Building Barcode and QR Code Scanners in iOS: AVFoundation Framework
In the ever-evolving world of mobile technology, barcode and QR code scanners have become an integral part of our daily lives. From making purchases to accessing information quickly, these scanners have proven to be efficient and convenient. If you’re an iOS developer looking to integrate barcode and QR code scanning capabilities into your app, you’re in the right place. In this guide, we’ll explore how to build robust code scanners using the AVFoundation framework in iOS.
Table of Contents
1. Introduction to AVFoundation Framework
AVFoundation is a powerful multimedia framework provided by Apple that enables developers to work with audio, video, and capture devices on iOS and macOS. It includes classes and protocols for capturing, processing, and outputting media data. One of the notable features of AVFoundation is its ability to interact with the device’s camera and capture real-time data, making it the perfect choice for building barcode and QR code scanners.
2. Setting Up the Xcode Project
Let’s start by creating a new Xcode project. Open Xcode and select “Create a new Xcode project.” Choose the “Single View App” template, name your project, and select your preferred settings. Once the project is created, make sure to request camera access in the Info.plist file by adding the “Privacy – Camera Usage Description” key with a corresponding message describing why your app needs camera access.
3. Creating a Basic Barcode Scanner
3.1. Importing AVFoundation
In order to use AVFoundation, import it at the top of your view controller file:
swift import AVFoundation
3.2. Initializing the Capture Session
The capture session is the core component that manages the data flow from the input devices (like the camera) to the output. Initialize it in your view controller:
swift var captureSession: AVCaptureSession! func setupCaptureSession() { captureSession = AVCaptureSession() }
3.3. Configuring the Camera Input
Add a function to configure and add the camera input:
swift func configureCameraInput() { guard let captureDevice = AVCaptureDevice.default(for: .video) else { print("No camera available.") return } do { let input = try AVCaptureDeviceInput(device: captureDevice) if captureSession.canAddInput(input) { captureSession.addInput(input) } } catch { print("Error setting up camera input: \(error.localizedDescription)") } }
3.4. Configuring Metadata Output
Set up metadata output to handle the code scanning process:
swift func configureMetadataOutput() { let metadataOutput = AVCaptureMetadataOutput() if captureSession.canAddOutput(metadataOutput) { captureSession.addOutput(metadataOutput) metadataOutput.setMetadataObjectsDelegate(self, queue: DispatchQueue.main) metadataOutput.metadataObjectTypes = [.qr, .ean13, .ean8, .code128] // Add more types as needed } }
3.5. Implementing AVCaptureMetadataOutputObjectsDelegate
Conform to the AVCaptureMetadataOutputObjectsDelegate to handle scanned metadata:
swift extension ViewController: AVCaptureMetadataOutputObjectsDelegate { func metadataOutput( _ output: AVCaptureMetadataOutput, didOutput metadataObjects: [AVMetadataObject], from connection: AVCaptureConnection ) { // Process the scanned metadata objects } }
4. Enhancing the Scanner for QR Codes
Now that we have the basic barcode scanner in place, let’s enhance it to specifically handle QR codes.
4.1. Focusing on QR Codes
Modify the configureMetadataOutput function to focus only on QR codes:
swift func configureMetadataOutputForQR() { let metadataOutput = AVCaptureMetadataOutput() if captureSession.canAddOutput(metadataOutput) { captureSession.addOutput(metadataOutput) metadataOutput.setMetadataObjectsDelegate(self, queue: DispatchQueue.main) metadataOutput.metadataObjectTypes = [.qr] } }
4.2. Displaying the Scanned QR Code
In the metadataOutput delegate method, extract the QR code data and handle it accordingly:
swift func metadataOutput( _ output: AVCaptureMetadataOutput, didOutput metadataObjects: [AVMetadataObject], from connection: AVCaptureConnection ) { guard let qrCode = metadataObjects.first as? AVMetadataMachineReadableCodeObject, let codeValue = qrCode.stringValue else { return } print("Scanned QR code: \(codeValue)") }
5. Customizing the User Experience
A great user experience is essential for any app. Let’s explore how to customize the scanner’s appearance.
5.1. Adding a Preview Layer
Display the camera feed using a AVCaptureVideoPreviewLayer:
swift func setupPreviewLayer() { let previewLayer = AVCaptureVideoPreviewLayer(session: captureSession) previewLayer.videoGravity = .resizeAspectFill previewLayer.frame = view.layer.bounds view.layer.insertSublayer(previewLayer, at: 0) }
5.2. Adding a Scanner Overlay
Create a transparent overlay to guide users in positioning the code:
swift func addScannerOverlay() { let overlayView = UIView() overlayView.layer.borderColor = UIColor.green.cgColor overlayView.layer.borderWidth = 2.0 view.addSubview(overlayView) // Customize overlay position and size as needed }
6. Handling Scanned Data
With the basic setup and customization in place, it’s time to handle the data captured from the codes.
6.1. Launching Actions
Based on the scanned data, you can trigger various actions:
swift func handleScannedData(_ data: String) { // Perform actions like opening URLs, displaying information, etc. }
7. Error Handling and Edge Cases
As with any feature, error handling is crucial for a smooth user experience.
7.1. Permission and Device Checks
Ensure proper permissions and device availability:
swift func checkCameraAuthorization() { switch AVCaptureDevice.authorizationStatus(for: .video) { case .authorized: setupCaptureSession() case .notDetermined: AVCaptureDevice.requestAccess(for: .video) { granted in if granted { self.setupCaptureSession() } else { print("Camera access denied.") } } case .denied, .restricted: print("Camera access denied.") @unknown default: fatalError("Unhandled case.") } }
7.2. Handling Errors
Handle potential errors gracefully:
swift func displayError(_ error: Error) { let alertController = UIAlertController( title: "Error", message: error.localizedDescription, preferredStyle: .alert ) alertController.addAction(UIAlertAction(title: "OK", style: .default)) present(alertController, animated: true) }
Conclusion
Congratulations! You’ve successfully built a barcode and QR code scanner using the AVFoundation framework in iOS. You’ve learned how to set up a capture session, configure camera input, process metadata, and provide a user-friendly interface. Remember, this is just the beginning. You can further refine and expand your scanner’s capabilities, integrate it into your existing app, and make it an essential feature that enhances user interactions.
By following this guide and understanding the fundamentals of AVFoundation, you’re equipped to create powerful, efficient, and user-friendly code scanning apps that cater to the needs of your users in today’s fast-paced digital world.
Remember, practice makes perfect. Experiment with different settings, customize the user interface, and explore additional features to elevate your scanner app to the next level. Happy coding!
Table of Contents
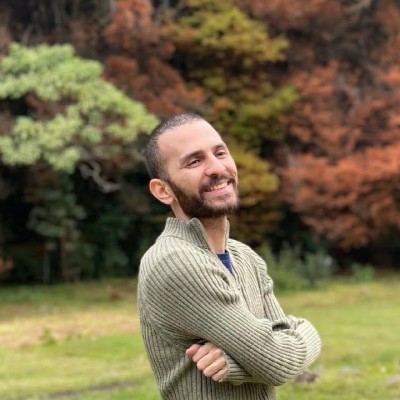
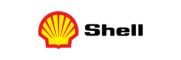