Working with Core Animation in iOS: Layer Transformations and Keyframe Animations
Are you looking to add some eye-catching animations to your iOS app? Look no further than Core Animation. Core Animation is a powerful framework provided by Apple that enables developers to create stunning animations with ease. In this guide, we’ll dive into the world of Core Animation, focusing specifically on layer transformations and keyframe animations.
Layer Transformations
Layer transformations allow you to manipulate the position, scale, rotation, and more of a layer in your iOS app. These transformations can breathe life into your user interface and create a more engaging user experience.
Let’s start with a simple example of translating a layer horizontally:
let layer = CALayer() layer.frame = CGRect(x: 0, y: 0, width: 100, height: 100) layer.backgroundColor = UIColor.blue.cgColor // Translate the layer horizontally by 100 points let translation = CATransform3DMakeTranslation(100, 0, 0) layer.transform = translation
In this example, we create a CALayer with a blue background color and then apply a translation transformation to move the layer 100 points to the right.
But layer transformations are not limited to just translation. You can also rotate and scale layers:
// Rotate the layer by 45 degrees let rotation = CATransform3DMakeRotation(.pi / 4, 0, 0, 1) layer.transform = rotation // Scale the layer by 2x in both the X and Y directions let scale = CATransform3DMakeScale(2.0, 2.0, 1.0) layer.transform = scale
By combining different transformations, you can create complex animations that bring your app to life.
Keyframe Animations
Keyframe animations allow you to specify a series of intermediate values for a property over a period of time. This gives you precise control over how an animation progresses, allowing for more dynamic and expressive animations.
Here’s an example of creating a keyframe animation to animate the position of a layer:
let animation = CAKeyframeAnimation(keyPath: "position") animation.values = [ CGPoint(x: 0, y: 0), CGPoint(x: 100, y: 100), CGPoint(x: 200, y: 0) ] animation.duration = 2.0 layer.add(animation, forKey: "positionAnimation")
In this example, we create a CAKeyframeAnimation for the position property of the layer and specify an array of CGPoint values representing the position of the layer at different points in time. We then set the duration of the animation to 2 seconds and add it to the layer.
External Resources
- Apple’s Core Animation Programming Guide – A comprehensive guide from Apple on getting started with Core Animation.
- Ray Wenderlich’s Core Animation Tutorial – A beginner-friendly tutorial on Core Animation by Ray Wenderlich.
- SwiftLee’s Core Animation in SwiftUI – Learn how to integrate Core Animation with SwiftUI for even more powerful animations.
Conclusion
Core Animation is a versatile framework that allows you to create captivating animations in your iOS app. Whether you’re transforming layers or creating keyframe animations, Core Animation provides the tools you need to bring your app to life. Experiment with different techniques and let your creativity shine!
Happy animating!
Table of Contents
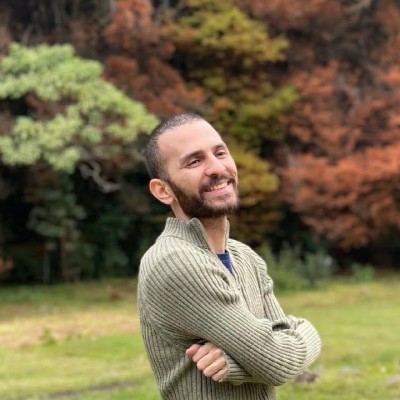
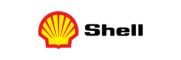