Go Q & A
What are some performance optimization techniques in Go?
Optimizing performance in Go applications involves identifying bottlenecks, reducing resource consumption, and improving execution speed. Here are some performance optimization techniques in Go:
- Profiling: Use Go’s built-in profiling tools (pprof) to identify CPU, memory, and blocking profile of your application. Profiling helps pinpoint areas of code that consume the most resources or experience performance bottlenecks.
- Goroutines and Concurrency: Leverage Goroutines and channels for concurrent execution of tasks. Design your application to take advantage of Go’s lightweight threading model, which enables efficient parallelism and concurrency.
- Memory Management: Be mindful of memory allocation and deallocation in your code. Use object pooling, reuse memory buffers, and minimize unnecessary allocations to reduce memory overhead and garbage collection pressure.
- Optimized Data Structures and Algorithms: Choose data structures and algorithms optimized for your application’s specific requirements. Use built-in data structures like slices, maps, and arrays efficiently and prefer algorithms with lower time and space complexity.
- Compiler Optimizations: Utilize Go compiler optimizations (-gcflags) to optimize code generation, inlining, and escape analysis. Experiment with different compiler flags to fine-tune performance characteristics of your application.
- Caching and Memoization: Cache frequently accessed data or expensive computations to avoid redundant work and improve overall application performance. Use caching libraries like bigcache or groupcache for efficient in-memory caching.
- Database and I/O Optimization: Optimize database queries, index selection, and network I/O operations to minimize latency and maximize throughput. Consider using connection pooling, batch processing, and asynchronous I/O for better performance.
- Benchmarking and Testing: Write benchmarks and unit tests to measure the performance impact of code changes and ensure that optimizations yield expected results. Benchmark critical code paths and identify opportunities for further optimization.
By applying these performance optimization techniques, you can enhance the efficiency, scalability, and responsiveness of your Go applications, delivering better user experience and reducing operational costs.
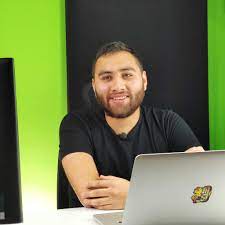
Previously at
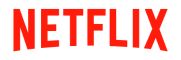
Over 5 years of experience in Golang. Led the design and implementation of a distributed system and platform for building conversational chatbots.