Journey into iOS Core Data: An Exploration of Persistence Mechanisms
Core Data is an object-graph management and persistence framework provided by Apple. It allows developers, including those you might hire as iOS developers, to model their data as objects within their code. This feature significantly reduces the complexity of handling raw data and directly interacting with a database. The beauty of Core Data lies in its ability to store, organize, and retrieve data as needed, thus making it one of the most reliable and efficient persistence solutions on iOS.
The power of Core Data becomes even more evident when dealing with complex object graphs and relationships. So whether you’re an individual developer or looking to hire iOS developers for a larger project, let’s dive into the world of data persistence and explore how Core Data handles it in iOS.
Core Data Stack
Before proceeding with any code, we should first understand the Core Data Stack components:
- Managed Object Model: It’s essentially your database schema. It defines the types of objects (Entities) that can be created and their properties. It’s represented as a `.xcdatamodeld` file in your Xcode project.
- Persistent Store Coordinator: This component is responsible for managing multiple persistent stores. It ensures that data is saved and loaded properly from the disk.
- Managed Object Context: Consider this as a temporary work area in your application where objects are created, edited, and deleted. Any changes in this context aren’t permanent until you save it.
Getting Started with Core Data
Creating a new Xcode project provides an option to include Core Data. Checking that option will automatically add a `.xcdatamodeld` file to your project and add some boilerplate Core Data stack setup in your AppDelegate.
For example, we’ll create a simple note-taking app. The note entity will have `title`, `content`, and `dateCreated` attributes.
In the `.xcdatamodeld` file, add an entity named `Note`. This entity will have three attributes: `title` (String), `content` (String), and `dateCreated` (Date).
Saving Data
Let’s consider how we might save a note:
```swift func saveNote(title: String, content: String) { let appDelegate = UIApplication.shared.delegate as! AppDelegate let context = appDelegate.persistentContainer.viewContext let newNote = NSEntityDescription.insertNewObject(forEntityName: "Note", into: context) newNote.setValue(title, forKey: "title") newNote.setValue(content, forKey: "content") newNote.setValue(Date(), forKey: "dateCreated") do { try context.save() } catch { print("Failed saving") } } ```
In this function, we first get the managed object context. Then we create a new note object and set its values. Finally, we save the context, which will persist our new note to the underlying store.
Fetching Data
Now that we’ve saved some data, let’s fetch it:
```swift func fetchNotes() -> [NSManagedObject]? { let appDelegate = UIApplication.shared.delegate as! AppDelegate let context = appDelegate.persistentContainer.viewContext let fetchRequest = NSFetchRequest<NSFetchRequestResult>(entityName: "Note") fetchRequest.sortDescriptors = [NSSortDescriptor(key: "dateCreated", ascending: false)] do { let result = try context.fetch(fetchRequest) return result as? [NSManagedObject] } catch { print("Failed fetching") return nil } } ```
This function sends a fetch request to the managed object context for all Note entities. We also added a sort descriptor to get the notes sorted by the date they were created. The function returns an optional array of `NSManagedObject`s, which can be cast to the appropriate type later.
Deleting Data
Let’s now delete a note:
```swift func delete(note: NSManagedObject) { let appDelegate = UIApplication.shared.delegate as! AppDelegate let context = appDelegate.persistentContainer.viewContext context.delete(note) do { try context.save() } catch { print("Failed deleting") } } ```
This function receives a `NSManagedObject` to delete. After deleting the note from the context, we save the context to persist the changes.
Conclusion
“Core Data offers robust and efficient data persistence in iOS. Its ability to manage complex object graphs, track changes, and handle relationships is unparalleled. With practice and understanding, both you and your team of hired iOS developers can make the most out of Core Data and build robust, data-driven iOS apps.
However, Core Data isn’t always the best tool for every job. If you need to share data across different platforms or prioritize read speed over complex object graph management, you, along with your skilled iOS developers, may want to consider alternatives like SQLite or Realm.
In the end, choose the technology that best fits your specific needs, and don’t be afraid to experiment. Whether you’re working alone or collaborating with expert iOS developers, the right approach to data persistence can make all the difference. Happy coding!”
Note: The example provided here is a simple, one-entity model. Core Data’s true power shines when dealing with complex models with multiple entities and relationships. Be sure to explore those aspects as well when you feel comfortable with the basics.
Table of Contents
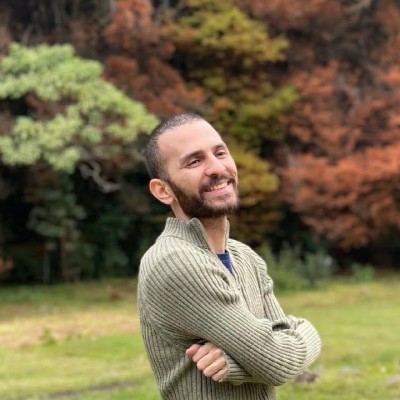
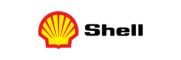