Advanced Animation Techniques in iOS: Bringing Your Apps to Life
In the world of app development, creating a visually appealing and engaging user experience is paramount. While sleek designs and intuitive interfaces are essential, adding animation takes your iOS app to a whole new level. Advanced animation techniques not only captivate users but also provide valuable feedback, guide interactions, and make your app memorable. In this guide, we’ll delve into the realm of advanced animation techniques for iOS app development, exploring key concepts, providing code samples, and sharing expert insights to help you bring your apps to life.
1. Introduction to iOS Animation
1.1 The Importance of Animation
Animation isn’t just about making your app visually appealing – it serves a functional purpose too. Well-implemented animation provides users with visual feedback, helping them understand the result of their actions. From a gentle bounce when pressing a button to indicating loading progress, animation enhances the user experience by making interactions intuitive and delightful.
1.2 Core Animation Framework Overview
At the heart of iOS animation lies the Core Animation framework. This powerful framework provides the foundation for smooth and efficient animations. It operates on a layer-based architecture, allowing you to animate individual views or groups of views. With Core Animation, you can animate a wide range of properties, including position, scale, rotation, opacity, and more.
Here’s a snippet demonstrating how to perform a simple animation using Core Animation:
swift import UIKit class ViewController: UIViewController { @IBOutlet weak var animatedView: UIView! override func viewDidLoad() { super.viewDidLoad() let animation = CABasicAnimation(keyPath: "opacity") animation.fromValue = 1.0 animation.toValue = 0.0 animation.duration = 1.0 animatedView.layer.add(animation, forKey: "opacityAnimation") } }
2. Key Animation Concepts
2.1 Timing and Easing Functions
Controlling the timing of animations is crucial for achieving realistic motion. Easing functions play a significant role in determining the acceleration and deceleration of an animation. iOS provides various built-in easing functions like ease-in, ease-out, linear, and more. Custom easing functions can also be created to achieve specific motion effects.
2.2 Physics-based Animation
For a more natural and organic feel, physics-based animation comes into play. UIKit Dynamics, a part of the UIKit framework, allows you to simulate real-world physics in your animations. You can define behaviors such as gravity, collision, and attachment, enabling views to interact dynamically.
swift import UIKit class ViewController: UIViewController { var animator: UIDynamicAnimator! override func viewDidLoad() { super.viewDidLoad() animator = UIDynamicAnimator(referenceView: view) let square = UIView(frame: CGRect(x: 100, y: 100, width: 50, height: 50)) square.backgroundColor = UIColor.blue view.addSubview(square) let gravity = UIGravityBehavior(items: [square]) animator.addBehavior(gravity) } }
2.3 Layer Transformations
Transformations allow you to modify a view’s scale, rotation, and translation. Combining these transformations can create intricate animations. The CATransform3D class lets you define 3D transformations, adding depth to your animations.
swift let rotationTransform = CATransform3DMakeRotation(CGFloat.pi, 0, 1, 0) let scaleTransform = CATransform3DMakeScale(1.5, 1.5, 1.0) let translationTransform = CATransform3DMakeTranslation(100, 0, 0) let transform = CATransform3DConcat(CATransform3DConcat(rotationTransform, scaleTransform), translationTransform) UIView.animate(withDuration: 1.0) { self.animatedView.layer.transform = transform }
3. Advanced Animation Techniques
3.1 Property Animations
Property animations allow you to animate changes to view properties over time. Alongside the basic animations we’ve seen, more advanced animations can be achieved using keyframes. Keyframe animations enable you to define specific values at certain points in time, resulting in intricate motion.
swift UIView.animateKeyframes(withDuration: 4.0, delay: 0, options: [], animations: { UIView.addKeyframe(withRelativeStartTime: 0.0, relativeDuration: 0.25) { self.animatedView.center.x += 50 } UIView.addKeyframe(withRelativeStartTime: 0.25, relativeDuration: 0.25) { self.animatedView.center.y -= 100 } // More keyframes... }, completion: nil)
3.2 View Controller Transitions
View controller transitions involve animated changes between different screens in your app. Custom view controller transitions can be designed to provide unique and engaging visual effects during navigation. The UIViewControllerAnimatedTransitioning protocol allows you to define custom animations for view controller transitions.
swift class CustomTransitionAnimator: NSObject, UIViewControllerAnimatedTransitioning { func animateTransition(using transitionContext: UIViewControllerContextTransitioning) { // Custom animation logic } func transitionDuration(using transitionContext: UIViewControllerContextTransitioning?) -> TimeInterval { return 1.0 } }
3.3 Interactive Gesture-driven Animation
User interaction can drive animations in response to gestures. The UIViewPropertyAnimator class lets you create interactive animations that can be paused, reversed, or adjusted based on user gestures.
swift let animator = UIViewPropertyAnimator(duration: 1.0, curve: .easeInOut, animations: { // Animation changes }) animator.addCompletion { position in if position == .end { // Animation completed } } animator.startAnimation()
4. Layered Animations
4.1 Creating Complex Animations
Layered animations involve animating multiple views simultaneously to create more complex effects. Coordinated animations can make user interactions feel connected and seamless.
swift UIView.animate(withDuration: 0.5) { self.view1.center.x += 100 } UIView.animate(withDuration: 0.5, delay: 0.2, options: [], animations: { self.view2.center.y -= 50 }, completion: nil)
4.2 Parallax Scrolling Effects
Parallax scrolling adds depth to your app’s interface by moving background elements at different speeds from the foreground elements. This effect creates a visually captivating experience and can be achieved through adjustments in view positions as users scroll.
5. Performance Considerations
5.1 Hardware Acceleration
Leverage hardware acceleration to ensure smooth animations. Utilize the GPU for rendering whenever possible, as it’s optimized for graphics-intensive tasks.
5.2 Rendering and Framerate
Maintain a consistent framerate to provide a polished experience. Strive for 60 frames per second (fps) to achieve fluid animations. Minimize overloading the main thread to avoid animation stuttering.
5.3 Memory Management
Animations can consume memory, especially if they involve complex graphics. Be cautious with retaining references to views, animations, or layers to prevent memory leaks.
6. Expert Tips for Seamless Animation
6.1 Prioritizing Consistency
Consistency in animation styles and speeds throughout your app contributes to a cohesive user experience. Familiarity helps users understand how different elements relate and respond to their interactions.
6.2 Embracing Apple’s Design Guidelines
Apple provides Human Interface Guidelines that offer recommendations on animations. Adhering to these guidelines ensures that your animations align with iOS design principles and feel native to the platform.
6.3 User Customization and Accessibility
Consider offering users the option to customize animation settings. Additionally, ensure that animations are accessible to everyone, including users who might have motion-related sensitivities. Provide options to reduce or disable animations if needed.
Conclusion
In conclusion, advanced animation techniques are a powerful toolset for elevating your iOS apps to the next level. By mastering timing, physics, transformations, and interactive animations, you can create delightful and engaging user experiences that leave a lasting impression. Remember to balance creativity with performance considerations and accessibility to create animations that truly shine on the iOS platform. So go ahead, bring your apps to life through the art of animation!
Table of Contents
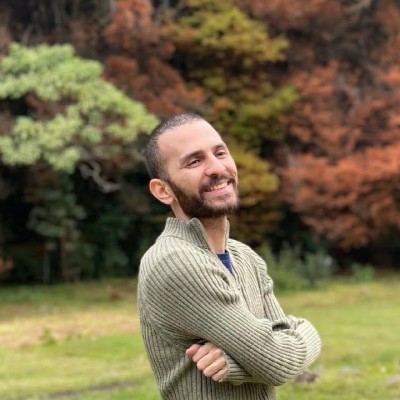
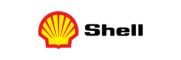