Introduction to Go’s Reflection API: Dynamic Programming in GoLang
When working with programming languages, the ability to inspect and manipulate data types and structures dynamically can be invaluable. It allows us to write more flexible and generic code, enabling us to handle unknown types and structures at runtime. This capability is known as reflection, and GoLang provides a powerful and concise Reflection API to achieve this.
In this blog post, we will delve into the world of Go’s Reflection API, exploring its features and understanding how it facilitates dynamic programming in GoLang. We’ll also explore various use cases where reflection can be a handy tool, but it’s essential to use it judiciously due to its potential performance overhead.
1. What is Reflection?
Reflection is the ability of a program to examine its own structure and properties at runtime. It allows us to analyze variables, functions, and data structures without knowing their types at compile-time. In essence, reflection empowers us to treat types as first-class citizens, enabling dynamic manipulation of objects based on their types.
GoLang’s Reflection API provides a set of packages and functions that allow us to achieve this dynamic behavior. The primary package for reflection is reflect, which offers methods to inspect and modify variables, function values, and data structures.
2. Getting Started with Reflection
Before we dive into specific examples, let’s understand the fundamental concepts of reflection in GoLang. The central concept in reflection is the reflect.Type and reflect.Value types. reflect.Type represents the type of a GoLang value, and reflect.Value represents the actual value of the object.
2.1. Getting the Type and Value of an Object
To obtain the type and value of an object using reflection, we use the reflect.TypeOf() and reflect.ValueOf() functions, respectively. Let’s see a simple example:
go package main import ( "fmt" "reflect" ) func main() { var num int = 42 typeOfNum := reflect.TypeOf(num) valueOfNum := reflect.ValueOf(num) fmt.Println("TypeOf:", typeOfNum) // Output: TypeOf: int fmt.Println("ValueOf:", valueOfNum) // Output: ValueOf: 42 }
In this example, we declared a variable num of type int and used reflect.TypeOf() to get its type, which is int, and reflect.ValueOf() to get its value, which is 42.
2.2. Kind and Type
The reflect.Type has a method called Kind() that returns the specific kind of type. Kind refers to the basic classification of a type, such as int, string, bool, struct, etc.
go package main import ( "fmt" "reflect" ) func main() { var name string = "Alice" typeOfName := reflect.TypeOf(name) fmt.Println("TypeOf:", typeOfName) // Output: TypeOf: string fmt.Println("Kind:", typeOfName.Kind()) // Output: Kind: string }
Here, we get the reflect.Type of a string variable name and then use the Kind() method to determine that the kind of type is a string.
2.3. Using Reflection to Modify Values
Reflection not only enables us to extract information about a variable but also allows us to modify its value if it’s addressable. Addressable values can be modified through reflection. Here’s an example:
go package main import ( "fmt" "reflect" ) func main() { var num int = 42 valueOfNum := reflect.ValueOf(&num) if valueOfNum.Kind() == reflect.Ptr { valueOfNum = valueOfNum.Elem() valueOfNum.SetInt(100) // Modify the value of num using reflection } fmt.Println("Modified value:", num) // Output: Modified value: 100 }
In this example, we have a variable num, and we obtain its reflect.Value using reflect.ValueOf(). Since we want to modify the value of num, we check if the valueOfNum is a pointer using Kind(). If it is, we use Elem() to get the actual value and then modify it using SetInt().
3. Dynamic Programming with Reflection
Now that we have a basic understanding of reflection and how to use reflect.Type and reflect.Value, let’s explore how we can utilize reflection for dynamic programming in GoLang.
3.1. Creating Generic Functions
GoLang is a statically typed language, and it does not support generics like other languages (e.g., Java, C#). However, with reflection, we can create generic-like functions that can work with various types.
Let’s create a generic function called PrintTypeAndValue that takes an interface{} and prints its type and value:
go package main import ( "fmt" "reflect" ) func PrintTypeAndValue(data interface{}) { dataType := reflect.TypeOf(data) dataValue := reflect.ValueOf(data) fmt.Println("Type:", dataType) fmt.Println("Value:", dataValue) } func main() { var num int = 42 PrintTypeAndValue(num) // Output: Type: int, Value: 42 var name string = "Alice" PrintTypeAndValue(name) // Output: Type: string, Value: Alice }
In this example, the PrintTypeAndValue function can accept any type of data using the empty interface interface{}. Inside the function, we utilize reflection to determine the type and value of the input data, allowing us to print it accordingly.
However, it’s important to note that using reflection for generic functions comes with a performance cost since reflection involves runtime type checks and lookups.
3.2. Unmarshaling JSON with Reflection
Unmarshaling JSON data into GoLang structs is a common task. By using reflection, we can create a more generic JSON unmarshaling function that works with different types of structs.
Consider the following example:
go package main import ( "encoding/json" "fmt" "reflect" ) type Person struct { Name string `json:"name"` Age int `json:"age"` } func UnmarshalJSON(jsonData []byte, target interface{}) error { value := reflect.ValueOf(target) if value.Kind() != reflect.Ptr || value.IsNil() { return fmt.Errorf("target must be a non-nil pointer") } return json.Unmarshal(jsonData, target) } func main() { jsonData := []byte(`{"name": "Alice", "age": 30}`) var person Person err := UnmarshalJSON(jsonData, &person) if err != nil { fmt.Println("Error:", err) } else { fmt.Println("Unmarshaled Person:", person) } }
In this example, we have a generic JSON unmarshaling function UnmarshalJSON that accepts any type of struct as the target. The function checks whether the target is a non-nil pointer before proceeding with the unmarshaling process.
3.3. Accessing Struct Fields with Reflection
Reflection allows us to access and modify struct fields dynamically, which can be useful when dealing with unknown or dynamic data structures. Let’s see an example of accessing and modifying struct fields using reflection:
go package main import ( "fmt" "reflect" ) type Person struct { Name string Age int } func main() { person := Person{ Name: "Bob", Age: 25, } // Accessing struct fields using reflection personValue := reflect.ValueOf(person) nameField := personValue.FieldByName("Name") ageField := personValue.FieldByName("Age") fmt.Println("Original Name:", nameField.String()) // Output: Original Name: Bob fmt.Println("Original Age:", ageField.Int()) // Output: Original Age: 25 // Modifying struct fields using reflection nameField.SetString("Alice") ageField.SetInt(30) fmt.Println("Modified Name:", person.Name) // Output: Modified Name: Alice fmt.Println("Modified Age:", person.Age) // Output: Modified Age: 30 }
In this example, we create a Person struct and use reflection to access its fields using reflect.ValueOf() and FieldByName(). We then modify the struct fields using reflection with SetString() and SetInt().
Conclusion
In this blog post, we explored the world of GoLang’s Reflection API, understanding its core concepts and how it enables dynamic programming. We learned how to retrieve the type and value of objects, modify values using reflection, and create generic-like functions that can handle different types. Additionally, we explored practical use cases of reflection, such as generic functions, JSON unmarshaling, and accessing struct fields dynamically.
However, it’s crucial to use reflection judiciously, as it can lead to reduced performance and can make the code harder to understand and maintain. In most cases, static typing and interfaces should be preferred over reflection for better code clarity and efficiency.
Reflection, when used thoughtfully, can significantly enhance the versatility and dynamism of your GoLang applications, making it a powerful tool in your programming arsenal. Happy coding!
Table of Contents
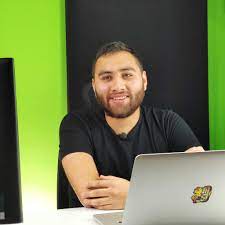
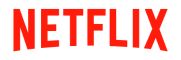