Building Adaptive User Interfaces in iOS: Size Classes Demystified
In the ever-evolving landscape of mobile devices, creating user interfaces that cater to various screen sizes and orientations is a critical challenge for app developers. Apple’s iOS ecosystem spans a multitude of devices, from compact iPhones to expansive iPads, each with its own unique screen dimensions. To tackle this challenge, iOS developers have at their disposal a powerful tool known as “Size Classes.” In this blog post, we will unravel the concept of size classes and delve into how they empower developers to build adaptive user interfaces for a diverse range of iOS devices.
1. Understanding the Need for Adaptive Interfaces
Gone are the days when developers could design interfaces with fixed dimensions to fit a single device. In today’s dynamic environment, users expect apps to seamlessly adapt to their device’s screen size and orientation, ensuring a consistent and visually appealing experience across the board. This is where adaptive user interfaces come into play.
2. The Challenge of Device Diversity
With iPhones, iPads, and even newer form factors like the iPad Pro, developers face a significant challenge in accommodating various screen sizes and orientations. A layout that looks perfect on an iPhone 8 might appear cramped on an iPhone SE or get lost in the expanse of an iPad Pro. This is precisely where size classes offer a solution.
3. Introducing Size Classes
Size classes are an ingenious concept introduced by Apple to enable adaptive layouts in iOS apps. They provide a way to categorize different device sizes into a limited set of classes, simplifying the process of designing interfaces that look and function well on a wide range of devices. The two primary size classes are Regular and Compact, and they are applicable to both horizontal and vertical dimensions.
3.1 Regular and Compact Size Classes
- Regular: Devices with ample screen space fall under this category. iPads, particularly in landscape mode, are a typical example of devices that fall into the “Regular” size class. When designing for the regular size class, you have more space to accommodate additional content and features.
- Compact: Devices with limited screen space are considered “Compact.” This includes most iPhones and iPads in portrait mode. Designing for the compact size class requires optimizing layouts for constrained space.
3.2 Combining Size Classes
Size classes aren’t limited to just Regular and Compact; they can be combined to create a more nuanced layout differentiation. For instance, an iPad in portrait mode might be categorized as Compact width and Regular height. By understanding and leveraging these combinations, developers can create finely-tuned layouts for specific scenarios.
Implementing Adaptive Layouts with Size Classes
Let’s dive into some practical implementation steps to demonstrate how size classes can be used to build adaptive user interfaces in iOS.
1. Adaptive Constraints
Auto Layout, Apple’s layout system, plays a crucial role in building adaptive interfaces. By defining adaptive constraints based on size classes, you can ensure that your UI elements adapt gracefully to different screen sizes and orientations.
swift // Define constraints for regular width and compact height (e.g., iPad in portrait) if traitCollection.horizontalSizeClass == .regular && traitCollection.verticalSizeClass == .compact { // Apply constraints suitable for this size class } else { // Apply default constraints for other size classes }
2. Conditional UI
Size classes also enable you to conditionally load different UI components based on the device’s characteristics. This is particularly useful when you want to present different features or layouts for devices with more screen real estate.
swift if traitCollection.horizontalSizeClass == .regular { // Load and display additional content for iPads } else { // Display default content for other devices }
3. Adaptive Fonts
Text readability is crucial across all devices. Size classes can be used to adjust font sizes based on the available screen space, ensuring optimal legibility.
swift if traitCollection.horizontalSizeClass == .compact { label.font = UIFont.systemFont(ofSize: 14) } else { label.font = UIFont.systemFont(ofSize: 18) }
Conclusion
In the realm of iOS app development, creating adaptive user interfaces is no longer a luxury but a necessity. With the wide array of devices in the market, ensuring that your app looks and functions flawlessly across them all can be a daunting task. This is where size classes step in, offering a simplified yet powerful approach to building adaptive layouts.
By understanding the concepts of regular and compact size classes, combining them intelligently, and implementing adaptive layouts through techniques like adaptive constraints, conditional UI, and adaptive fonts, you can craft a user interface that seamlessly adapts to the diverse iOS ecosystem.
So, the next time you embark on an iOS app development journey, remember the magic that size classes bring to the table. Embrace them, and watch your app shine on every device, big or small, in any orientation. Happy coding!
Table of Contents
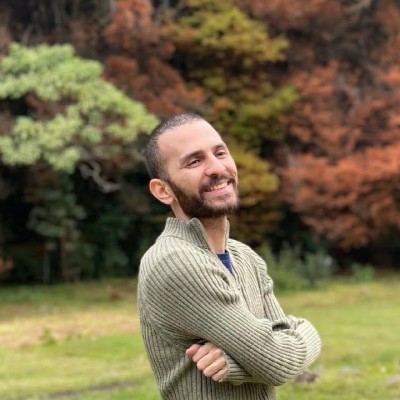
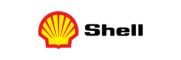