimizations are effective.
Conclusion
Optimizing Go applications for performance is a continuous process that involves profiling, analyzing, and tuning. By following the profiling techniques and performance tuning strategies outlined in this blog, you can make your Go code faster, more efficient, and better equipped to handle increased workloads. Whether you’re building web services, microservices, or command-line tools, optimizing your Go applications will ultimately lead to a better user experience and a competitive edge in the world of software development. So, roll up your sleeves, dive into your code, and start optimizing for greatness.
Table of Contents
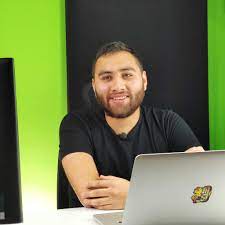
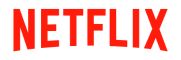