Diving into Go’s Standard Library: Exploring Powerful Built-in Packages
Go is a modern, statically-typed programming language known for its simplicity, performance, and excellent concurrency support. One of the key strengths of Go is its comprehensive standard library, which provides a rich set of packages and functionalities for developers to leverage. In this blog post, we will dive deep into Go’s standard library and explore some of its powerful built-in packages. By understanding the capabilities of these packages and learning how to use them effectively, you can enhance your productivity and write more efficient Go programs.
Introduction to Go’s Standard Library:
The standard library is a collection of packages that come bundled with every Go installation. It offers a wide range of functionalities, including string manipulation, file I/O, network operations, encoding/decoding, and more. These packages are designed to be efficient, reliable, and optimized for performance.
By relying on the standard library, developers can avoid reinventing the wheel and focus on writing business logic rather than low-level infrastructure code. Additionally, since the packages are part of the standard library, they undergo rigorous testing and are well-maintained by the Go community. Let’s take a look at some of the more common packages of the standard library:
1. Package: fmt
Printing and Formatting Output:
The fmt package is one of the most commonly used packages in the Go standard library. It provides functions for formatted I/O operations, including printing output to the console and formatting strings.
go package main import "fmt" func main() { fmt.Println("Hello, World!") fmt.Printf("Pi is approximately %.2f\n", 3.14159) }
In the above code snippet, the Println function is used to print the “Hello, World!” message to the console. The Printf function demonstrates string formatting, where the placeholder %.2f specifies that the floating-point number should be displayed with two decimal places.
2. Package: strings
Manipulating Strings:
The strings package provides a wide range of functions for manipulating strings in Go. It includes operations like concatenation, trimming, replacing, splitting, and case conversions.
go package main import ( "fmt" "strings" ) func main() { str := "Welcome to Go" fmt.Println(strings.ToUpper(str)) fmt.Println(strings.Replace(str, "Go", "Golang", 1)) fmt.Println(strings.Split(str, " ")) }
In the above example, we use the ToUpper function to convert the string to uppercase, Replace to replace the word “Go” with “Golang” (with a limit of 1 replacement), and Split to split the string into individual words based on spaces.
3. Package: io
Input/Output Operations:
The io package provides essential interfaces and functions for performing input/output operations in Go. It enables reading from and writing to various data sources, such as files, network connections, and in-memory buffers.
go package main import ( "fmt" "io/ioutil" "os" ) func main() { file, err := os.Open("input.txt") if err != nil { fmt.Println("Error opening file:", err) return } defer file.Close() data, err := ioutil.ReadAll(file) if err != nil { fmt.Println("Error reading file:", err) return } fmt.Println("File contents:", string(data)) }
In the above code snippet, we open a file called “input.txt” using os.Open and read its contents using ioutil.ReadAll. The file is then closed using the defer statement to ensure it is properly closed after reading.
4. Package: net/http
Building Web Applications:
The net/http package is a powerful package for building web applications and working with HTTP in Go. It provides functions and types for handling HTTP requests and responses, routing, serving static files, and more.
go package main import ( "fmt" "net/http" ) func helloHandler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, World!") } func main() { http.HandleFunc("/", helloHandler) http.ListenAndServe(":8080", nil) }
In the above example, we define an HTTP handler function helloHandler that writes the “Hello, World!” message to the response writer. The http.HandleFunc function sets up the routing, and http.ListenAndServe starts the HTTP server on port 8080.
5. Package: time
Time and Date Operations:
The time package provides functionalities for working with time and date in Go. It offers features like parsing and formatting dates, calculating durations, and manipulating time zones.
go package main import ( "fmt" "time" ) func main() { now := time.Now() fmt.Println("Current time:", now.Format("2006-01-02 15:04:05")) future := now.Add(time.Hour * 24) fmt.Println("Tomorrow:", future.Format("2006-01-02 15:04:05")) duration := future.Sub(now) fmt.Println("Duration:", duration.Hours(), "hours") }
In the above code snippet, we use time.Now to get the current time and Format to format it as a string. We also demonstrate adding a day to the current time using Add, calculating the duration between two times using Sub, and retrieving the duration in hours.
Conclusion:
In this blog post, we have explored just a few of the many powerful packages available in Go’s standard library. By leveraging these built-in packages, you can save time, write more efficient code, and enhance the functionality of your Go programs.
The Go standard library is a treasure trove of utilities and functionalities waiting to be discovered. Take the time to explore the documentation and experiment with different packages to unlock the full potential of Go for your development projects.
Table of Contents
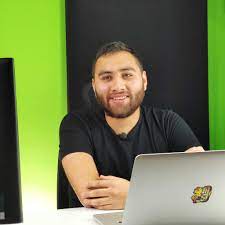
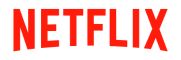