Building Cross-Platform Apps with React Native and iOS
In today’s fast-paced tech landscape, developing mobile applications that cater to both iOS and Android users is a must. React Native, a powerful framework developed by Facebook, has emerged as a game-changer in the realm of cross-platform app development. This blog post delves into the world of React Native and iOS development, uncovering the benefits, tools, and techniques that make building cross-platform apps a breeze.
Table of Contents
1. Why Cross-Platform Apps Matter:
Before we dive into the specifics, let’s understand why cross-platform app development is crucial. In a world where users are spread across different platforms and devices, offering a consistent experience is essential. However, maintaining separate codebases for iOS and Android apps can be time-consuming and error-prone. This is where React Native comes to the rescue.
2. The Power of React Native:
React Native allows developers to create mobile apps using a single codebase that works seamlessly on both iOS and Android platforms. It achieves this through a concept called “native components,” which enable developers to write code in JavaScript while rendering native UI components. This not only ensures a native-like performance but also streamlines the development process.
3. Setting Up Your Development Environment:
Let’s get started by setting up our development environment for building React Native apps on iOS.
Step 1: Install Node.js and npm:
Make sure you have Node.js and npm (Node Package Manager) installed on your machine. You can download them from the official Node.js website.
Step 2: Install React Native CLI:
Install the React Native Command Line Interface (CLI) globally using npm. This CLI tool will aid in creating, building, and managing React Native projects.
bash npm install -g react-native-cli
Step 3: Install Xcode:
For iOS development, you’ll need Xcode, Apple’s integrated development environment. You can download Xcode from the Mac App Store.
Step 4: Create a New React Native Project:
Create a new React Native project using the following command:
bash react-native init MyAwesomeApp
4. Coding a Simple iOS App:
Now that we have our project set up, let’s code a simple iOS app using React Native.
Step 1: Navigate to Your Project Directory:
bash cd MyAwesomeApp
Step 2: Open App.js:
Replace the code in App.js with the following:
jsx import React from 'react'; import { View, Text, StyleSheet } from 'react-native'; const App = () => { return ( <View style={styles.container}> <Text style={styles.text}>Hello, iOS with React Native!</Text> </View> ); }; const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', }, text: { fontSize: 20, fontWeight: 'bold', }, }); export default App;
Step 3: Run the App on iOS Simulator:
Use the following command to run the app on the iOS simulator:
bash react-native run-ios
5. Benefits of React Native for iOS Development:
5.1. Code Reusability:
One of the standout advantages of React Native is its code reusability. Since you’re working with a single codebase, most of the logic and components can be shared between your iOS and Android apps. This dramatically reduces development time and ensures consistent functionality across platforms.
5.2. Native Performance:
React Native doesn’t sacrifice performance for the sake of cross-platform compatibility. It compiles down to native code and utilizes native components, resulting in apps that are almost indistinguishable from those built using native languages like Swift or Objective-C.
5.3. Hot Reloading:
React Native offers a fantastic feature called hot reloading. This allows you to instantly see the changes you make in the code on the simulator or device, without the need to recompile the entire app. This feature greatly speeds up the development cycle.
5.4. Strong Developer Community:
The React Native community is vast and active, which means you’ll have access to a plethora of open-source libraries, tools, and resources. If you encounter an issue, chances are someone else has faced it too and has likely provided a solution.
Conclusion
In this blog post, we’ve scratched the surface of building cross-platform apps with React Native and iOS. We’ve learned about the advantages of cross-platform development, explored the power of React Native in enabling code reusability and native performance, and even created a simple iOS app using React Native.
With React Native, the world of cross-platform app development is more accessible than ever. Whether you’re a seasoned developer or just starting, React Native empowers you to create impressive, performant, and consistent mobile applications that cater to iOS and Android users alike. So, why not dive into the world of React Native and revolutionize your app development process?
Remember, this is just the beginning. As you continue your journey, you’ll uncover more advanced techniques, optimize your app’s performance, and create even more engaging and feature-rich experiences for your users. Happy coding!
Table of Contents
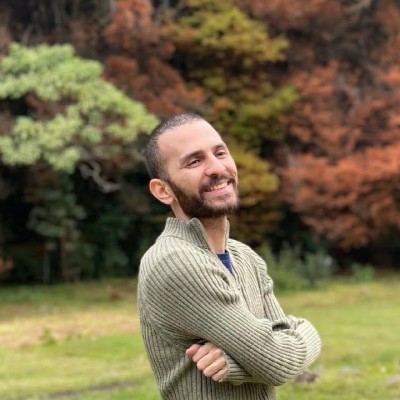
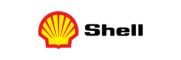