Does Go have support for middleware?
Yes, Go has support for middleware through the use of the net/http package and third-party middleware libraries. Middleware functions in Go are used to intercept incoming HTTP requests, perform pre-processing or post-processing tasks, and delegate to the next handler in the request processing chain.
Middleware functions can add cross-cutting concerns such as logging, authentication, authorization, rate limiting, request/response transformations, and error handling to HTTP handlers and endpoints in Go web applications.
The net/http package provides a simple and flexible mechanism for defining middleware functions using the http.Handler interface. Middleware functions in Go are implemented as ordinary Go functions that accept an http.Handler as input and return a new http.Handler that wraps the original handler with additional functionality.
Here’s a basic example demonstrating how to implement middleware in Go using the net/http package:
go package main import ( "log" "net/http" "time" ) func loggerMiddleware(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { start := time.Now() log.Printf("Request: %s %s", r.Method, r.URL.Path) next.ServeHTTP(w, r) log.Printf("Response time: %v", time.Since(start)) }) } func helloHandler(w http.ResponseWriter, r *http.Request) { w.Write([]byte("Hello, World!")) } func main() { mux := http.NewServeMux() mux.HandleFunc("/hello", helloHandler) // Use loggerMiddleware for all requests http.ListenAndServe(":8080", loggerMiddleware(mux)) }
In this example, we define a loggerMiddleware function that logs information about incoming HTTP requests, including the HTTP method and URL path, as well as the response time. The loggerMiddleware function wraps the original HTTP handler and delegates the request processing to it using the ServeHTTP method of the http.Handler interface. +96
Middleware functions can be composed and chained together to form a request processing pipeline, where each middleware function performs a specific task or adds a specific behavior to the request processing flow. Middleware functions can be applied globally to all HTTP handlers and endpoints or selectively to specific routes or groups of routes.
In addition to the built-in middleware support provided by the net/http package, there are also several third-party middleware libraries available for Go that offer additional functionality and features, such as authentication, authorization, session management, CORS (Cross-Origin Resource Sharing) support, and more.
By using middleware in Go web applications, developers can modularize and encapsulate common HTTP request processing logic, promote code reuse and maintainability, and implement cross-cutting concerns in a flexible and scalable manner.
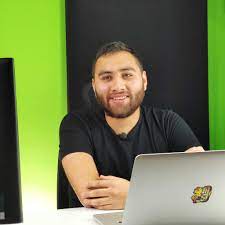
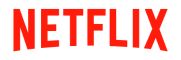