Building Audio Effects in iOS: AudioUnit and AudioToolbox Frameworks
When it comes to creating immersive audio experiences in iOS apps, developers have a powerful arsenal at their disposal: the AudioUnit and AudioToolbox frameworks. These frameworks empower developers to integrate audio effects seamlessly, enhancing user experiences across a wide range of applications, from music production tools to gaming apps. In this guide, we’ll delve into the intricacies of building audio effects in iOS using these frameworks, exploring their capabilities and providing practical examples to illustrate their usage.
Understanding AudioUnit and AudioToolbox Frameworks
Before diving into the implementation details, let’s take a moment to understand the two frameworks at the heart of iOS audio processing:
- AudioUnit Framework: AudioUnit is a powerful framework that allows developers to create and manipulate audio units, which are modular components responsible for generating, processing, or analyzing audio signals. With AudioUnit, developers can implement custom audio processing algorithms, ranging from simple filters to complex synthesizers.
- AudioToolbox Framework: AudioToolbox provides a set of high-level APIs for handling audio data and performing common audio tasks, such as playback, recording, and format conversion. While not as low-level as AudioUnit, AudioToolbox simplifies audio-related operations, making it ideal for scenarios where rapid development is paramount.
Building Audio Effects with AudioUnit
AudioUnit offers unparalleled flexibility for building custom audio effects tailored to specific requirements. Let’s explore a practical example of implementing a reverb effect using AudioUnit:
- Initializing the AudioUnit: First, we need to initialize the AudioUnit instance responsible for applying the reverb effect. We can accomplish this by creating an instance of the AVAudioUnitReverb class and configuring its parameters, such as room size and wet/dry mix.
- Connecting Audio Sources: Next, we connect the audio source, such as a microphone input or audio file, to the input of the reverb AudioUnit. This can be achieved using the AVAudioEngine class to create a signal processing graph and establish connections between audio nodes.
- Processing Audio Data: Once the audio source is connected to the reverb AudioUnit, we process the incoming audio data in real-time, applying the reverb effect to create a sense of spatial depth and immersion. The processed audio can then be routed to the output for playback or further processing.
Implementing Audio Effects with AudioToolbox
While AudioUnit provides fine-grained control over audio processing, AudioToolbox offers a more streamlined approach for implementing common audio effects. Let’s consider an example of adding a distortion effect using AudioToolbox:
- Initializing the Audio Processing Graph: We start by creating an instance of the AUGraph class, which represents the audio processing graph containing various audio units. We add audio units for playback, distortion, and output, connecting them to form a processing chain.
- Configuring the Distortion Effect: We configure the parameters of the distortion audio unit, such as the type of distortion (e.g., overdrive, fuzz) and the intensity of the effect. These parameters can be adjusted dynamically to achieve the desired sound characteristics.
- Processing Audio Data: As audio data flows through the audio processing graph, the distortion effect is applied to alter the waveform, introducing harmonic overtones and creating a gritty, distorted sound. The processed audio is then routed to the output for playback.
Conclusion
In conclusion, the AudioUnit and AudioToolbox frameworks provide robust tools for building immersive audio effects in iOS apps. Whether you’re creating a music production app, a game with dynamic soundscapes, or a voice processing tool, these frameworks offer the flexibility and performance needed to bring your audio vision to life.
External Resources:
- Apple’s official documentation on AudioUnit Framework
- iOS Audio Programming Guide by Chris Adamson
- Ray Wenderlich’s tutorial on AudioToolbox Framework
Unlock the full potential of audio in your iOS apps and elevate the user experience with the power of AudioUnit and AudioToolbox frameworks. Happy coding!
Table of Contents
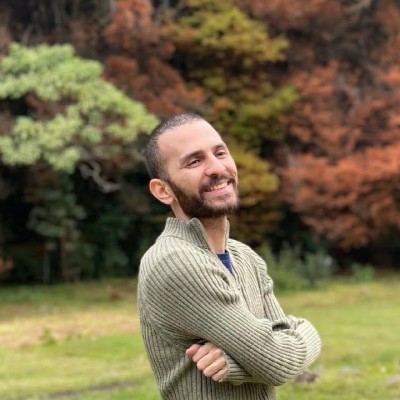
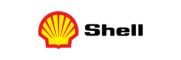