Achieving iOS Excellence: Masterful Techniques for Apple App Development
The world of iOS development is an exciting and dynamic one, brimming with opportunities to build innovative and impactful applications for millions of users. Sometimes, these opportunities might necessitate you to hire iOS developers who are experts in navigating this world. Whether you’re an aspiring developer or a project manager seeking to enhance your team, understanding the nuances of iOS development is vital. You need more than just the basics of coding. To truly shine in this field, you should master the tips and tricks that can help craft elegant, efficient, and user-friendly apps. Here are the essential insights that everyone, from solo developers to those who hire iOS developers, needs in order to master the art of iOS development.
Understand the Language: Swift
When it comes to iOS development, Swift is the go-to language. Apple introduced Swift in 2014, and it has gained wide popularity because of its simplicity, safety, and efficiency. Swift is intuitive and easy to understand, even for beginners.
Tip 1: Using Optionals Wisely
Optionals in Swift help you deal with the absence of a value. They either contain a value or nil. But, incorrect use of optionals can lead to runtime crashes.
Consider this example:
```swift var optionalString: String? = "Hello, World!" print(optionalString!) // Output: Hello, World! optionalString = nil print(optionalString!) // Runtime error: unexpectedly found nil while unwrapping an Optional value ```
In the above code, force unwrapping an optional string causes a runtime error. Instead, use optional binding or optional chaining to safely unwrap optionals.
```swift var optionalString: String? = "Hello, World!" if let unwrappedString = optionalString { print(unwrappedString) // Output: Hello, World! } else { print("String is nil") } ```
Tip 2: Use Guard Statements
Guard statements allow you to write cleaner and more understandable code by handling error conditions upfront. A guard statement checks a condition, and if it doesn’t meet, it exits the current scope.
```swift func greet(person: [String: String]) { guard let name = person["name"] else { print("Could not find a name.") return } print("Hello, \(name)!") } ```
Leverage Interface Builder
Interface Builder is a powerful visual tool for designing and testing user interfaces without writing any code. It’s important to leverage Interface Builder to make your app development process easier.
Tip 3: Use Auto Layout
Auto Layout dynamically calculates the size and position of all the views in your view hierarchy based on constraints. This helps your app’s UI to look consistent across different device sizes and orientations. Here’s a simple trick to make a view maintain its aspect ratio:
– Select the view in Interface Builder.
– In the Size Inspector, add a new ratio constraint.
– Enter 1:1 in the Ratio field.
Tip 4: Prototype Cells for UITableView and UICollectionView
Prototype cells can be used to easily design and customize cells directly in the storyboard. This helps to visualize how the cell looks in the context of the rest of the UI. To add a prototype cell:
– Select the UITableView or UICollectionView.
– In the Attributes Inspector, increase the Prototype Cells count.
– Design the cell directly in the storyboard.
Code Management
Good code management practices are key to maintaining your app in the long run.
Tip 5: MVC – Model View Controller
MVC is a software architectural pattern for implementing user interfaces. It separates an application into three interconnected parts: the model, the view, and the controller.
– Model: Handles data and business logic.
– View: Presents the data to the user.
– Controller: Manages the communication between the Model and View.
By following MVC, you can create more manageable, flexible, and testable code.
Tip 6: Use Git for Version Control
Git allows you to track changes in your code, collaborate with other developers, and revert to earlier versions of your project if necessary. Make sure to commit often and write clear, meaningful commit messages. This makes it easier to understand the history of your project and debug when necessary.
Use iOS SDKs Wisely
iOS SDKs are software libraries that provide features to accomplish various tasks. Using them wisely can save a lot of development time.
Tip 7: Core Data for Persistence
Core Data is a powerful framework for managing and persisting your app’s data. It provides object graph and persistence which makes it easier to work with data.
```swift let appDelegate = UIApplication.shared.delegate as! AppDelegate let context = appDelegate.persistentContainer.viewContext let entity = NSEntityDescription.entity(forEntityName: "Person", in: context)! let person = NSManagedObject(entity: entity, insertInto: context) person.setValue("John", forKey: "name") ```
Tip 8: Use Grand Central Dispatch (GCD) for Concurrency
iOS apps are event-driven. To keep your app responsive, time-consuming tasks should be done asynchronously. The GCD provides an efficient way to manage concurrent operations.
```swift DispatchQueue.global(qos: .background).async { // Time-consuming task here DispatchQueue.main.async { // Update UI here } } ```
Testing and Debugging
Effective testing and debugging are essential to ensure your app runs flawlessly.
Tip 9: Leverage Xcode’s Debugging Tools
Xcode offers powerful debugging tools, including the debugger, view debugger, and various gauges that provide real-time statistics of CPU, memory, energy, and more. Familiarize yourself with these tools to troubleshoot issues effectively.
Tip 10: Unit Testing and UI Testing
Testing is a crucial part of the development process. Xcode provides the XCTest framework for unit testing and UI testing. Unit tests ensure that individual components of your app work as expected, while UI tests simulate user interaction and ensure that the whole app works as expected.
```swift import XCTest @testable import YourApp class YourAppTests: XCTestCase { func testExample() { // Your test code here } } ```
Conclusion
Mastering iOS development is indeed a journey, not a destination. For some projects, you might find it beneficial to hire iOS developers to boost your productivity. These professionals come equipped with the knowledge of the tips and tricks discussed here, and they can help you expedite the development process. But remember, whether you’re developing on your own or with a team, practice is key. Keep building, keep learning, and keep pushing the boundaries of what you and your team can do. Happy coding!
Table of Contents
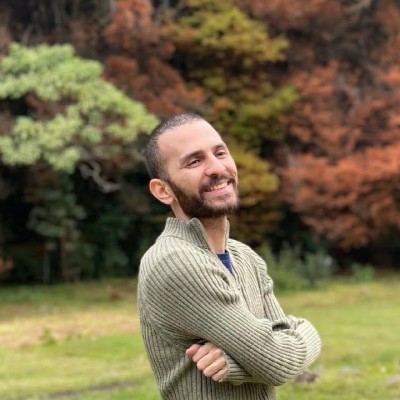
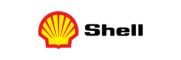