Working with JSON in Go: Parsing, Encoding, and Manipulating Data
JSON (JavaScript Object Notation) has become the de facto standard for data interchange on the web. Its simplicity and human-readable format make it popular among developers. When working with JSON data in Go, you have powerful tools at your disposal. In this blog post, we will explore how to parse, encode, and manipulate JSON data using the Go programming language. Whether you’re building web applications, APIs, or working with external services, understanding how to handle JSON effectively in Go is crucial.
1. Understanding JSON in Go:
JSON is a lightweight data interchange format that uses human-readable text to transmit and store structured data. In Go, the standard library provides excellent support for working with JSON. The encoding/json package offers functions and types that simplify parsing and encoding JSON data.
2. Parsing JSON in Go:
2.1 Unmarshaling JSON:
To parse JSON data into Go structs or maps, you can use the Unmarshal function provided by the encoding/json package. It automatically maps JSON keys to struct fields or map keys. You can define the struct fields with appropriate data types to match the JSON structure.
Code Sample:
go type Person struct { Name string `json:"name"` Age int `json:"age"` } func main() { data := []byte(`{"name":"John Doe","age":30}`) var person Person if err := json.Unmarshal(data, &person); err != nil { log.Fatal(err) } fmt.Println(person) }
2.2 Parsing Nested JSON:
JSON data often contains nested objects or arrays. Go allows you to parse nested JSON structures by defining nested structs or using the map[string]interface{} type for dynamic parsing. You can access nested fields using dot notation or type assertions.
Code Sample:
go type Book struct { Title string `json:"title"` Author struct { Name string `json:"name"` Country string `json:"country"` } `json:"author"` } func main() { data := []byte(`{"title":"The Go Programming Language","author":{"name":"Alan A. A. Donovan","country":"United States"}}`) var book Book if err := json.Unmarshal(data, &book); err != nil { log.Fatal(err) } fmt.Println(book.Title) fmt.Println(book.Author.Name) }
2.3 Handling JSON Arrays:
When dealing with JSON arrays, you can unmarshal them into Go slices or arrays. The JSON data will be automatically mapped to the appropriate Go data types. You can then access individual elements using array indexing.
Code Sample:
go type City struct { Name string `json:"name"` Population int `json:"population"` } func main() { data := []byte(`[{"name":"New York City","population":8336817},{"name":"Los Angeles","population":3979576}]`) var cities []City if err := json.Unmarshal(data, &cities); err != nil { log.Fatal(err) } for _, city := range cities { fmt.Println(city.Name) fmt.Println(city.Population) } }
3. Encoding JSON in Go:
3.1 Marshaling Structs to JSON:
To convert Go structs or maps into JSON data, you can use the Marshal function provided by the encoding/json package. It automatically converts struct fields or map keys into JSON keys.
Code Sample:
go type Person struct { Name string `json:"name"` Age int `json:"age"` } func main() { person := Person{Name: "Jane Smith", Age: 25} data, err := json.Marshal(person) if err != nil { log.Fatal(err) } fmt.Println(string(data)) }
3.2 Encoding Custom Data Types:
Go provides flexibility when encoding custom data types to JSON. You can implement the json.Marshaler interface to define custom encoding logic for your types. This allows you to control how your data is represented in JSON.
Code Sample:
go type CustomTime time.Time func (t CustomTime) MarshalJSON() ([]byte, error) { formatted := time.Time(t).Format("2006-01-02") return []byte(`"` + formatted + `"`), nil } type Event struct { Name string `json:"name"` Date CustomTime `json:"date"` } func main() { event := Event{Name: "Birthday Party", Date: CustomTime(time.Now())} data, err := json.Marshal(event) if err != nil { log.Fatal(err) } fmt.Println(string(data)) }
3.3 Pretty Printing JSON:
By default, the Marshal function produces compact JSON without any indentation or line breaks. If you want to generate human-readable JSON output, you can use the MarshalIndent function, which adds indentation and line breaks.
Code Sample:
go type Person struct { Name string `json:"name"` Age int `json:"age"` } func main() { person := Person{Name: "John Doe", Age: 30} data, err := json.MarshalIndent(person, "", " ") if err != nil { log.Fatal(err) } fmt.Println(string(data)) }
4. Manipulating JSON Data:
4.1 Modifying Existing JSON:
To modify an existing JSON object, you can unmarshal it into a struct or map, make the desired changes, and then marshal it back to JSON. This approach allows you to manipulate specific fields or nested objects easily.
4.2 Creating JSON from Scratch:
If you need to create JSON from scratch, you can define the data structure using Go types, populate the fields, and then marshal it to JSON. This is useful when constructing JSON payloads for APIs or generating configuration files.
4.3 Filtering JSON Data:
Go provides various techniques for filtering JSON data, such as using struct tags to select specific fields during marshaling or unmarshaling. You can also use JSONPath libraries or iterate over the parsed JSON to filter data based on specific criteria.
Conclusion:
In this blog post, we explored the basics of working with JSON in Go. We learned how to parse JSON data into Go structs, encode Go data into JSON, and manipulate JSON objects effectively. With the powerful features offered by the encoding/json package, handling JSON in Go becomes straightforward and efficient. JSON is a fundamental part of modern web development, and mastering its usage in Go will make you a more proficient developer.
Table of Contents
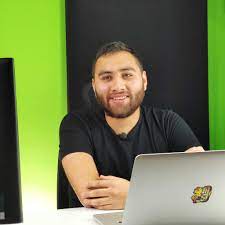
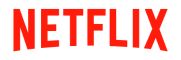