Exploring Go’s Template Engine: Creating Dynamic HTML Templates
When building web applications, one of the essential tasks is generating dynamic HTML content to present data to users. Go, also known as Golang, is a popular programming language renowned for its simplicity, performance, and concurrency capabilities. It comes with a powerful built-in template engine that allows developers to create dynamic HTML templates with ease. In this blog, we’ll delve into Go’s template engine and explore how to use it effectively to render dynamic HTML templates.
1. Understanding the Go Template Engine
The Go template engine, part of the standard library, is inspired by Django’s template system and provides a robust way to generate dynamic content. It separates the presentation layer from the logic and ensures clean, maintainable code. The templates are plain text files containing placeholders for dynamic data that will be replaced during rendering.
The placeholders, also known as template actions, are enclosed within double curly braces {{ }}. When executed, these actions generate the final content by evaluating expressions and invoking functions.
2. Getting Started with Templates
To use Go’s template engine, start by importing the html/template package. You can either use the built-in template.New() function to create a new template or load templates from files using template.ParseFiles().
go package main import ( "html/template" "os" ) func main() { // Create a new template tmpl := template.New("myTemplate") // Parse the template content templateContent := "Hello, {{.}}!" tmpl, err := tmpl.Parse(templateContent) if err != nil { panic(err) } // Provide data to the template data := "Gopher" err = tmpl.Execute(os.Stdout, data) if err != nil { panic(err) } }
In this example, we create a new template, parse a simple template string, and provide data (“Gopher”) to replace the placeholder ({{.}}) before rendering it to the standard output.
3. Basic Template Actions
Go’s template engine provides various actions to manipulate data and control the rendering process. Let’s explore some of the basic template actions:
3.1. Printing Values
To print the value of a variable, use {{ . }}. This action replaces the placeholder with the actual value during rendering.
go package main import ( "html/template" "os" ) func main() { tmpl := template.New("printTemplate") templateContent := "Hello, {{.}}!" tmpl, err := tmpl.Parse(templateContent) if err != nil { panic(err) } data := "World" err = tmpl.Execute(os.Stdout, data) if err != nil { panic(err) } }
Output:
Hello, World!
3.2. Conditional Statements
You can use {{ if }}, {{ else }}, and {{ end }} to create conditional blocks in your template. The content within the {{ if }} block will be rendered if the condition is true; otherwise, the content within the {{ else }} block (if provided) will be rendered.
go package main import ( "html/template" "os" ) func main() { tmpl := template.New("conditionalTemplate") templateContent := ` {{ if . }} <p>{{ . }}</p> {{ else }} <p>No data available.</p> {{ end }} ` tmpl, err := tmpl.Parse(templateContent) if err != nil { panic(err) } data := "Some content" err = tmpl.Execute(os.Stdout, data) if err != nil { panic(err) } }
Output:
css <p>Some content</p>
If data is an empty string, the output will be:
css <p>No data available.</p>
3.3. Iterating Over Lists
You can use {{ range }} and {{ end }} to iterate over lists in your template. The content within the {{ range }} block will be rendered for each item in the list.
go package main import ( "html/template" "os" ) func main() { tmpl := template.New("loopTemplate") templateContent := ` <ul> {{ range . }} <li>{{ . }}</li> {{ end }} </ul> ` tmpl, err := tmpl.Parse(templateContent) if err != nil { panic(err) } data := []string{"Apple", "Banana", "Orange"} err = tmpl.Execute(os.Stdout, data) if err != nil { panic(err) } }
Output:
css <ul> <li>Apple</li> <li>Banana</li> <li>Orange</li> </ul>
4. Advanced Template Actions
Go’s template engine also provides powerful features like function calls, custom functions, and template inclusion.
4.1. Function Calls
You can call functions from within the templates using the {{ call }} action. This allows you to perform more complex operations and calculations on your data.
go package main import ( "html/template" "os" "strings" ) func toUppercase(s string) string { return strings.ToUpper(s) } func main() { tmpl := template.New("functionCallTemplate") templateContent := ` {{ $name := "gopher" }} {{ call .ToUpper $name }} ` tmpl, err := tmpl.Funcs(template.FuncMap{ "ToUpper": toUppercase, }).Parse(templateContent) if err != nil { panic(err) } err = tmpl.Execute(os.Stdout, nil) if err != nil { panic(err) } }
Output:
GOPHER
In this example, we define a custom toUppercase function and call it using {{ call }} within the template.
4.2. Custom Functions
You can define and use custom functions within your templates. Custom functions allow you to encapsulate complex logic and reuse it across different templates.
go package main import ( "html/template" "os" "strings" ) func toUppercase(s string) string { return strings.ToUpper(s) } func main() { tmpl := template.New("customFunctionTemplate") templateContent := ` {{ $name := "gopher" }} {{ toUpper $name }} ` tmpl, err := tmpl.Funcs(template.FuncMap{ "toUpper": toUppercase, }).Parse(templateContent) if err != nil { panic(err) } err = tmpl.Execute(os.Stdout, nil) if err != nil { panic(err) } }
Output:
GOPHER
In this example, we define the toUppercase function as a custom function (toUpper) and use it directly in the template.
4.3. Template Inclusion
Go’s template engine allows you to include other templates within a template using the {{ template }} action. This enables better organization and reusability of your templates.
go package main import ( "html/template" "os" ) func main() { tmpl := template.New("parentTemplate") templateContent := ` <!DOCTYPE html> <html> <head> <title>{{ .Title }}</title> </head> <body> {{ template "contentTemplate" . }} </body> </html> ` tmpl, err := tmpl.Parse(templateContent) if err != nil { panic(err) } contentTemplateContent := ` <h1>Hello, Gopher!</h1> <p>Welcome to Go Template Engine.</p> ` tmpl, err = tmpl.New("contentTemplate").Parse(contentTemplateContent) if err != nil { panic(err) } data := map[string]string{"Title": "My Page"} err = tmpl.Execute(os.Stdout, data) if err != nil { panic(err) } }
Output:
php <!DOCTYPE html> <html> <head> <title>My Page</title> </head> <body> <h1>Hello, Gopher!</h1> <p>Welcome to Go Template Engine.</p> </body> </html>
In this example, we define a parent template that includes a child template called “contentTemplate” using {{ template }}. The child template is provided with dynamic data using the data map.
Conclusion
Go’s template engine provides an elegant and powerful solution for creating dynamic HTML templates. Its simplicity, combined with the ability to execute custom functions and conditionally render content, makes it a versatile tool for generating dynamic web content. By mastering the Go template engine, developers can build scalable and maintainable web applications that present data in a dynamic and engaging manner.
In this blog, we’ve explored the fundamentals of Go’s template engine, demonstrated basic and advanced template actions, and touched on the use of custom functions and template inclusion. Armed with this knowledge, you’re now ready to dive deeper into Go’s template capabilities and build amazing web applications with dynamic HTML templates. Happy coding!
Table of Contents
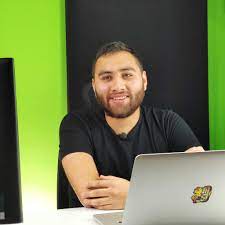
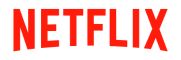