Can you perform unit testing in Go?
Yes, Go has robust support for unit testing built into the standard library through the testing package. Unit testing is an essential practice in software development that involves testing individual units or components of a program in isolation to ensure they function correctly.
The testing package in Go provides a simple and effective framework for writing and executing unit tests for Go code. It includes functions and utilities for defining test cases, running tests, and reporting test results.
Unit testing in Go follows a convention where test files are suffixed with _test.go to distinguish them from regular source files. Each test file contains one or more test functions, which are functions with names starting with Test followed by the name of the function or method being tested.
Here’s an example of a simple unit test in Go:
go package mypackage import ( "testing" ) func Add(a, b int) int { return a + b } func TestAdd(t *testing.T) { result := Add(2, 3) expected := 5 if result != expected { t.Errorf("Add(2, 3) returned %d, expected %d", result, expected) } }
In this example, we define a function Add that adds two integers and a corresponding test function TestAdd that verifies the correctness of the Add function. We use the testing.T type to report test failures and errors using methods like Errorf and Fatal.
To run the tests, we use the go test command, which automatically discovers and executes test functions in all files ending with _test.go in the current directory and its subdirectories. The go test command compiles the test code, executes the tests, and reports the results, including the number of tests executed, the number of failures, and any error messages.
Unit testing is an integral part of the Go development workflow and is widely used by Go developers to ensure code correctness, reliability, and maintainability. By writing comprehensive unit tests, developers can identify and fix bugs early in the development process, improve code quality, and build more robust and resilient software systems.
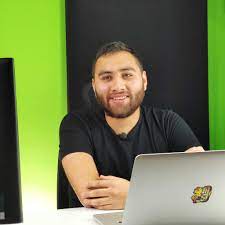
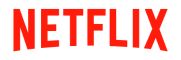