Exploring Core Graphics in iOS: Drawing and Rendering 2D Graphics
In the pulsating heart of iOS development, amidst the whirlwind of Swift and the majesty of SwiftUI, lies an unsung hero that has been part of Apple’s framework ensemble from the very start – Core Graphics. Often referred to by its affectionate nickname, Quartz 2D, this powerful, low-level C-based API is the go-to tool for drawing and rendering 2D graphics directly. Today, we’re diving deep into the world of Core Graphics, exploring its capabilities, and understanding how it stands as an essential tool for iOS developers looking to add that extra layer of polish and precision to their apps.
What is Core Graphics?
Core Graphics is a part of the Core Foundation framework, a collection of C interfaces and libraries that provide fundamental software services useful for application development. At its core (pun intended), Core Graphics deals with graphics rendering, including tasks such as drawing shapes, colors, images, and text. Think of it as the canvas and palette that allows developers to create detailed and complex graphics by coding.
Getting Started with Core Graphics
To embark on this graphical journey, you’ll need a basic understanding of iOS development and Swift. The first step is to import the Core Graphics framework into your project. This is done by including import CoreGraphics at the beginning of your Swift files where you’ll be doing the drawing.
Drawing Basics
The essence of drawing with Core Graphics revolves around contexts. A context in Core Graphics is essentially a canvas where you draw your graphics. This can be an off-screen buffer, a window, a PDF file, or any other drawable surface. Here’s a simple example of how to draw a line:
func drawLine(from fromPoint: CGPoint, to toPoint: CGPoint, context: CGContext) { context.beginPath() context.move(to: fromPoint) context.addLine(to: toPoint) context.setStrokeColor(UIColor.black.cgColor) context.strokePath() }
Advanced Drawing: Gradients and Patterns
Once you’ve mastered the basics, you can start exploring more complex techniques like gradients and patterns. Gradients can add depth and a sense of realism to your graphics, while patterns can bring texture and character.
Creating a gradient is straightforward with Core Graphics. You define the colors and locations, then draw the gradient in your context. Here’s a quick peek into creating a linear gradient:
func drawGradient(in rect: CGRect, context: CGContext) { let colorSpace = CGColorSpaceCreateDeviceRGB() let colors = [UIColor.red.cgColor, UIColor.blue.cgColor] as CFArray let gradient = CGGradient(colorsSpace: colorSpace, colors: colors, locations: [0.0, 1.0]) let startPoint = CGPoint(x: rect.midX, y: rect.minY) let endPoint = CGPoint(x: rect.midX, y: rect.maxY) context.drawLinearGradient(gradient!, start: startPoint, end: endPoint, options: []) }
Text and Fonts
Core Graphics also allows for precise control over text rendering, offering a range of options to customize font size, style, and layout. Drawing text involves setting the font and string attributes, then drawing the string to the context.
Performance Considerations
While Core Graphics is powerful, it operates at a low level, meaning that misuse can lead to performance bottlenecks. Always remember to:
- Minimize context state changes.
- Avoid unnecessary drawing.
- Use caching for complex drawings.
Core Graphics vs. SwiftUI
In the age of SwiftUI, you might wonder where Core Graphics fits in. SwiftUI provides a modern, high-level way to build user interfaces with less code, but when you need fine-grained control over your app’s graphics, Core Graphics is your tool. It’s about choosing the right tool for the job.
Learning Resources
To dive deeper into Core Graphics, check out these resources:
- Apple’s Core Graphics Guide: Directly from the source, this guide covers everything from basic concepts to advanced techniques. Visit Apple’s Developer Documentation.
- Ray Wenderlich’s Core Graphics Tutorials: For a more hands-on approach, Ray Wenderlich offers tutorials that range from beginner to advanced levels. Ray Wenderlich’s Website.
- Core Graphics on Stack Overflow: Sometimes, the best way to learn is by seeing how others solve their problems. Stack Overflow has a wealth of knowledge on Core Graphics. Stack Overflow.
Wrapping Up
Exploring Core Graphics is like peeling an onion. There are layers upon layers of complexity and capability, each more powerful and intricate than the last. Whether you’re looking to add subtle visual enhancements or create elaborate custom drawings, Core Graphics offers the tools you need to bring your creative visions to life. Dive into Core Graphics and elevate your iOS app’s visual appeal with mastery in drawing and rendering 2D graphics.
Happy coding!
Table of Contents
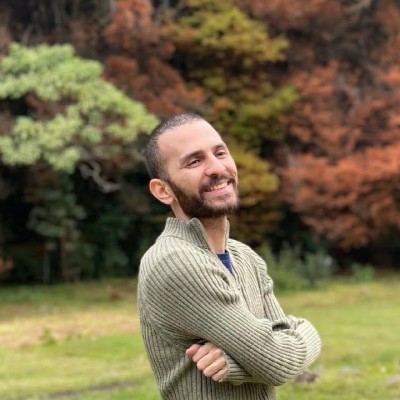
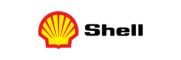