Polishing Your iOS Skills: An Exploration of Advanced Programming Techniques
As a dedicated iOS developer, you’re always striving to make your applications more efficient, faster, and generally better. This journey never ends, because no matter how good you get, there’s always something new to learn or some new technique to adopt. Your continuous quest for learning is exactly what organizations look for when they aim to hire iOS developers. This article is a deep dive into some advanced iOS programming techniques you might not be using yet, but certainly should consider. Adopting these skills can make you even more desirable to those looking to hire iOS developers. We’ll explore concepts like Grand Central Dispatch, protocol-oriented programming, lazy loading, generics, and more. Get ready to boost your iOS game to the next level!
1. Grand Central Dispatch (GCD)
GCD, also known as Dispatch, is a low-level API for managing concurrent operations. It can help improve your app’s responsiveness by allowing it to perform multiple tasks at once. Let’s see an example:
```swift let queue = DispatchQueue(label: "com.example.myQueue", qos: .userInteractive) queue.async { // Run a task in the background. let data = loadDataFromDisk() DispatchQueue.main.async { // Update the UI on the main thread. updateUI(with: data) } } ```
In this example, we’re loading data from disk, which could be a slow operation. We’re running it on a background queue to avoid blocking the main thread, which should always remain free to update the UI. Once the data is loaded, we’re switching back to the main thread to update the UI.
2. Protocol-Oriented Programming (POP)
POP is a design pattern that focuses on defining protocols and implementing behavior based on those protocols. This encourages composition over inheritance and provides more flexibility. Let’s see how this might work in practice:
```swift protocol Flyable { func fly() } class Bird: Flyable { func fly() { print("Flap flap flap") } } class Airplane: Flyable { func fly() { print("Zoom zoom zoom") } } let objects: [Flyable] = [Bird(), Airplane()] for object in objects { object.fly() } ```
Here, instead of making `Bird` and `Airplane` subclasses of some superclass `Flyer`, we’ve defined a `Flyable` protocol. Any class that can fly simply conforms to this protocol. This is a simple example, but in more complex programs, POP can provide much more flexibility than traditional inheritance.
3. Lazy Loading
Lazy loading is a strategy where you delay the creation of an object or some other expensive process until it’s needed. Here’s an example:
```swift class ExpensiveClass { init() { print("ExpensiveClass has been initialized.") } } class MyClass { lazy var expensiveObject = ExpensiveClass() } let myObject = MyClass() // ExpensiveClass is not initialized yet. print(myObject.expensiveObject) // Now it is. ```
In this example, `ExpensiveClass` is not initialized when we create an instance of `MyClass`. It’s only initialized when we first access `expensiveObject`. This can save resources if `ExpensiveClass` is not always used.
4. Generics
Generics are a way to write flexible, reusable functions and types that can work with any type. Here’s an example:
```swift func swapTwoValues<T>(_ a: inout T, _ b: inout T) { let temporaryA = a a = b b = temporaryA } var someInt = 3 var anotherInt = 107 swapTwoValues(&someInt, &anotherInt) ```
In this example, `swapTwoValues(_:_:)` is a generic function that can swap the values of two variables, no matter what type those variables are (as long as they’re the same type).
5. Optional Chaining
Optional chaining is a process for querying and calling properties, methods, and subscripts on an optional that might currently be `nil`. If the optional contains a value, the property, method, or subscript call succeeds; if the optional is `nil`, the property, method, or subscript call returns `nil`. Here’s an example:
```swift class Residence { var numberOfRooms = 1 } class Person { var residence: Residence? } let john = Person() if let roomCount = john.residence?.numberOfRooms { print("John's residence has \(roomCount) room(s).") } else { print("Unable to retrieve the number of rooms.") } ```
In this example, because `john.residence` is `nil`, the entire `john.residence?.numberOfRooms` expression also evaluates to `nil`.
Conclusion
By mastering these techniques and incorporating them into your daily development work, you’ll find that you can write cleaner, more efficient, and more maintainable code. This makes you an ideal candidate for companies looking to hire iOS developers. The key to becoming a proficient iOS developer lies in continuously learning and growing, always seeking to push your understanding of the platform and the Swift language to the next level. Whether you’re looking to get hired or hoping to refine your existing skills, these advanced techniques are indispensable. Happy coding!
Table of Contents
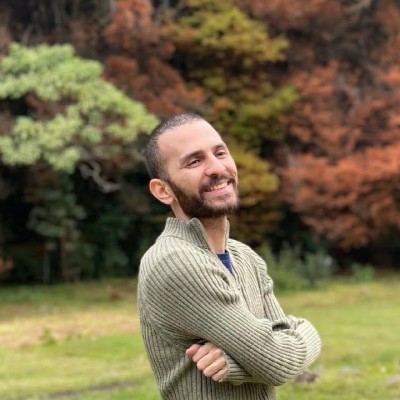
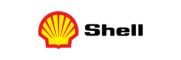