The Future of Monitoring: Go and WebSocket for Real-Time Dashboards
In today’s fast-paced tech landscape, real-time data is crucial for making informed decisions and staying ahead of the competition. Whether you’re a startup founder, a VC investor, or a tech leader, the ability to create real-time dashboards can be a game-changer for monitoring your business or investments. In this article, we’ll dive into the world of real-time dashboards and how to build them using the Go programming language and WebSocket technology.
Table of Contents
1. Why Real-Time Dashboards Matter
Before we delve into the technical details, let’s understand why real-time dashboards are essential for early-stage startup founders, VC investors, and tech leaders. Real-time dashboards provide:
- Immediate Insights: With real-time data, you can react quickly to market changes, customer behavior, or system performance issues.
- Competitive Advantage: By staying updated in real time, you can outperform competitors who rely on static reports or delayed data.
- Enhanced Decision-Making: Real-time dashboards empower you to make data-driven decisions promptly.
Now, let’s get down to business and explore how to build real-time dashboards using Go and WebSocket.
2. Getting Started with Go and WebSocket
Go, also known as Golang, is a robust and efficient programming language that’s gaining popularity in the tech industry. WebSocket, on the other hand, is a communication protocol that enables bidirectional, real-time communication between clients and servers. Combining Go and WebSocket, you can create dynamic, responsive dashboards.
2.1. Setting Up Your Environment
Before you start coding, ensure you have Go installed on your machine. You can download it from the official website: [https://golang.org/]
2.2. Installing WebSocket Libraries
Go has excellent WebSocket libraries that make it easy to implement real-time functionality. One of the most popular libraries is `gorilla/websocket`. You can install it using the following command:
``` go get github.com/gorilla/websocket ```
2.3. Building a Simple WebSocket Server
Now, let’s create a basic WebSocket server in Go. Here’s a simplified example:
```go package main import ( "net/http" "github.com/gorilla/websocket" ) var upgrader = websocket.Upgrader{ ReadBufferSize: 1024, WriteBufferSize: 1024, } func handler(w http.ResponseWriter, r *http.Request) { conn, err := upgrader.Upgrade(w, r, nil) if err != nil { return } defer conn.Close() for { // Read and write WebSocket messages here } } func main() { http.HandleFunc("/ws", handler) http.ListenAndServe(":8080", nil) } ```
This code sets up a WebSocket server that listens on port 8080 and handles incoming WebSocket connections. You can adapt this code to your specific use case.
2.4. Creating a Real-Time Dashboard
Now, let’s build a simple real-time dashboard using HTML and JavaScript. Your HTML file might look like this:
```html <!DOCTYPE html> <html> <head> <title>Real-Time Dashboard</title> </head> <body> <div id="data"></div> <script> const socket = new WebSocket("ws://localhost:8080/ws"); socket.onmessage = (event) => { const data = JSON.parse(event.data); document.getElementById("data").innerText = `Received data: ${data.message}`; }; </script> </body> </html> ```
This HTML file creates a WebSocket connection to your Go server and displays incoming data in real time.
2.5. Integrating Data Sources
To make your dashboard truly valuable, you’ll need to integrate it with relevant data sources. Whether it’s financial data, user analytics, or server performance metrics, ensure that your dashboard pulls data in real time and presents it in a meaningful way.
Conclusion
In this article, we’ve explored the importance of real-time dashboards for founders, investors, and tech leaders. We’ve also started building a real-time dashboard using Go and WebSocket, giving you the foundation to create your own dynamic monitoring tool.
Remember that the success of your dashboard relies on the quality of the data you collect and how you present it. With the power of Go and WebSocket, you can stay ahead of the competition and make data-driven decisions that drive your business or investments to new heights.
For further reading and resources on Go and WebSocket, you can check out the following links:
- Official Go Website – https://golang.org/
- gorilla/websocket Documentation – https://pkg.go.dev/github.com/gorilla/websocket
Table of Contents
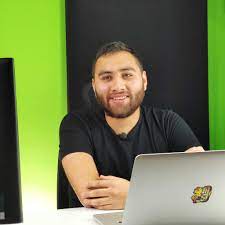
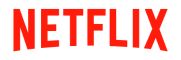