Optimizing Error Handling in Go: A Guide to Best Practices and Effective Patterns
Writing robust, maintainable, and efficient code requires meticulous error handling. In Go, an open-source statically typed compiled language, error handling is intentionally straightforward but doesn’t compromise on clarity and reliability. This level of coding expertise is what you get when you hire Golang developers who understand the language in-depth. This blog post delves into some best practices and patterns for error handling in Go, complete with practical examples. It provides insights into the kind of knowledge and skills that professional Golang developers bring to the table.
Understanding Error in Go
In Go, an `error` is an interface with a single method, `Error()`, that returns a string. This simple structure allows developers to build custom error types and include additional information. Here is the error interface:
```go type error interface { Error() string } ```
Always Check Errors
The first and most fundamental rule of error handling in Go is: always check errors. Unlike languages like Java or Python that use exceptions, Go uses return values to indicate errors. It’s tempting to ignore error return values, but doing so can lead to silent failures.
```go file, err := os.Open("nonexistent.txt") if err != nil { log.Fatal(err) } defer file.Close() ```
In the above code, `os.Open()` returns an error if the file doesn’t exist. By checking the error, we can exit the program rather than causing a nil pointer dereference when we attempt to close a non-existent file.
The `errors` Package
The `errors` package provides functions for creating new error types and inspecting errors. It’s a good practice to define your own error types for indicating specific conditions. The `errors.New()` function is used to create simple, static errors.
```go var ErrInvalidParameter = errors.New("invalid parameter") func process(n int) error { if n < 0 { return ErrInvalidParameter } // Processing logic here return nil } ```
In this example, we’ve defined a custom error that gets returned when a negative number is given as input to the `process()` function.
Wrapping Errors
Go 1.13 added `fmt.Errorf` and `errors.As`, `errors.Is`, and `errors.Unwrap` functions for wrapping and unwrapping errors, allowing you to add context to errors and preserve the original error type.
```go _, err := ioutil.ReadFile("nonexistent.txt") if err != nil { return fmt.Errorf("read failed: %w", err) } ```
The `%w` verb is a placeholder for an error. When this error is printed, it will have the additional “read failed” context. We can unwrap this error using `errors.Unwrap` or check if an error was a specific type using `errors.Is` or `errors.As`.
```go _, err := ioutil.ReadFile("nonexistent.txt") if err != nil { err = fmt.Errorf("read failed: %w", err) } if errors.Is(err, os.ErrNotExist) { fmt.Println("file does not exist") } ```
In this example, we’re checking if the error was specifically a file-not-exist error. Even though we wrapped the original error, we can still access it.
Use Error Variables for Comparisons
While using `errors.New()`, it’s recommended to declare errors as variables at the package level. This allows the calling code to compare the returned error to known values.
```go var ( ErrNotFound = errors.New("item not found") ErrTimeout = errors.New("operation timed out") ) func fetchData(id string) (Data, error) { // If the data is not found, return ErrNotFound // If fetching the data takes too long, return ErrTimeout } ``` The calling code can then do a comparison using the error variable: ``` go data, err := fetchData("123") if errors.Is(err, ErrNotFound) { // Handle not found case } else if errors.Is(err, ErrTimeout) { // Handle timeout case } ```
Using `pkg/errors`
The `pkg/errors` package extends Go’s error handling with functions like `Wrap` and `Cause`.
```go _, err := os.Open("nonexistent.txt") if err != nil { return errors.Wrap(err, "open failed") } ```
You can retrieve the root cause of the error using `errors.Cause()`.
```go cause := errors.Cause(err) if cause == os.ErrNotExist { // Handle the specific error } ```
Error Handling and Logging
Logging error details can be crucial for troubleshooting, especially in a language as explicit as Go. This is an important aspect of error handling that professional Golang developers understand well. It’s vital to log errors at the right place. As a rule of thumb, if the error is handled (not returned), it should be logged. If it’s returned, let the caller decide whether to log it or not. When you hire Golang developers, you can count on their expertise to manage these intricacies efficiently.
```go if err := doSomething(); err != nil { log.Printf("doSomething failed: %v", err) } ```
Conclusion
Go’s straightforward approach to error handling allows developers to write robust, clear code. This level of precision is what you gain when you hire Golang developers. They leverage patterns such as error wrapping, custom errors, and package-level error variables to ensure applications behave correctly in unexpected situations. Consistently checking and handling errors, along with thoughtful logging, can make troubleshooting easier and prevent silent failures. Understanding and applying these best practices is what distinguishes professional Golang developers and is essential for writing efficient Go code.
Table of Contents
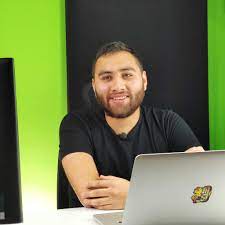
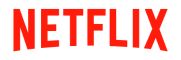