Implementing Handoff in iOS: Continuity Across Apple Devices
In today’s interconnected world, users expect a seamless experience when transitioning between their Apple devices. Apple’s Handoff feature allows developers to provide just that – continuity across iOS, macOS, and watchOS devices. Handoff enables users to start an activity on one device and seamlessly continue it on another. Whether you’re building a productivity app, a media player, or a document editor, implementing Handoff can greatly enhance the user experience. In this comprehensive guide, we’ll explore how to implement Handoff in iOS, complete with code samples and step-by-step instructions.
Table of Contents
1. Understanding Handoff
1.1 What is Handoff?
Handoff is a feature introduced by Apple that allows users to seamlessly transition their activities between Apple devices. This means users can start an activity on one device and continue it on another with minimal effort. Handoff relies on a combination of technologies, including Bluetooth Low Energy (BLE) and iCloud, to enable this smooth transition.
1.2 Why Implement Handoff?
Implementing Handoff in your iOS app offers several benefits:
- Enhanced User Experience: Handoff provides a more fluid and continuous experience for users who own multiple Apple devices.
- Increased Engagement: Users are more likely to engage with your app when they can easily switch between devices, which can lead to higher retention rates.
- Competitive Advantage: Handoff can set your app apart from competitors by offering a feature that simplifies users’ lives.
1.3 Supported Apple Devices
Handoff is supported on a wide range of Apple devices, including:
- iPhone
- iPad
- Mac
- Apple Watch
To implement Handoff successfully, ensure that your app is compatible with these devices.
2. Setting Up Your Xcode Project
Before you can start implementing Handoff in your iOS app, you need to set up your Xcode project correctly.
2.1 Create a New Project
If you haven’t already, create a new Xcode project for your app. Ensure you select the appropriate template (e.g., Single View App) that matches your app’s functionality.
2.2 Configure App IDs and Capabilities
To use Handoff, your app needs to be associated with an App ID in your Apple Developer account. Follow these steps:
- Log in to your Apple Developer account.
- Create an App ID for your app if you haven’t already.
- In Xcode, navigate to your project settings.
- Under the “Signing & Capabilities” tab, select your team and enable automatic signing.
- Add the App ID you created earlier to your project.
2.3 Enable Handoff Capability
To enable Handoff in your app, follow these steps:
- In Xcode, go to your project settings.
- Select your app target.
- Navigate to the “Signing & Capabilities” tab.
- Click the “+ Capability” button.
- Search for “Handoff” and add it to your project.
- Your Xcode project is now set up to implement Handoff.
3. Coding Handoff
Now that your Xcode project is configured, let’s dive into the code and implement Handoff.
3.1 User Activity and NSUserActivity
Handoff revolves around the concept of “User Activity,” represented by the NSUserActivity class in iOS. A User Activity represents a task or piece of content that the user is currently engaged with in your app.
To create a User Activity, follow these steps:
swift import UIKit class ViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() // Create a User Activity let userActivity = NSUserActivity(activityType: "com.yourapp.myactivity") userActivity.title = "My Activity" userActivity.userInfo = ["key": "value"] // Set the User Activity as the current activity self.userActivity = userActivity userActivity.becomeCurrent() } }
In the code above, we create a User Activity with a unique identifier (activityType), a title, and optional user information. We then set this User Activity as the current activity for the view controller.
3.2 Advertise User Activities
To make your User Activities discoverable by other devices, you need to advertise them. This is done using the NSUserActivity instance:
swift import UIKit class ViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() let userActivity = NSUserActivity(activityType: "com.yourapp.myactivity") userActivity.title = "My Activity" userActivity.userInfo = ["key": "value"] self.userActivity = userActivity userActivity.becomeCurrent() // Advertise the User Activity userActivity.isEligibleForHandoff = true userActivity.isEligibleForSearch = true userActivity.isEligibleForPublicIndexing = true } }
In this code, we set the isEligibleForHandoff, isEligibleForSearch, and isEligibleForPublicIndexing properties of the User Activity to make it discoverable through Handoff, Spotlight search, and public indexing, respectively.
3.3 Receive Handoff Data
Now, let’s implement the code that receives Handoff data in another device.
swift import UIKit class SecondViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() // Receive Handoff data NSUserActivity .userActivities(of: "com.yourapp.myactivity") .first .map { activity in if let userInfo = activity.userInfo as? [String: Any] { // Process the received data let value = userInfo["key"] // Do something with the value } } } }
In this code, we retrieve the User Activity with the specified activity type and extract the user information to process it accordingly.
3.4 Handling Handoff Errors
Error handling is crucial when implementing Handoff. You should be prepared to handle cases where Handoff might fail due to various reasons, such as network issues or user permissions.
swift import UIKit class SecondViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() NSUserActivity .userActivities(of: "com.yourapp.myactivity") .first .map { activity in if let userInfo = activity.userInfo as? [String: Any] { let value = userInfo["key"] // Handle the received data // Acknowledge Handoff completion activity.resignCurrent() } } .catch { error in // Handle Handoff error print("Handoff failed: \(error.localizedDescription)") } } }
Here, we use the .catch operator to handle any errors that may occur during the Handoff process.
4. Testing Handoff
4.1 Simulator Testing
You can test Handoff in the iOS Simulator by running your app on multiple simulator instances. Ensure that you are signed in with the same Apple ID on each simulator, and that Handoff is enabled in the simulator settings.
4.2 Device Testing
For thorough testing, it’s essential to test Handoff on physical devices. Ensure that the devices are signed in with the same Apple ID, connected to the same Wi-Fi network, and have Handoff enabled in their settings.
5. Best Practices
Implementing Handoff is just the beginning. To provide an excellent user experience, consider the following best practices:
5.1 Provide a Consistent User Experience
Make sure that the user experience is consistent when transitioning between devices. Users should feel like they are picking up where they left off seamlessly.
5.2 Privacy and Security Considerations
Handle user data with care. Ensure that sensitive information is not exposed during Handoff and implement appropriate security measures.
5.3 Handle App State Changes Gracefully
Users may switch devices at any time, even when your app is in the background or terminated. Handle app state changes gracefully to avoid data loss.
Conclusion
Implementing Handoff in your iOS app can significantly enhance the user experience by providing continuity across Apple devices. By following the steps outlined in this guide and adhering to best practices, you can seamlessly integrate Handoff into your app and delight your users with a more connected experience. Start implementing Handoff today and take your app to the next level of user engagement and satisfaction.
Table of Contents
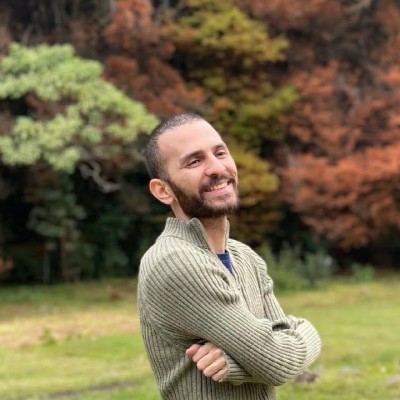
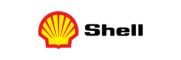