Implementing Game Center in iOS: Adding Social Gaming Features
Mobile gaming has evolved significantly over the years, transforming from simple single-player experiences to social, multiplayer adventures that connect players from around the world. To create a captivating gaming experience, it’s essential to incorporate social gaming features into your iOS games. Apple’s Game Center provides a powerful set of tools for achieving just that.
Table of Contents
In this comprehensive guide, we will walk you through the process of implementing Game Center in your iOS game. You’ll learn how to set up Game Center, add leaderboards, integrate achievements, and even enable real-time multiplayer gaming. So, let’s dive into the world of social gaming and enhance your iOS game’s engagement.
1. What is Game Center?
1.1 Understanding the Basics
Game Center is Apple’s social gaming platform, designed to enhance the multiplayer and social aspects of iOS games. It provides a wide range of features for developers to create engaging and competitive experiences. With Game Center, players can challenge friends, compare scores on leaderboards, and earn achievements, all while enjoying your game.
1.2 Benefits of Using Game Center
Why should you consider integrating Game Center into your iOS game? Here are some compelling reasons:
- Enhanced Engagement: Social gaming features like leaderboards and achievements can keep players hooked on your game as they compete with friends and other players.
- Increased Retention: Multiplayer gaming fosters a sense of community and encourages players to return to your game regularly.
- Monetization Opportunities: You can implement in-app purchases or ads strategically within your game to capitalize on its popularity.
- App Store Promotion: Games with Game Center features may get featured by Apple, resulting in increased visibility and downloads.
Now that you understand the benefits, let’s move on to the implementation.
2. Setting Up Game Center in Your Project
2.1 Creating an App ID
Before you can start using Game Center, you need to create an App ID for your game on the Apple Developer portal. Follow these steps:
- Log in to your Apple Developer account.
- Navigate to the “Certificates, IDs & Profiles” section.
- Under “Identifiers,” click on “App IDs” and then the “+” button to create a new App ID.
- Fill in the required information, including the Bundle ID of your game.
- Enable the “Game Center” capability for your App ID.
- Save the App ID.
2.2 Enabling Game Center in Xcode
With your App ID configured, it’s time to enable Game Center in your Xcode project:
- Open your Xcode project.
- Select the target for your game.
- Under the “Signing & Capabilities” tab, click the “+” button to add a new capability.
- Search for “Game Center” and enable it.
- Xcode will automatically create an entitlements file and update your project settings.
Now, Game Center is integrated into your project, and you’re ready to start implementing its features.
3. Implementing Leaderboards
3.1 Creating Leaderboards on App Store Connect
Leaderboards allow players to compete for the top spots on various scoreboards. To add leaderboards to your game, you need to set them up on App Store Connect:
- Log in to your App Store Connect account.
- Go to “My Apps” and select your game.
- Under the “Features” section, click on “Game Center.”
- Create leaderboards for your game, specifying their names and other details.
- Save your changes.
3.2 Integrating Leaderboards in Your Game
Now that you have created leaderboards, it’s time to integrate them into your iOS game. Here’s a simplified example of how you can submit a player’s score to a leaderboard using Swift:
swift import GameKit // Submit a player's score to a leaderboard func submitScoreToLeaderboard(score: Int, leaderboardID: String) { let scoreReporter = GKScore(leaderboardIdentifier: leaderboardID) scoreReporter.value = Int64(score) GKScore.report([scoreReporter]) { error in if let error = error { print("Error submitting score: \(error.localizedDescription)") } else { print("Score submitted successfully!") } } }
In this code snippet, we use the GameKit framework to create a GKScore object and submit a player’s score to the specified leaderboard. Don’t forget to handle errors gracefully and provide feedback to the player.
4. Adding Achievements
4.1 Setting Up Achievements on App Store Connect
Achievements are an excellent way to challenge and reward players in your game. To add achievements, follow these steps on App Store Connect:
- Log in to your App Store Connect account.
- Go to “My Apps” and select your game.
- Under the “Features” section, click on “Game Center.”
- Create achievements for your game, specifying their names, descriptions, and point values.
- Define the criteria required to unlock each achievement.
- Save your changes.
4.2 Incorporating Achievements into Your Game
Now, let’s implement achievements in your iOS game using Swift:
swift import GameKit // Unlock an achievement func unlockAchievement(achievementID: String) { let achievement = GKAchievement(identifier: achievementID) achievement.percentComplete = 100.0 GKAchievement.report([achievement]) { error in if let error = error { print("Error unlocking achievement: \(error.localizedDescription)") } else { print("Achievement unlocked successfully!") } } }
In this code snippet, we use the GameKit framework to unlock an achievement for the player. Remember to customize this code to match your game’s specific achievements and criteria.
5. Enabling Real-time Multiplayer Gaming
5.1 Setting Up Multiplayer Capabilities
If your game supports real-time multiplayer, you’ll need to configure multiplayer capabilities. Here’s how:
- In Xcode, open your project settings.
- Select the target for your game.
- Under the “Signing & Capabilities” tab, click the “+” button to add a new capability.
- Search for “GameKit” and enable it.
- Configure the multiplayer settings as needed, such as enabling voice chat or custom matchmaking.
- Implement the necessary logic to manage multiplayer sessions in your game.
5.2 Implementing Real-time Multiplayer Gameplay
Implementing real-time multiplayer gameplay is a complex topic that goes beyond the scope of this guide. However, GameKit provides tools and APIs for managing multiplayer sessions, handling player invitations, and synchronizing game state between devices. Refer to Apple’s official documentation and sample code for a deeper dive into this exciting feature.
6. Testing and Debugging
6.1 Testing Game Center Functionality
To ensure your Game Center integration works flawlessly, conduct thorough testing. Use the iOS Simulator to simulate different game scenarios, submit scores, and unlock achievements. Additionally, test multiplayer features on physical devices to catch any issues that might not surface in the simulator.
6.2 Common Debugging Tips
When debugging Game Center issues, consider the following:
- Check for network connectivity issues.
- Verify that the player is signed in to Game Center.
- Ensure that leaderboards and achievements are correctly configured on App Store Connect.
- Monitor error codes and messages for insights into what went wrong.
7. Best Practices and Tips
7.1 Designing Engaging Leaderboards
Create multiple leaderboards to cater to different skill levels or game modes.
Implement periodic leaderboard resets or seasons to keep the competition fresh.
Use meaningful leaderboard names and descriptions to entice players to participate.
7.2 Crafting Meaningful Achievements
Make achievements challenging but achievable to keep players engaged.
Offer a mix of easy, medium, and hard achievements to appeal to various player skill levels.
Provide hints or progress tracking to guide players toward unlocking achievements.
7.3 Ensuring Fair and Fun Multiplayer Gameplay
Implement fair matchmaking algorithms to avoid one-sided matches.
Encourage positive player interactions and penalize disruptive behavior.
Continuously monitor and update your game’s multiplayer features based on player feedback.
Conclusion
Integrating Game Center into your iOS game can significantly enhance its social and competitive aspects, making it more appealing to players and increasing its longevity. By following the steps outlined in this guide, you’ll be well on your way to creating a captivating gaming experience that keeps players coming back for more.
Remember that Game Center is a dynamic platform, so stay updated with the latest features and best practices to continually improve your game and provide players with an exciting and engaging social gaming experience.
Table of Contents
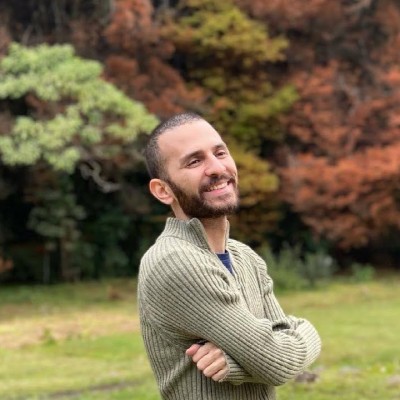
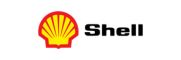