Crafting Seamless Web Experiences with Go’s net/http
When it comes to building web applications in the Go programming language, one of the most critical packages in your toolkit is `net/http`. This package provides the building blocks for handling HTTP requests and responses, making it an essential component for developing web services and applications in Go.
Table of Contents
In this detailed guide, we will embark on a journey to understand how to effectively utilize Go’s `net/http` package for routing and middleware. By the end of this post, you’ll have a solid grasp of these concepts and be well-equipped to create robust web applications in Go.
1. Routing with `net/http`
Routing is the process of mapping incoming HTTP requests to specific handler functions in your application. It enables you to define how different URLs are handled within your web application. Go’s `net/http` package simplifies routing, making it easy to create clean and organized routes for your web application.
2. Setting Up a Basic Router
Let’s start with a basic example of setting up a router in Go using the `net/http` package:
```go package main import ( "net/http" ) func main() { http.HandleFunc("/", homeHandler) http.HandleFunc("/about", aboutHandler) http.ListenAndServe(":8080", nil) } func homeHandler(w http.ResponseWriter, r *http.Request) { // Handle the home route w.Write([]byte("Welcome to the home page")) } func aboutHandler(w http.ResponseWriter, r *http.Request) { // Handle the about route w.Write([]byte("Learn more about us on the about page")) } ```
In this example, we have defined two route handlers for the root (“/”) and “/about” paths. When an HTTP request arrives at your Go server for these paths, the respective handler function is invoked to generate and send the response.
3. URL Parameters and Path Variables
Routing becomes more powerful when you can work with dynamic URLs and extract data from them. Go’s `net/http` package supports URL parameters, allowing you to capture data from the URL path.
```go func productHandler(w http.ResponseWriter, r *http.Request) { vars := mux.Vars(r) productID := vars["id"] // Use productID to retrieve and display product details } ```
In this example, we use the Gorilla Mux router, a popular third-party package for routing in Go, to capture a product ID from the URL path.
4. Middleware in Go
Middleware is a crucial concept in web development that enables you to execute code before or after the main handler function. It’s a powerful tool for implementing cross-cutting concerns such as authentication, logging, and request/response modification.
5. Implementing Middleware
Let’s dive into a more detailed example of implementing middleware in Go:
```go func main() { // Create a new router router := mux.NewRouter() // Attach middleware router.Use(loggingMiddleware) // Define routes and handlers router.HandleFunc("/", homeHandler) router.HandleFunc("/about", aboutHandler) http.Handle("/", router) http.ListenAndServe(":8080", nil) } func loggingMiddleware(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { // Perform logging operations before handling the request log.Println("Request received:", r.URL.Path) next.ServeHTTP(w, r) // Perform logging operations after handling the request log.Println("Request completed.") }) } ```
In this example, we use the Gorilla Mux router again and create a custom middleware function `loggingMiddleware`. This middleware logs information about each incoming request before and after it’s handled by the main handler.
6. Chaining Middleware
One of the strengths of middleware is its ability to be chained together. This allows you to apply multiple middleware functions to a route or group of routes in a specific order.
```go func main() { // Create a new router router := mux.NewRouter() // Attach multiple middleware functions router.Use(loggingMiddleware) router.Use(authMiddleware) // Define routes and handlers router.HandleFunc("/", homeHandler) router.HandleFunc("/about", aboutHandler) http.Handle("/", router) http.ListenAndServe(":8080", nil) } ```
In this example, both `loggingMiddleware` and `authMiddleware` are applied to all routes defined within the router.
7. External Resources for Further Learning
To deepen your understanding of Go’s `net/http` package, routing, and middleware, here are some external resources to explore:
- Official `net/http` documentation – https://pkg.go.dev/net/http: The official documentation provides in-depth information on the `net/http` package and its usage.
- Gorilla Mux – https://github.com/gorilla/mux: If you need advanced routing capabilities, the Gorilla Mux router is a popular choice in the Go community.
- Creating Middleware in Go – https://drstearns.github.io/tutorials/gomiddleware/: This tutorial offers a comprehensive look at creating middleware in Go and explores various use cases.
Conclusion
Go’s `net/http` package, along with the flexibility of middleware, empowers you to build robust web applications. With the knowledge gained from this guide and further exploration of the provided resources, you are well on your way to becoming a proficient Go web developer. Happy coding!
Table of Contents
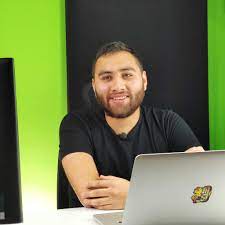
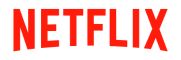