Building RESTful Microservices with Go and gRPC
In the world of modern software development, building applications that are scalable, maintainable, and efficient is a top priority. Microservices architecture has emerged as a popular approach to achieve these goals, allowing developers to break down large monolithic applications into smaller, independent services that can be developed, deployed, and scaled individually. Two popular communication protocols for microservices are RESTful APIs and gRPC, both of which offer distinct advantages. In this blog post, we’ll explore how to build RESTful microservices and leverage gRPC in Go, providing a comprehensive guide to creating robust and high-performing microservices.
1. Introduction to Microservices and Communication Protocols
Microservices architecture involves breaking down an application into small, specialized services that communicate with each other to fulfill specific functions. Efficient communication between these services is crucial for the overall success of the architecture. RESTful APIs and gRPC are two widely used communication protocols in the microservices world.
REST (Representational State Transfer) is an architectural style that uses HTTP methods to perform CRUD (Create, Read, Update, Delete) operations on resources. It relies on a stateless client-server interaction model and is ideal for exposing resources over HTTP.
gRPC, on the other hand, is a high-performance, open-source framework for remote procedure calls. It employs the HTTP/2 protocol and uses Protocol Buffers (protobufs) for serialization, making it highly efficient in terms of both speed and data size. gRPC supports multiple programming languages and provides features such as bi-directional streaming and automatic generation of client and server code.
2. Building RESTful Microservices with Go
2.1. Setting Up the Development Environment
Before we dive into building RESTful microservices, ensure you have Go installed. You can download it from the official website and set up your workspace accordingly.
2.2. Creating a Basic RESTful Service
Let’s start by creating a simple RESTful microservice using Go and the popular Gorilla Mux router.
go package main import ( "fmt" "log" "net/http" "github.com/gorilla/mux" ) func main() { r := mux.NewRouter() r.HandleFunc("/api/resource", getResource).Methods("GET") r.HandleFunc("/api/resource", createResource).Methods("POST") http.Handle("/", r) fmt.Println("Server started on :8080") log.Fatal(http.ListenAndServe(":8080", nil)) } func getResource(w http.ResponseWriter, r *http.Request) { // Implement logic to fetch resource } func createResource(w http.ResponseWriter, r *http.Request) { // Implement logic to create resource }
2.3. Implementing Endpoints and Handlers
In the code above, we’ve defined two endpoints, one for retrieving a resource and another for creating a resource. However, the handler functions getResource and createResource are placeholders. You can implement the actual logic to fetch and create resources within these functions.
go func getResource(w http.ResponseWriter, r *http.Request) { // Fetch the resource and send a JSON response } func createResource(w http.ResponseWriter, r *http.Request) { // Parse the request body, create the resource, and send a JSON response }
2.4. Data Validation and Error Handling
As part of building robust microservices, it’s essential to implement data validation and proper error handling. You can use third-party libraries like validator for data validation and return appropriate HTTP status codes and error messages for various scenarios.
go func createResource(w http.ResponseWriter, r *http.Request) { // Parse and validate the request body // If validation fails, return a 400 Bad Request response // Create the resource // If creation is successful, return a 201 Created response with the resource details // If creation fails, return a 500 Internal Server Error response }
2.5. Testing the RESTful Service
Testing is a critical part of microservices development. Write unit tests using the Go testing framework to ensure that your endpoints and handlers function as expected. Additionally, you can use tools like curl or Postman to manually test the endpoints.
3. Leveraging gRPC for Microservices Communication
3.1. Introduction to gRPC
gRPC is a powerful framework for microservices communication, providing features like bi-directional streaming, payload compression, and automatic client and server code generation. It uses Protocol Buffers (protobufs) for defining service methods and data structures.
3.2. Defining gRPC Services and Messages
Start by defining your gRPC service and messages using Protocol Buffers. Here’s an example of a simple service for managing user accounts:
protobuf syntax = "proto3"; package user; service UserService { rpc CreateUser(UserRequest) returns (UserResponse); rpc GetUser(GetUserRequest) returns (User); } message UserRequest { string username = 1; string email = 2; } message GetUserRequest { string user_id = 1; } message UserResponse { string user_id = 1; string message = 2; } message User { string user_id = 1; string username = 2; string email = 3; }
3.3. Implementing gRPC Server and Client in Go
Next, use the Protocol Buffers definition to generate Go code for your gRPC server and client. Implement the server-side logic and client-side code accordingly.
go package main import ( "context" "fmt" "log" "net" "google.golang.org/grpc" "userpb" // Import the generated protobuf code "github.com/google/uuid" ) type userServiceServer struct { } func (s *userServiceServer) CreateUser(ctx context.Context, req *userpb.UserRequest) (*userpb.UserResponse, error) { // Implement user creation logic } func (s *userServiceServer) GetUser(ctx context.Context, req *userpb.GetUserRequest) (*userpb.User, error) { // Implement logic to fetch user by ID } func main() { fmt.Println("Starting gRPC server...") lis, err := net.Listen("tcp", ":50051") if err != nil { log.Fatalf("Failed to listen: %v", err) } s := grpc.NewServer() userpb.RegisterUserServiceServer(s, &userServiceServer{}) if err := s.Serve(lis); err != nil { log.Fatalf("Failed to serve: %v", err) } }
On the client side, you can use the generated client code to interact with the gRPC service.
go package main import ( "context" "fmt" "log" "google.golang.org/grpc" "userpb" // Import the generated protobuf code ) func main() { fmt.Println("Starting gRPC client...") conn, err := grpc.Dial("localhost:50051", grpc.WithInsecure()) if err != nil { log.Fatalf("Could not connect: %v", err) } defer conn.Close() client := userpb.NewUserServiceClient(conn) // Use the client to make gRPC calls }
3.4. Error Handling and Interceptors in gRPC
gRPC provides a robust error handling mechanism, allowing you to define custom error codes and messages in your protobuf definitions. You can also use interceptors to add cross-cutting concerns such as logging, authentication, and rate limiting to your gRPC services.
3.5. Comparing RESTful APIs and gRPC
Both RESTful APIs and gRPC have their strengths and use cases. RESTful APIs are well-suited for simple communication over HTTP, while gRPC excels in scenarios where high performance and efficiency are essential, especially in microservices architectures.
4. Best Practices for Microservices Development
As you build microservices using Go and utilize both RESTful APIs and gRPC, consider these best practices:
4.1. Service Isolation and Independence
Design your microservices to be isolated and independent, each responsible for a specific domain or functionality. This allows for easier maintenance, scaling, and deployment.
4.2. Versioning and Compatibility
Implement versioning in your APIs to ensure backward and forward compatibility as your microservices evolve. Consider using URL versioning or content negotiation for RESTful APIs and protocol buffers for gRPC.
4.3. Monitoring and Logging
Implement thorough monitoring and logging to gain insights into the performance and health of your microservices. Use tools like Prometheus and Grafana for monitoring and log aggregation services for centralized logging.
4.4. Security Considerations
Implement strong security practices such as authentication and authorization mechanisms, especially when dealing with sensitive data or interactions between microservices.
Conclusion
Building microservices using Go and combining the strengths of RESTful APIs and gRPC can lead to a powerful and scalable architecture. RESTful APIs provide simplicity and familiarity, while gRPC offers efficiency and flexibility. By understanding these communication protocols and following best practices, you can create microservices that are robust, maintainable, and capable of meeting the demands of modern software development.
In this blog post, we’ve covered the basics of building RESTful microservices with Go, explored the features of gRPC, and discussed best practices for microservices development. Whether you’re a seasoned developer or just getting started, mastering these techniques will empower you to create highly efficient and resilient microservices architectures.
Table of Contents
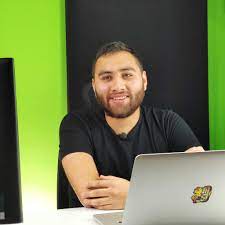
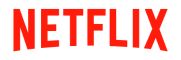