Working with CloudKit in iOS: Syncing Data Across Devices
In today’s interconnected world, the ability to seamlessly synchronize data across multiple devices has become a cornerstone of modern app development. Whether you’re building a to-do list app, a note-taking tool, or a collaborative project management platform, ensuring that users can access and update their data from any of their devices is essential. This is where CloudKit, Apple’s cloud-based framework, comes into play. In this article, we will delve into the intricacies of working with CloudKit in iOS applications to achieve efficient and reliable data synchronization.
Table of Contents
1. Introduction to CloudKit
1.1. What is CloudKit?
CloudKit is a powerful and versatile cloud service provided by Apple, designed to simplify the process of building apps that require cloud-based data storage and synchronization. It provides developers with backend services to manage user authentication, data storage, and real-time collaboration, all seamlessly integrated into the iOS ecosystem.
1.2. Key Benefits
- Seamless Integration: CloudKit is tightly integrated with iOS, macOS, watchOS, and tvOS, making it an ideal choice for building applications that need to run across multiple Apple devices.
- Free Tier: CloudKit offers a generous free tier, allowing developers to get started without immediate cost concerns. It also provides various paid tiers for scaling as your app gains popularity.
- Authentication and Security: User authentication and data security are taken care of by CloudKit, ensuring that user data remains private and secure.
- Real-time Collaboration: CloudKit enables real-time collaboration by offering subscription-based notifications for changes in the database, making it perfect for collaborative apps.
- Offline Support: CloudKit’s built-in caching mechanism allows apps to continue functioning even when the device is offline, ensuring a smooth user experience.
2. Setting Up CloudKit in Your Project
2.1. Enabling CloudKit
Before diving into CloudKit integration, ensure that your Xcode project is set up and connected to your Apple Developer account. Then, enable CloudKit by navigating to your project’s target settings, selecting the “Capabilities” tab, and turning on the “CloudKit” capability.
2.2. Creating a CloudKit Container
CloudKit data is organized within containers, which act as isolated environments for your app’s data. To create a new container, visit the CloudKit Dashboard on the Apple Developer website and add a new container linked to your app’s bundle identifier.
2.3. Defining the Data Schema
CloudKit uses a structured database to store data. Before you start uploading data, define your data schema by creating record types and fields. For instance, if you’re building a to-do list app, you might create a “Task” record type with fields like “title,” “due date,” and “is completed.”
3. Uploading Data to CloudKit
3.1. Creating Records
Records are the fundamental units of data in CloudKit. Each record belongs to a specific record type and contains fields that hold the actual data. In our to-do list app example, each task would be represented as a record.
3.2. Saving Records to the Cloud
To save records to CloudKit, you use the CKDatabase class. You can choose between a public database (for data shared between users) and a private database (for user-specific data). Creating and saving a record involves these steps:
swift import CloudKit // Create a CloudKit record let taskRecord = CKRecord(recordType: "Task") taskRecord["title"] = "Complete Project" taskRecord["dueDate"] = dueDate taskRecord["isCompleted"] = false // Get the default private database let privateDatabase = CKContainer.default().privateCloudDatabase // Save the record to the database privateDatabase.save(taskRecord) { (record, error) in if let error = error { print("Error saving record: \(error.localizedDescription)") } else { print("Record saved successfully") } }
4. Fetching and Displaying CloudKit Data
4.1. Building Queries
To retrieve data from CloudKit, you build queries using the CKQuery class. Queries specify which record types to fetch and optional filters for more specific results.
4.2. Retrieving Records
swift // Create a query for fetching tasks let query = CKQuery(recordType: "Task", predicate: NSPredicate(value: true)) // Perform the query on the private database privateDatabase.perform(query, inZoneWith: nil) { (records, error) in if let error = error { print("Error fetching records: \(error.localizedDescription)") } else if let records = records { // Process fetched records for record in records { // Handle each record } } }
4.3. Displaying Data in UI
Once you have fetched the CloudKit records, you can update your app’s UI to display the retrieved data to the user.
5. Real-time Collaboration with CloudKit
5.1. Subscriptions and Push Notifications
CloudKit supports subscriptions, allowing you to receive push notifications whenever changes occur in the database. This is crucial for real-time collaboration, enabling users to stay updated on changes made by other users.
5.2. Handling Changes in Real Time
swift let subscription = CKQuerySubscription( recordType: "Task", predicate: NSPredicate(value: true), options: [.firesOnRecordCreation, .firesOnRecordUpdate, .firesOnRecordDeletion] ) let notificationInfo = CKSubscription.NotificationInfo() notificationInfo.alertBody = "A task was updated." subscription.notificationInfo = notificationInfo privateDatabase.save(subscription) { (subscription, error) in if let error = error { print("Error creating subscription: \(error.localizedDescription)") } else { print("Subscription created successfully") } }
6. Implementing Offline Support
6.1. Caching Data Locally
CloudKit automatically caches data locally, allowing your app to function offline. When the device regains connectivity, CloudKit automatically syncs the changes with the server.
6.2. Resolving Conflicts
In collaborative apps, conflicts can arise when multiple users edit the same record simultaneously. CloudKit provides conflict management features to help you handle such situations gracefully.
7. Best Practices for Efficient Syncing
7.1. Batch Operations
When dealing with multiple records, consider using batch operations to minimize the number of network requests and improve efficiency.
7.2. Error Handling Strategies
Handle errors gracefully by implementing appropriate error handling mechanisms. CloudKit provides error codes that can help you identify and resolve issues during data syncing.
7.3. Data Privacy and Security
Prioritize data privacy by adhering to Apple’s guidelines for handling user data. Use appropriate permissions and roles to ensure that users have the right level of access to data.
Conclusion
In a world where users expect their data to seamlessly synchronize across their devices, CloudKit emerges as a robust solution for iOS developers. By enabling efficient data storage, real-time collaboration, and offline support, CloudKit empowers developers to create apps that provide a unified experience across Apple devices. As you embark on your journey of integrating CloudKit into your iOS projects, this guide equips you with the fundamental knowledge and code samples needed to build a successful and synchronized app experience.
Table of Contents
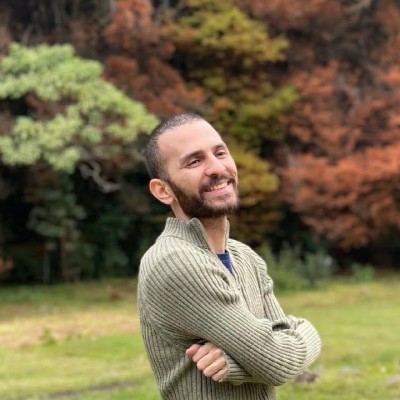
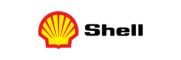