Go for Web Development: Choosing the Right Framework
In the rapidly evolving landscape of web development, choosing the right framework can make all the difference. Go, also known as Golang, has gained significant traction in recent years due to its speed, efficiency, and simplicity. If you’re considering using Go for your web development projects, you’re in for a treat. In this blog post, we’ll delve into the world of Go web development frameworks, explore popular options, and guide you through the process of selecting the framework that aligns best with your project’s needs.
1. Why Go for Web Development?
Go is a statically typed, compiled programming language designed to be efficient and productive. Its simple and clean syntax, along with its robust standard library, makes it an attractive choice for web development. Here are a few reasons why Go is a great option for building web applications:
- Performance: Go’s compiled nature and lightweight concurrency model make it highly performant, ensuring your web applications can handle a large number of users and requests without compromising speed.
- Concurrency: Go’s goroutines and channels enable seamless concurrency, allowing developers to write efficient and scalable code that can handle multiple tasks concurrently.
- Scalability: Go’s architecture is well-suited for building scalable applications. It’s an excellent choice for microservices architectures and distributed systems.
- Simplicity: Go’s simple and intuitive syntax reduces the learning curve for new developers, making it easier to onboard team members and maintain codebases.
2. Popular Go Web Development Frameworks
When it comes to choosing a framework for your Go web development project, several options are available, each catering to different needs. Let’s explore some of the most popular Go web frameworks:
2.1. Gin: Lightweight and High-Performance
Gin is a minimalistic web framework that focuses on speed and performance. It’s designed to be fast and efficient, making it an excellent choice for building APIs and microservices. Here’s a simple example of how you can create a basic web server using Gin:
go package main import ( "github.com/gin-gonic/gin" ) func main() { r := gin.Default() r.GET("/", func(c *gin.Context) { c.JSON(200, gin.H{ "message": "Hello, Gin!", }) }) r.Run(":8080") }
2.2. Echo: Fast and Uncomplicated
Echo is another lightweight framework that prides itself on being easy to use and high-performing. It provides a simple and clean API for building web applications. Here’s a snippet demonstrating how to set up a basic route in Echo:
go package main import ( "net/http" "github.com/labstack/echo/v4" ) func main() { e := echo.New() e.GET("/", func(c echo.Context) error { return c.String(http.StatusOK, "Hello, Echo!") }) e.Start(":8080") }
2.3. Fiber: Expressive and Flexible
Fiber is known for its expressiveness and flexibility, offering a wide range of features for building web applications. It’s designed to be efficient and has a similar API to Express.js. Here’s a simple Fiber example:
go package main import ( "github.com/gofiber/fiber/v2" ) func main() { app := fiber.New() app.Get("/", func(c *fiber.Ctx) error { return c.SendString("Hello, Fiber!") }) app.Listen(":8080") }
3. Factors to Consider When Choosing a Framework
Choosing the right framework involves careful consideration of your project’s requirements, team expertise, and long-term goals. Here are some factors to keep in mind:
3.1. Project Scope and Complexity
Evaluate the complexity of your project. For simple APIs or microservices, a lightweight framework like Gin or Echo might be sufficient. If you’re building a more extensive application with multiple components, a more feature-rich framework like Fiber could be a better fit.
3.2. Performance and Scalability
Consider the expected traffic and load on your application. Frameworks like Gin are optimized for performance and are ideal for high-concurrency scenarios. If your project demands efficient resource utilization and scalability, prioritize frameworks that excel in these areas.
3.3. Learning Curve
Assess the familiarity of your team with the chosen framework. While Go’s syntax is straightforward, different frameworks may have varying levels of complexity. Opt for a framework that aligns with your team’s skill set to ensure a smoother development process.
3.4. Community and Support
A strong community can provide valuable resources, plugins, and solutions to common problems. Check the framework’s documentation, GitHub repository, and community forums to gauge the level of support and engagement.
3.5. Ecosystem and Integrations
Consider the compatibility of the framework with external tools, libraries, and services you plan to use. A framework with a robust ecosystem and integrations can streamline your development process and enhance your application’s functionality.
Conclusion
Go has emerged as a powerful language for web development, offering a variety of frameworks to suit different project requirements. Whether you’re aiming for high performance, simplicity, or flexibility, there’s a Go framework that fits the bill. By considering factors such as project scope, performance needs, team expertise, and community support, you can confidently choose the right framework for your web development endeavors. Embrace Go’s efficiency and build web applications that shine in both functionality and speed. Happy coding!
In this blog post, we’ve explored the world of Go web development frameworks, delving into popular options like Gin, Echo, and Fiber. We’ve also discussed essential factors to consider when making your framework choice. Armed with this knowledge, you’re now well-equipped to embark on your Go web development journey and create efficient, high-performing applications that meet your project’s unique demands.
Table of Contents
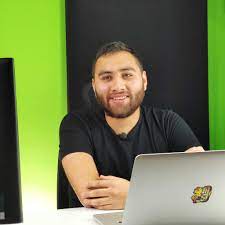
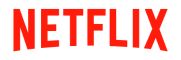