Building RESTful Web Services with Go and the Gin Framework
In the modern world of web development, building robust and efficient RESTful web services is crucial for creating scalable and maintainable applications. One of the standout tools in this domain is the Go programming language, known for its performance and simplicity. Complementing Go’s capabilities, the Gin web framework provides a lightweight yet powerful foundation for creating RESTful APIs. In this guide, we’ll explore the process of building RESTful web services using Go and the Gin framework, step by step.
1. What are RESTful Web Services?
REST, or Representational State Transfer, is an architectural style for designing networked applications. RESTful web services are APIs that adhere to the principles of REST, using HTTP methods for communication and utilizing URIs to represent resources. These services are stateless, scalable, and offer a standardized way for different systems to communicate and exchange data.
2. Introducing the Go Programming Language
Go, often referred to as Golang, is a statically typed, compiled language designed with simplicity and performance in mind. Its concise syntax, garbage collection, and excellent support for concurrency make it an ideal choice for building high-performance web services.
3. Getting Started with the Gin Framework
3.1. Installation
Before diving into Gin, make sure you have Go installed on your system. You can install Gin using the following command:
bash go get -u github.com/gin-gonic/gin
3.2. Creating a Basic Server
To create a basic server using Gin, import the necessary packages and define a simple route. Here’s an example:
go package main import ( "github.com/gin-gonic/gin" ) func main() { r := gin.Default() r.GET("/", func(c *gin.Context) { c.JSON(200, gin.H{ "message": "Hello, Gin!", }) }) r.Run(":8080") }
4. Designing RESTful Endpoints
4.1. Routing
Gin provides a powerful routing mechanism that allows you to define RESTful endpoints easily. You can use the GET, POST, PUT, PATCH, and DELETE methods to map routes to specific functions.
go r.GET("/users", getUsers) r.POST("/users", createUser) r.GET("/users/:id", getUserByID) r.PUT("/users/:id", updateUser) r.DELETE("/users/:id", deleteUser)
4.2. Handling Requests and Responses
Gin’s context (c) provides various methods to handle requests and responses. You can parse incoming JSON data, access query parameters, and send JSON responses effortlessly.
go func createUser(c *gin.Context) { var user User if err := c.ShouldBindJSON(&user); err != nil { c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()}) return } // Create the user and send a response }
5. Structuring Data and Models
5.1. Defining Data Structures
Define your data structures using Go’s struct types. These structures represent the shape of your data and can be used for both input validation and output serialization.
go type User struct { ID int `json:"id"` Username string `json:"username"` Email string `json:"email"` }
5.2. Working with JSON
Gin’s context provides methods to easily marshal and unmarshal JSON data. This makes it simple to convert data between JSON and Go structs.
go func getUserByID(c *gin.Context) { id := c.Param("id") // Fetch user by ID and send as JSON response }
6. Implementing CRUD Operations
6.1. Creating Records
To create a record, bind the incoming JSON data to a struct and then perform the necessary database operations.
go func createUser(c *gin.Context) { var user User if err := c.ShouldBindJSON(&user); err != nil { c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()}) return } // Create the user and send a response }
6.2. Reading Records
Retrieve records based on various criteria, such as IDs or query parameters, and return them as JSON responses.
go func getUsers(c *gin.Context) { // Fetch users from the database and send as JSON response }
6.3. Updating Records
Update records by parsing incoming JSON data, finding the record by ID, and applying the changes.
go func updateUser(c *gin.Context) { id := c.Param("id") var updatedUser User if err := c.ShouldBindJSON(&updatedUser); err != nil { c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()}) return } // Update the user and send a response }
6.4. Deleting Records
Delete records by their ID or other unique identifiers.
go func deleteUser(c *gin.Context) { id := c.Param("id") // Delete the user and send a response }
7. Middleware for Enhanced Functionality
7.1. Logging
Gin supports middleware, allowing you to add cross-cutting concerns like logging to your application.
go func LoggerMiddleware() gin.HandlerFunc { return func(c *gin.Context) { // Log request details c.Next() // Log response details } } // Attach the middleware r.Use(LoggerMiddleware())
7.2. Authentication and Authorization
Implement middleware to handle authentication and authorization for your routes.
go func AuthMiddleware() gin.HandlerFunc { return func(c *gin.Context) { // Check authentication and authorization if authorized { c.Next() } else { c.AbortWithStatus(http.StatusUnauthorized) } } } // Attach the middleware to specific routes r.GET("/protected", AuthMiddleware(), protectedRouteHandler)
8. Error Handling and Validation
8.1. Handling Errors
Gin provides a way to handle errors and return appropriate responses to clients.
go func getUserByID(c *gin.Context) { id := c.Param("id") user, err := getUserFromDatabase(id) if err != nil { c.JSON(http.StatusNotFound, gin.H{"error": "User not found"}) return } c.JSON(http.StatusOK, user) }
8.2. Input Validation
Gin has built-in validation features that allow you to validate incoming data easily.
go type CreateUserInput struct { Username string `json:"username" binding:"required"` Email string `json:"email" binding:"required,email"` } func createUser(c *gin.Context) { var input CreateUserInput if err := c.ShouldBindJSON(&input); err != nil { c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()}) return } // Create the user and send a response }
9. Testing Your RESTful Web Services
9.1. Unit Testing
Write unit tests to ensure the correctness of your individual functions and handlers.
go func TestCreateUser(t *testing.T) { // Set up mock environment // Call createUser function // Check the response }
9.2. Integration Testing
Perform integration tests to ensure that your API works as expected when all components are combined.
go func TestAPI(t *testing.T) { // Set up test server // Make requests to the API // Check responses and behaviors }
10. Best Practices for Building RESTful Services with Gin
- Keep your routes and handlers organized and modular.
- Use middleware for cross-cutting concerns to keep your codebase clean.
- Validate incoming data and handle errors gracefully.
- Keep your data structures well-defined and follow consistent naming conventions.
- Thoroughly test your API endpoints to ensure correctness and reliability.
- Implement proper authentication and authorization mechanisms to secure your API.
Conclusion
In this guide, we’ve explored the process of building RESTful web services using Go and the Gin framework. We’ve covered the basics of creating a server, designing RESTful endpoints, handling requests and responses, working with data models, implementing CRUD operations, incorporating middleware, handling errors, and testing your services. Armed with this knowledge, you can confidently create efficient and powerful RESTful APIs using the Go programming language and the Gin framework. Happy coding!
Table of Contents
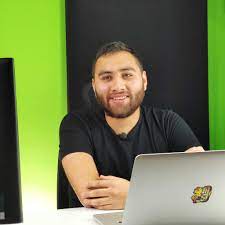
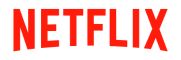