Exploring Go’s Testing Framework: Writing Effective Unit Tests
In the world of software development, testing plays a crucial role in ensuring the reliability, stability, and correctness of our code. Go, a statically typed language developed at Google, comes with a built-in testing framework that empowers developers to write effective unit tests. In this blog post, we’ll dive into the fundamentals of Go’s testing framework and uncover strategies for writing unit tests that stand the test of time.
1. Why Unit Testing Matters
Unit testing involves testing individual units or components of a software application in isolation. These units could be functions, methods, or even entire packages. The goal of unit testing is to validate that each unit of code performs as expected and produces the desired output for a given set of inputs. Unit tests are an essential part of the development process as they:
- Catch Bugs Early: By identifying issues at an early stage, unit tests help prevent the propagation of bugs to other parts of the codebase.
- Facilitate Refactoring: Unit tests provide a safety net that allows developers to confidently make changes to the codebase, knowing that if something breaks, the tests will catch it.
- Improve Code Quality: Writing tests encourages writing modular, well-organized, and maintainable code.
- Document Behavior: Unit tests serve as documentation by showcasing the intended behavior of the code.
2. Go’s Built-in Testing Framework
Go’s testing framework is comprehensive and straightforward, making it easy for developers to create and run tests. The framework relies on the standard library’s testing package, which provides the necessary tools for writing tests, running them, and reporting the results.
2.1. Writing Your First Test
To get started, create a new file ending with _test.go in the same directory as the code you want to test. For instance, if you have a file named calculator.go, your test file should be named calculator_test.go. In this file, you can define test functions using the Test prefix, which will be automatically discovered and executed by the testing framework.
Here’s a basic example of a test function for a simple Add function:
go // calculator.go package calculator func Add(a, b int) int { return a + b } go // calculator_test.go package calculator_test import ( "testing" "yourmodulepath/calculator" ) func TestAdd(t *testing.T) { result := calculator.Add(2, 3) if result != 5 { t.Errorf("Expected 5, but got %d", result) } }
In the test function, we’re using the t.Errorf method to report failures. If the test fails, this method will print the error message along with the stack trace, making it easy to diagnose the issue.
2.2. Running Tests
Running tests in Go is as simple as using the go test command. Open your terminal and navigate to the directory containing your test files and code files. Then, run the command:
sh go test
The testing framework will automatically discover and execute the test functions in the _test.go files. You’ll see output indicating whether the tests have passed or failed.
3. Writing Effective Unit Tests
While writing unit tests is a straightforward process, writing effective unit tests requires careful consideration and adherence to best practices.
3.1. Test Coverage
Test coverage refers to the percentage of your code that is covered by tests. Aim for high test coverage to ensure that most, if not all, of your code is validated through tests. Go provides tools to measure test coverage, such as the go test command with the -cover flag.
sh go test -cover
3.2. Table-Driven Tests
Table-driven tests involve creating a table of input-output pairs to validate different scenarios. This approach makes it easier to add new test cases and ensures that the same test logic is applied to multiple inputs.
go func TestAdd(t *testing.T) { testCases := []struct { a, b, expected int }{ {2, 3, 5}, {-1, 1, 0}, {0, 0, 0}, } for _, tc := range testCases { result := calculator.Add(tc.a, tc.b) if result != tc.expected { t.Errorf("For %d + %d, expected %d but got %d", tc.a, tc.b, tc.expected, result) } } }
3.3. Mocking Dependencies
When testing functions that rely on external dependencies, such as databases or APIs, it’s essential to mock those dependencies to isolate the unit under test. Tools like the gomock library can help you create mock implementations for your dependencies.
3.4. Benchmarking
Go’s testing framework also provides support for benchmarking code using the Benchmark functions. Benchmarking helps you assess the performance of your code and identify bottlenecks.
go func BenchmarkAdd(b *testing.B) { for i := 0; i < b.N; i++ { calculator.Add(2, 3) } }
3.5. Using t.Run for Subtests
Go’s testing framework allows you to create subtests using the t.Run method. Subtests can help you logically group related tests and provide more granular feedback in case of failures.
go func TestCalculator(t *testing.T) { t.Run("Addition", func(t *testing.T) { // Your addition test cases here }) t.Run("Subtraction", func(t *testing.T) { // Your subtraction test cases here }) }
Conclusion
Writing effective unit tests in Go is not just about ensuring code correctness; it’s about building a reliable and maintainable codebase. Go’s built-in testing framework simplifies the process, and by following best practices such as maintaining high test coverage, using table-driven tests, and mocking dependencies, you can create robust tests that stand the test of time. Embrace unit testing as a fundamental part of your development workflow, and you’ll be well on your way to delivering high-quality software. Happy testing!
Table of Contents
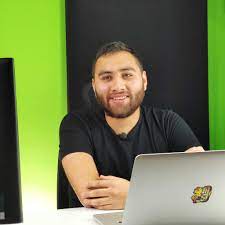
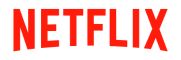