Working with MongoDB in Go: Database Operations and Querying
MongoDB is a popular NoSQL database that provides flexibility and scalability for storing and retrieving data. Go, also known as Golang, is a powerful programming language that’s gaining popularity for its performance and simplicity. When you combine the capabilities of MongoDB with the efficiency of Go, you get a robust solution for building scalable and high-performance applications.
In this blog post, we’ll explore how to work with MongoDB in Go. We’ll cover essential topics such as connecting to MongoDB, performing basic database operations, and executing queries. By the end of this guide, you’ll have a solid understanding of how to harness the power of MongoDB in your Go applications.
1. Prerequisites
Before diving into MongoDB with Go, make sure you have the following prerequisites in place:
- Go installed on your machine: You can download and install Go from the official website.
- MongoDB installed and running: You can follow the installation instructions for your operating system on the MongoDB website.
- MongoDB Go driver: To interact with MongoDB in Go, you’ll need to install the official MongoDB Go driver. You can install it using the following command:
go go get go.mongodb.org/mongo-driver/mongo
Now that we have our prerequisites sorted, let’s dive into the exciting world of MongoDB and Go.
2. Connecting to MongoDB
The first step in working with MongoDB in Go is establishing a connection to your MongoDB server. To do this, you’ll need to create a MongoDB client using the MongoDB Go driver. Here’s a code sample that demonstrates how to connect to a MongoDB instance:
go package main import ( "context" "fmt" "go.mongodb.org/mongo-driver/mongo" "go.mongodb.org/mongo-driver/mongo/options" ) func main() { // Set up a context with a timeout ctx, cancel := context.WithTimeout(context.Background(), 10*time.Second) defer cancel() // Specify the MongoDB connection string connectionString := "mongodb://localhost:27017" // Create a MongoDB client client, err := mongo.Connect(ctx, options.Client().ApplyURI(connectionString)) if err != nil { fmt.Println("Error connecting to MongoDB:", err) return } // Check if the connection was successful err = client.Ping(ctx, nil) if err != nil { fmt.Println("Error pinging MongoDB:", err) return } fmt.Println("Connected to MongoDB!") // Close the MongoDB client when done defer client.Disconnect(ctx) }
In this code snippet:
- We import the necessary packages, including the MongoDB Go driver.
- We set up a context with a timeout. It’s essential to manage context properly to avoid resource leaks.
- We specify the MongoDB connection string, which includes the hostname and port where your MongoDB server is running.
- We create a MongoDB client using the mongo.Connect method and provide the connection options.
- We check if the connection was successful by pinging the MongoDB server.
- Finally, we defer the client’s disconnection to ensure it’s closed when we’re done with it.
Now that we’ve established a connection to MongoDB, let’s move on to performing some basic database operations.
3. Performing Basic Database Operations
3.1. Inserting Data
One of the most common database operations is inserting data. Let’s see how we can insert documents into a MongoDB collection using Go. In this example, we’ll insert a document into a collection called “users.”
go // Define a struct for the user document type User struct { Name string `bson:"name"` Email string `bson:"email"` } func main() { // ... (previous code for connecting to MongoDB) // Get a handle to the "users" collection userCollection := client.Database("mydb").Collection("users") // Create a new user document newUser := User{ Name: "John Doe", Email: "johndoe@example.com", } // Insert the user document into the collection _, err = userCollection.InsertOne(ctx, newUser) if err != nil { fmt.Println("Error inserting document:", err) return } fmt.Println("Inserted a new user document!") }
In this code snippet:
- We define a User struct that represents the structure of our user document. The bson tags help map Go struct fields to MongoDB document fields.
- We get a handle to the “users” collection using the client.Database(“mydb”).Collection(“users”) syntax, where “mydb” is the name of the MongoDB database.
- We create a new User struct with the data we want to insert.
- We use the InsertOne method to insert the user document into the collection.
3.2. Querying Data
Now that we’ve inserted data, let’s explore how to query it. MongoDB offers powerful querying capabilities, and the MongoDB Go driver makes it easy to execute queries. Here’s an example of how to query all users from the “users” collection:
go func main() { // ... (previous code for connecting to MongoDB) // Get a handle to the "users" collection userCollection := client.Database("mydb").Collection("users") // Define a filter to match all documents (empty filter) filter := bson.D{} // Find all users in the collection cursor, err := userCollection.Find(ctx, filter) if err != nil { fmt.Println("Error finding documents:", err) return } defer cursor.Close(ctx) // Iterate through the cursor and decode documents var users []User for cursor.Next(ctx) { var user User if err := cursor.Decode(&user); err != nil { fmt.Println("Error decoding document:", err) return } users = append(users, user) } // Handle the queried users fmt.Println("Queried users:", users) }
In this code snippet:
- We get a handle to the “users” collection as before.
- We define a filter using a bson.D{} object, which matches all documents (an empty filter).
- We use the Find method to execute the query and obtain a cursor to the results.
- We iterate through the cursor, decode each document into a User struct, and collect the users in a slice.
- Finally, we handle the queried users as needed.
3.3. Updating and Deleting Data
MongoDB also provides methods for updating and deleting documents. Here’s how you can update a document in the “users” collection:
go func main() { // ... (previous code for connecting to MongoDB) // Get a handle to the "users" collection userCollection := client.Database("mydb").Collection("users") // Define a filter to match the document you want to update filter := bson.D{{"email", "johndoe@example.com"}} // Define the update operation update := bson.D{ {"$set", bson.D{ {"name", "Updated Name"}, }}, } // Perform the update result, err := userCollection.UpdateOne(ctx, filter, update) if err != nil { fmt.Println("Error updating document:", err) return } fmt.Printf("Updated %v document(s)\n", result.ModifiedCount) }
In this code snippet:
- We define a filter that matches the document we want to update based on the email field.
- We specify the update operation using the $set operator to update the “name” field.
- We use the UpdateOne method to perform the update and get the result, which includes information about how many documents were modified.
- Similarly, you can delete documents using the DeleteOne or DeleteMany methods, depending on your needs.
4. Querying with Filters and Sorting
MongoDB allows you to query data with various filtering criteria and sort the results. Let’s explore how to perform more complex queries in Go.
4.1. Filtering Data
Suppose you want to query users with a specific condition, such as finding all users with a name that starts with “J.” You can use the $regex operator to create a regular expression filter:
go func main() { // ... (previous code for connecting to MongoDB) // Get a handle to the "users" collection userCollection := client.Database("mydb").Collection("users") // Define a filter with a regular expression filter := bson.D{{"name", bson.D{ {"$regex", "^J"}, }}} // Find users matching the filter cursor, err := userCollection.Find(ctx, filter) if err != nil { fmt.Println("Error finding documents:", err) return } defer cursor.Close(ctx) // Iterate through the cursor and decode documents var users []User for cursor.Next(ctx) { var user User if err := cursor.Decode(&user); err != nil { fmt.Println("Error decoding document:", err) return } users = append(users, user) } fmt.Println("Users with names starting with 'J':", users) }
In this code snippet:
- We define a filter that uses the $regex operator to match user documents with a name starting with “J.”
- We execute the query as usual and retrieve the matching users.
4.2. Sorting Data
You can also sort the query results in ascending or descending order based on a specific field. Here’s how to sort users by their names in ascending order:
go func main() { // ... (previous code for connecting to MongoDB) // Get a handle to the "users" collection userCollection := client.Database("mydb").Collection("users") // Define a filter to match all documents (empty filter) filter := bson.D{} // Define the sorting criteria opts := options.Find().SetSort(bson.D{{"name", 1}}) // 1 for ascending, -1 for descending // Find all users and sort by name in ascending order cursor, err := userCollection.Find(ctx, filter, opts) if err != nil { fmt.Println("Error finding documents:", err) return } defer cursor.Close(ctx) // Iterate through the cursor and decode documents var users []User for cursor.Next(ctx) { var user User if err := cursor.Decode(&user); err != nil { fmt.Println("Error decoding document:", err) return } users = append(users, user) } fmt.Println("Users sorted by name (ascending):", users) }
In this code snippet:
- We define the sorting criteria using the options.Find().SetSort method. We sort by the “name” field in ascending order (1) by default.
- We pass the sorting options to the Find method to retrieve the sorted results.
- By combining filtering and sorting, you can tailor your queries to retrieve the exact data you need from MongoDB in your Go applications.
Conclusion
Working with MongoDB in Go opens up a world of possibilities for building efficient and scalable applications. In this blog post, we’ve covered the essential aspects of using MongoDB with Go, including connecting to the database, performing basic database operations, and executing queries with filtering and sorting.
As you continue your journey with MongoDB and Go, remember to explore the official MongoDB Go driver documentation for more advanced features and best practices. Additionally, consider diving deeper into Go and MongoDB to harness their full potential in your projects.
Now that you’ve learned the fundamentals, it’s time to start building your own Go applications powered by MongoDB. Happy coding!
Table of Contents
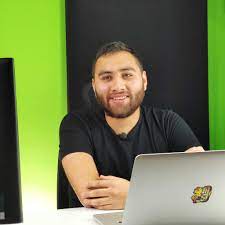
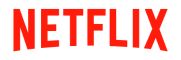