Building iBeacon Apps in iOS: Location-Based Experiences
In the rapidly evolving landscape of mobile technology, location-based experiences have become a powerful tool for businesses and developers alike. iBeacon, a technology developed by Apple, has paved the way for creating engaging and context-aware iOS applications that offer users personalized interactions based on their physical proximity to beacons.
Table of Contents
This blog post will guide you through the process of building iBeacon apps for iOS, allowing you to harness the potential of location-based experiences. Whether you’re developing a retail app, a museum guide, or an event companion, iBeacon technology can add a new dimension to your app’s capabilities.
1. What Are iBeacons?
Before diving into the nitty-gritty of building iBeacon apps, let’s understand what iBeacons are and how they work.
1.1. iBeacon Basics
iBeacons are small, low-cost devices that transmit Bluetooth Low Energy (BLE) signals to nearby iOS devices. These signals contain unique identifiers that help iOS apps determine their proximity to specific iBeacons. The key components of an iBeacon signal are:
- UUID (Universally Unique Identifier): A 128-bit value that identifies a specific beacon network. All beacons within the same network share the same UUID.
- Major Value: A 16-bit value that distinguishes a group of beacons within the same network.
- Minor Value: A 16-bit value that identifies an individual beacon within a group.
These identifiers allow your iOS app to recognize and differentiate between different iBeacons, enabling you to trigger location-based actions when a user approaches or moves away from a beacon.
1.2. How iBeacons Work
iBeacons continuously broadcast their unique identifier data using BLE technology. When a user’s iOS device comes into range of an iBeacon, the app can detect the beacon’s signal and calculate the user’s proximity to it. This proximity can be categorized into three main regions:
- Immediate: The user is very close to the beacon, typically within a few inches.
- Near: The user is within a few meters of the beacon.
- Far: The user is at a greater distance from the beacon, usually more than 10 meters.
Now that you have a fundamental understanding of iBeacons, let’s explore how to build iOS apps that leverage this technology for immersive location-based experiences.
2. Setting Up Your Development Environment
Before you start coding your iBeacon app, you’ll need to set up your development environment. Ensure you have the following prerequisites:
2.1. Hardware
- Mac computer (iBeacon app development is specific to macOS)
- iOS device for testing (optional but recommended)
- iBeacon hardware (e.g., Estimote, Kontakt.io, or compatible BLE devices)
2.2. Software
- Xcode: Download and install the latest version of Xcode from the Mac App Store.
- iOS Development Account: You’ll need an Apple Developer account to deploy your app to a physical iOS device or distribute it on the App Store.
- CocoaPods (optional but recommended): CocoaPods is a dependency manager for Swift and Objective-C projects. You can install it using RubyGems by running gem install cocoapods in your Terminal.
3. Creating an iBeacon App
Now that your development environment is set up, let’s create a simple iBeacon app step by step. We’ll build a basic iOS app that detects and responds to iBeacons in its vicinity.
Step 1: Create a New Xcode Project
- Open Xcode and click on “Create a new Xcode project.”
- Choose the “Single View App” template and click “Next.”
- Fill in your project details, such as the product name and organization identifier, and choose your preferred language (Swift or Objective-C). Click “Next.”
- Select the location where you want to save your project and click “Create.”
Step 2: Add Core Location Framework
To work with iBeacons, we need to use the Core Location framework. Follow these steps to add it to your project:
- In Xcode’s Project Navigator, select your project at the top.
- Under “TARGETS,” select your app target.
- Go to the “General” tab, scroll down to the “Frameworks, Libraries, and Embedded Content” section, and click the “+” button.
- Search for “Core Location” in the search bar and select it from the list. Click “Add.”
Step 3: Request Location Permissions
Your app needs user permission to access their device’s location. To do this, you should add the necessary descriptions and keys to your app’s Info.plist file. Here’s how:
- In the Project Navigator, find your Info.plist file and open it.
- Add the “Privacy – Location When In Use Usage Description” key and provide a user-friendly description of why your app needs location access.
- Add the “NSLocationWhenInUseUsageDescription” key with a brief description of your location request.
Step 4: Implement iBeacon Scanning
Now, it’s time to write the code for scanning and detecting iBeacons. We’ll implement this in the ViewController.swift file. Here’s a basic example:
swift import UIKit import CoreLocation class ViewController: UIViewController, CLLocationManagerDelegate { let locationManager = CLLocationManager() override func viewDidLoad() { super.viewDidLoad() locationManager.delegate = self locationManager.requestWhenInUseAuthorization() } // MARK: - CLLocationManagerDelegate Methods func locationManager(_ manager: CLLocationManager, didChangeAuthorization status: CLAuthorizationStatus) { if status == .authorizedWhenInUse { startScanning() } } func startScanning() { let uuid = UUID(uuidString: "YOUR_BEACON_UUID") let beaconRegion = CLBeaconRegion(uuid: uuid!, identifier: "Your Beacon") locationManager.startMonitoring(for: beaconRegion) locationManager.startRangingBeacons(in: beaconRegion) } // Implement beacon detection and ranging methods here }
Replace “YOUR_BEACON_UUID” with the UUID of your iBeacon. This code initializes the CLLocationManager, requests location authorization, and starts scanning for beacons with the specified UUID.
Step 5: Handle Beacon Detection
To handle beacon detection and ranging, implement the following methods within your ViewController:
swift func locationManager(_ manager: CLLocationManager, didRangeBeacons beacons: [CLBeacon], in region: CLBeaconRegion) { for beacon in beacons { switch beacon.proximity { case .immediate: // Handle immediate proximity break case .near: // Handle near proximity break case .far: // Handle far proximity break case .unknown:swift func locationManager(_ manager: CLLocationManager, didRangeBeacons beacons: [CLBeacon], in region: CLBeaconRegion) { for beacon in beacons { switch beacon.proximity { case .immediate: // Handle immediate proximity break case .near: // Handle near proximity break case .far: // Handle far proximity break case .unknown: // Handle unknown proximity break @unknown default: fatalError("New beacon proximity case added. Update the code.") } } } func locationManager(_ manager: CLLocationManager, didEnterRegion region: CLRegion) { // User entered the beacon's region } func locationManager(_ manager: CLLocationManager, didExitRegion region: CLRegion) { // User exited the beacon's region } // Handle unknown proximity break @unknown default: fatalError("New beacon proximity case added. Update the code.") } } } func locationManager(_ manager: CLLocationManager, didEnterRegion region: CLRegion) { // User entered the beacon's region } func locationManager(_ manager: CLLocationManager, didExitRegion region: CLRegion) { // User exited the beacon's region }
In the didRangeBeacons method, you can define actions to be taken based on the user’s proximity to the beacon. For example, you might display a special offer when the user is in immediate proximity to a retail beacon.
Step 6: Testing Your App
To test your iBeacon app, follow these steps:
- Connect your iOS device to your Mac.
- Select your device as the deployment target in Xcode.
- Build and run your app on your iOS device.
- Ensure that your iBeacon hardware is nearby and transmitting.
- Observe your app’s behavior as you move closer to or farther away from the beacon.
4. Best Practices and Tips
As you dive deeper into building iBeacon apps, consider these best practices and tips to create effective location-based experiences:
4.1. Optimize Beacon Placement
Strategically place your iBeacons to ensure a reliable and accurate detection range. Factors like physical obstructions and interference can affect beacon signals.
4.2. Use Background Monitoring
To provide a seamless experience, enable background monitoring of beacons. This allows your app to react to beacon events even when it’s not actively running.
4.3. Design User-Friendly Prompts
When requesting location permissions, explain why your app needs them and how users will benefit from enabling them. Clear and concise prompts increase user trust.
4.4. Test Extensively
Test your app in various real-world scenarios to ensure accurate beacon detection and a smooth user experience.
4.5. Implement Analytics
Use analytics to gain insights into how users interact with your iBeacon app. This data can help you refine your location-based experiences over time.
Conclusion
Building iBeacon apps in iOS opens up a world of possibilities for creating immersive location-based experiences. Whether you’re enhancing retail shopping, guiding visitors through a museum, or adding interactive elements to events, iBeacon technology empowers you to engage users in innovative ways.
By following the steps outlined in this guide and adhering to best practices, you can create compelling iOS apps that leverage iBeacons to deliver personalized, context-aware experiences. So, start exploring the world of location-based interactions and take your iOS app development to the next level with iBeacons. Happy coding!
Table of Contents
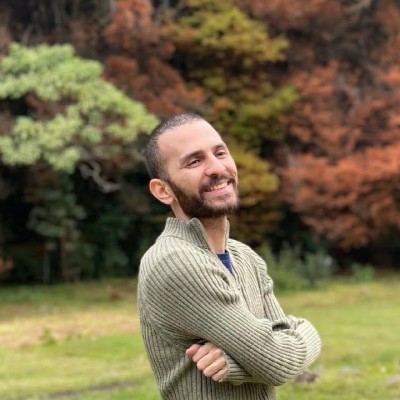
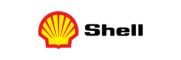