Optimizing Memory Usage in Go: Reducing Memory Footprint
Go (also known as Golang) is a popular programming language known for its simplicity, efficiency, and concurrency support. However, like any programming language, Go applications can suffer from memory usage issues if not properly optimized. Excessive memory consumption not only affects the performance of your application but can also lead to scalability problems.
In this blog post, we will delve into the world of memory optimization in Go. We will explore various techniques, best practices, and code samples to help you reduce your Go application’s memory footprint. By the end of this article, you’ll be equipped with the knowledge to build efficient and memory-conscious Go applications.
1. Understanding Memory Usage in Go
Before we dive into optimization techniques, let’s briefly understand how memory is managed in Go.
1.1. Go’s Garbage Collector (GC)
Go uses a garbage collector (GC) to automatically manage memory. While this simplifies memory management for developers, it can also lead to unpredictable memory consumption if not used wisely. The GC periodically scans the heap to identify and free up memory that is no longer in use. However, improper memory handling can increase the frequency and duration of GC pauses, affecting the application’s performance.
1.2. Memory Allocation
In Go, memory is allocated using the new keyword or composite literals. For instance, var x = new(int) allocates memory for an integer and returns a pointer to it. Understanding how and when memory is allocated is crucial for optimizing memory usage.
2. Techniques for Optimizing Memory Usage
Now that we have a basic understanding of memory management in Go, let’s explore various techniques to optimize memory usage.
2.1. Use Pointers Wisely
Go’s pointers allow you to work with data indirectly, which can be memory-efficient. However, excessive use of pointers can lead to complex memory management and hinder performance. Use pointers when necessary, but don’t overuse them.
go // Avoid unnecessary pointers var x int y := &x // Unnecessary pointer
2.2. Avoid Global Variables
Global variables persist throughout the application’s lifetime, consuming memory even when not needed. Minimize the use of global variables and prefer local variables with limited scope.
go // Avoid global variables var globalData []int func main() { localData := []int{1, 2, 3} // Work with localData }
2.3. Reuse Data Structures
Instead of creating new data structures frequently, consider reusing them. This reduces memory allocation overhead and can significantly improve performance.
go // Create and reuse slices func processSlice(data []int) { // Process data } func main() { data1 := []int{1, 2, 3} processSlice(data1) data2 := []int{4, 5, 6} processSlice(data2) }
2.4. Use Sync.Pool
The sync.Pool package allows you to reuse objects of a specific type. This can be particularly useful for reducing memory allocations in high-concurrency scenarios.
go import ( "sync" ) var myPool = sync.Pool{ New: func() interface{} { return make([]byte, 1024) }, } func main() { data := myPool.Get().([]byte) defer myPool.Put(data) // Use data }
2.5. Minimize String Manipulation
Strings in Go are immutable, and manipulating them can result in multiple string copies in memory. To reduce memory usage, use the strings package efficiently and consider using []byte when frequent string modifications are required.
go // Inefficient string manipulation result := "" for _, s := range strings { result += s } // Efficient string manipulation var builder strings.Builder for _, s := range strings { builder.WriteString(s) } result := builder.String()
2.6. Profile Your Code
Go provides built-in profiling tools like pprof that can help you identify memory bottlenecks in your code. Profiling allows you to pinpoint areas that require optimization.
go import ( _ "net/http/pprof" "net/http" ) func main() { go func() { http.ListenAndServe(":6060", nil) }() // Your application code // Profile your code using pprof // (access http://localhost:6060/debug/pprof/ in your browser) }
2.7. Use Benchmarking
Benchmarking your code helps measure the impact of optimizations and ensures that changes do not introduce performance regressions. Go provides a testing and benchmarking framework that is easy to use.
go import ( "testing" ) func BenchmarkYourFunction(b *testing.B) { for i := 0; i < b.N; i++ { // Your code to benchmark } }
3. Best Practices for Memory Optimization
In addition to specific techniques, here are some best practices to keep in mind when optimizing memory usage in Go:
3.1. Profile First, Optimize Later
Don’t prematurely optimize your code. Use profiling tools to identify bottlenecks before making optimizations. This ensures that you focus on areas that truly impact performance.
3.2. Keep Data Structures Simple
Simplify your data structures whenever possible. Complex data structures often lead to increased memory usage and slower performance.
3.3. Test in Realistic Conditions
Benchmark and profile your code under conditions that resemble real-world usage. This ensures that your optimizations are relevant to your application’s actual performance requirements.
3.4. Stay Informed
Keep up-to-date with Go’s memory management improvements and best practices. The Go community regularly shares insights and updates on memory optimization techniques.
4. Real-World Memory Optimization Example
Let’s put our memory optimization knowledge to the test with a real-world example. Consider a simple web server that handles HTTP requests and stores data in memory. We’ll optimize the memory usage of this server.
go package main import ( "fmt" "net/http" ) var data []string func handler(w http.ResponseWriter, r *http.Request) { // Simulate adding data to our storage newData := "This is some data" data = append(data, newData) fmt.Fprintln(w, "Data added successfully!") } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
In this example, every HTTP request adds data to the data slice, potentially leading to unbounded memory usage. Let’s optimize it using the techniques we’ve discussed.
go package main import ( "fmt" "net/http" "sync" ) var dataPool = sync.Pool{ New: func() interface{} { return make([]string, 0, 1000) }, } func handler(w http.ResponseWriter, r *http.Request) { // Get the data slice from the pool data := dataPool.Get().([]string) defer dataPool.Put(data) // Simulate adding data to our storage newData := "This is some data" data = append(data, newData) fmt.Fprintln(w, "Data added successfully!") } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) }
In this optimized version, we use a sync.Pool to reuse the data slice, reducing memory allocations and preventing unbounded memory growth.
Conclusion
Efficient memory usage is crucial for the performance and scalability of your Go applications. By understanding Go’s memory management, applying optimization techniques, and following best practices, you can significantly reduce your application’s memory footprint.
Remember that memory optimization should be guided by profiling and benchmarking. Don’t optimize blindly; measure the impact of your changes to ensure they have a positive effect on your application’s performance.
Optimizing memory usage is an ongoing process, and staying informed about the latest developments in Go’s memory management is essential. By following these guidelines, you can build Go applications that are not only efficient but also capable of handling large-scale workloads with ease.
Table of Contents
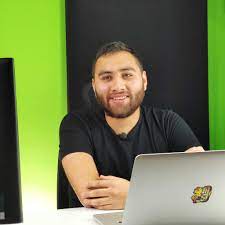
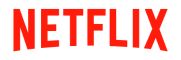