Exploring Core Motion in iOS: Building Motion-Sensitive Apps
In today’s rapidly evolving world of technology, our smartphones have become more than just communication devices. They have transformed into powerful tools that can sense and respond to various inputs, including motion. This has opened up a realm of possibilities for app developers to create innovative and engaging experiences for users. Thanks to the Core Motion framework in iOS, developers can now tap into the device’s built-in sensors to detect and respond to motion, leading to the creation of motion-sensitive apps that range from fitness trackers to interactive games.
Table of Contents
1. Understanding Core Motion: A Brief Overview
At its core, Core Motion is a framework provided by Apple in iOS that gives developers access to the device’s accelerometer, gyroscope, magnetometer, and other motion-related data. This data can be used to gather information about the device’s orientation, rotation rate, acceleration, and even its proximity to other objects. By leveraging this data, developers can build applications that respond to the user’s physical movements, enabling a whole new level of interactivity.
2. Getting Started with Core Motion
To begin utilizing Core Motion in your iOS app, you’ll need to import the Core Motion framework into your project. You can do this by adding the following line of code at the top of your Swift file:
swift import CoreMotion
With the framework imported, you can now create an instance of the CMMotionManager class. This class will be your main gateway to accessing motion-related data. Here’s how you can initialize it:
swift let motionManager = CMMotionManager()
3. Detecting Device Motion
3.1. Accelerometer Data
The accelerometer measures the acceleration force that the device experiences, including the force due to gravity. This data can be used to determine the device’s orientation and movement intensity. To start receiving accelerometer data, you can use the following code:
swift if motionManager.isAccelerometerAvailable { motionManager.accelerometerUpdateInterval = 0.1 // Update interval in seconds motionManager.startAccelerometerUpdates(to: .main) { data, error in if let acceleration = data?.acceleration { // Process acceleration data (x, y, z components) } } }
3.2. Gyroscope Data
The gyroscope measures the rotation rate of the device around its three axes. This can be used to track the device’s angular velocity and detect rotational movements. Here’s how you can access gyroscope data:
swift if motionManager.isGyroAvailable { motionManager.gyroUpdateInterval = 0.1 // Update interval in seconds motionManager.startGyroUpdates(to: .main) { data, error in if let rotationRate = data?.rotationRate { // Process rotation rate data (x, y, z components) } } }
3.3. Device Attitude
The device’s attitude refers to its orientation in space, represented as a combination of pitch, roll, and yaw angles. This can be extremely useful for creating augmented reality experiences and other applications that require accurate spatial awareness. To retrieve the device’s attitude, you can use the following code:
swift if motionManager.isDeviceMotionAvailable { motionManager.deviceMotionUpdateInterval = 0.1 // Update interval in seconds motionManager.startDeviceMotionUpdates(to: .main) { data, error in if let attitude = data?.attitude { // Process attitude data (pitch, roll, yaw angles) } } }
4. Building Motion-Sensitive Apps
Now that you have a grasp of how to access motion-related data using Core Motion, let’s explore some exciting possibilities for building motion-sensitive apps:
4.1. Fitness and Health Tracking
Core Motion can be employed to create fitness apps that track the user’s steps, distance traveled, and even estimate calories burned. By continuously monitoring the accelerometer data, you can detect the user’s steps and convert them into meaningful fitness metrics.
4.2. Interactive Gaming
Motion-sensitive games have taken the gaming industry by storm. You can develop games that utilize the device’s gyroscope and accelerometer to control in-game actions. Imagine racing games where players tilt their devices to steer or adventure games where the device orientation affects the player’s viewpoint.
4.3. Virtual Reality and Augmented Reality
For immersive experiences like virtual reality (VR) and augmented reality (AR), accurate motion tracking is crucial. Core Motion can provide the necessary data to track the user’s head movements and render the virtual environment accordingly, enhancing the sense of presence.
4.4. Gesture-Based Interfaces
Motion can also be used to create gesture-based interfaces within apps. By analyzing accelerometer and gyroscope data, you can recognize specific motions or patterns, allowing users to interact with your app through gestures like shaking, flipping, or tapping.
Conclusion
The Core Motion framework in iOS opens up a world of opportunities for creating motion-sensitive apps that captivate users and provide unique and interactive experiences. By harnessing the power of the device’s sensors, developers can design applications that respond to physical movements, revolutionizing industries like fitness, gaming, and augmented reality. So, whether you’re an aspiring developer or a seasoned pro, dive into Core Motion and unlock the potential of motion-sensitive app development. Your users will thank you for the engaging and dynamic experiences you deliver.
In this blog post, we’ve explored the fundamentals of Core Motion, delved into the code snippets for accessing accelerometer, gyroscope, and device attitude data, and discussed some compelling use cases for motion-sensitive apps. The world of motion-driven experiences is at your fingertips; it’s time to bring your creative ideas to life!
Table of Contents
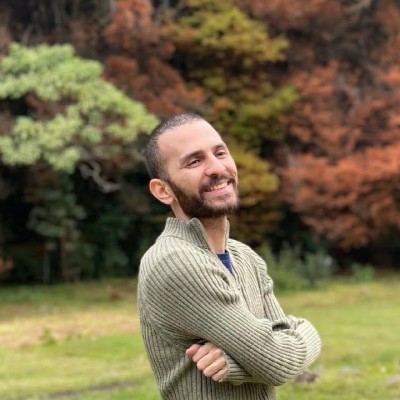
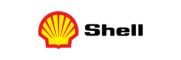