Making iOS App Development Easier with Swift
As technology evolves and consumer preferences shift toward mobile solutions, learning the tools and techniques for effective mobile application development has become vital for any aspiring or professional developer. In this context, the demand to hire iOS developers proficient in Swift has surged significantly. Apple’s iOS operating system powers millions of mobile devices globally, and Swift, its associated programming language, has proven to be a powerful tool for creating robust and efficient iOS applications. This blog post will delve into the power of Swift, a preferred language for those who hire iOS developers, and demonstrate how it has simplified iOS programming. We will examine some key features of Swift and illustrate these points with practical examples.
1. Introduction to Swift
Introduced by Apple in 2014, Swift is a general-purpose, multi-paradigm, compiled programming language designed for iOS, macOS, watchOS, and tvOS app development. It was developed as a more streamlined, powerful, and safer alternative to Objective-C, the earlier language used for Apple platforms. It boasts a modern syntax that is easy to read and write, supports multiple programming paradigms, and comes with powerful tools and frameworks, such as Xcode, SwiftUI, and Combine, to make development faster and easier.
2. The Power of Swift
Swift’s power lies in its simplicity, safety features, speed, and interactive coding environment, which makes iOS programming easy and efficient.
2.1 Simplicity
Swift has a clean syntax which makes it easier to read and write. Its syntax is not cluttered with semicolons or parentheses around conditional statements. Also, method and function calls use the comma-separated list of parameters within parentheses. This simplicity promotes a faster learning curve for new developers.
Example:
In Swift, printing “Hello, World!” is as simple as:
```swift print("Hello, World!") ```
2.2 Safety
Swift includes modern programming features like strong typing, optional types, and automatic memory management, which reduces the chances of encountering bugs in your code. This is a massive boost for developers since these features help prevent errors and enhance code stability.
Example:
Optional types allow variables to not have a value (i.e., to be `nil`). This feature helps prevent runtime crashes, which could occur if a variable is unexpectedly found to be `nil`.
```swift var optionalString: String? = "Hello" print(optionalString == nil) // Prints "false" optionalString = nil print(optionalString == nil) // Prints "true" ```
2.3 Speed
Swift is built to be fast. It includes high-level language features that make it easy to optimize the code and low-level features that make the execution of the code efficient. This combination enables developers to build apps that provide a smooth and responsive user experience.
Example:
The `filter` and `map` high-level functions are typical examples of Swift’s efficient handling of collections.
```swift let numbers = [1, 2, 3, 4, 5, 6] let evenNumbers = numbers.filter { $0 % 2 == 0 } print(evenNumbers) // Prints "[2, 4, 6]" ```
2.4 Interactive Coding Environment (Playgrounds)
Swift provides an interactive coding environment known as Playgrounds. This allows developers to test a new algorithm or graphics routine, with up to a few pages of code, right from within the development environment. This makes coding in Swift a lot more interactive and learning, a lot more fun.
3. Swift and iOS Development
Swift has made iOS development easier and more accessible to more developers. Its seamless compatibility with Cocoa and Cocoa Touch, the foundational frameworks for Apple platforms, allows developers to create powerful iOS apps.
3.1 SwiftUI
SwiftUI, a revolutionary framework introduced in 2019, allows developers to design their app’s UI in a declarative way, meaning they just have to describe the UI and SwiftUI takes care of the rest.
Example:
To create a list with SwiftUI:
```swift import SwiftUI struct ContentView: View { var body: some View { List { Text("First Row") Text("Second Row") Text("Third Row") } } } ```
3.2 Combine
Combine is a powerful framework that makes it easier to handle asynchronous events in a Swift application. It provides tools to process sequences of values over time, which is useful in situations like networking, where data is received at different times.
Example:
This example shows how Combine can be used to fetch data from a network:
```swift import Combine struct User: Codable { let id: Int let name: String } let url = URL(string: "https://api.example.com/users")! let publisher = URLSession.shared.dataTaskPublisher(for: url) .map(\.data) .decode(type: [User].self, decoder: JSONDecoder()) .receive(on: DispatchQueue.main) .sink(receiveCompletion: { completion in switch completion { case .failure(let error): print("Error: \(error)") case .finished: break } }, receiveValue: { users in for user in users { print("\(user.name)") } }) ```
Conclusion
Swift’s power, simplicity, safety features, and modern toolsets make it an excellent choice for iOS programming. Its compatibility with Cocoa and Cocoa Touch, combined with its comprehensive suite of development tools, helps create efficient and high-performing applications. If you’re looking to explore the world of iOS programming, or planning to hire an iOS developer, Swift provides a robust, accessible, and enjoyable gateway. With an iOS developer proficient in Swift, you’re set to create compelling and high-quality applications that stand out in the competitive landscape of the App Store.
Table of Contents
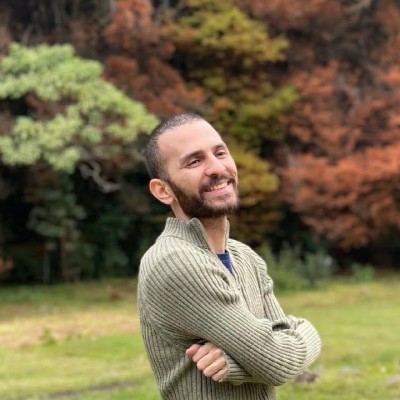
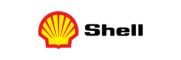