Go Q & A
How do you handle race conditions in Go?
Race conditions occur when multiple Goroutines access shared resources concurrently, leading to unpredictable behavior and data corruption. Go provides several mechanisms to handle race conditions and ensure safe concurrent access to shared resources:
- Mutexes and Locks: Use mutexes (sync.Mutex) and locks (sync.RWMutex) to synchronize access to shared resources and enforce mutual exclusion. Acquire locks before accessing shared data and release them after modification to prevent data races.
- Atomic Operations: Utilize atomic operations provided by the sync/atomic package to perform low-level synchronization of shared variables without explicit locking. Atomic operations ensure that operations on shared variables are executed atomically and avoid data races.
- Channel Communication: Use channels for communication and synchronization between Goroutines to avoid race conditions. Design your application to pass data between Goroutines using channels rather than shared memory to ensure safe concurrent access.
- Go Race Detector: Leverage Go’s built-in race detector (go run -race) to detect and identify data races during runtime. The race detector instruments your code and reports potential data races, enabling you to diagnose and fix race conditions early in the development cycle.
- Immutable Data Structures: Consider using immutable data structures and values to minimize shared mutable state and reduce the likelihood of race conditions. Immutable data structures are inherently thread-safe and eliminate the need for explicit synchronization.
- Concurrency Patterns: Familiarize yourself with common concurrency patterns such as worker pools, producer-consumer queues, and message passing to design concurrent applications that minimize contention and avoid race conditions.
- Testing and Code Review: Write comprehensive unit tests and conduct thorough code reviews to identify and address potential race conditions in your codebase. Test your application under various concurrency scenarios to ensure correctness and reliability.
By following these best practices and techniques, you can effectively handle race conditions in Go and build concurrent applications that are robust, reliable, and free from data races.
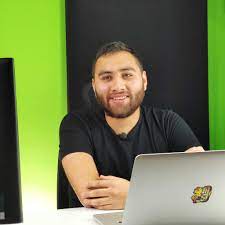
Previously at
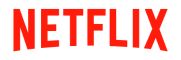
Over 5 years of experience in Golang. Led the design and implementation of a distributed system and platform for building conversational chatbots.