Exploring Core Location in iOS: Geocoding and Reverse Geocoding
Are you ready to unlock the power of location-based services in your iOS app? Look no further than Core Location, Apple’s framework that enables developers to integrate location awareness into their applications seamlessly. In this article, we’ll dive into two essential features of Core Location: geocoding and reverse geocoding.
Geocoding: Putting Locations on the Map
Geocoding is the process of converting a human-readable address into geographic coordinates, such as latitude and longitude. This functionality is invaluable for applications that need to display locations on a map or perform spatial queries.
Using Core Location’s geocoding capabilities is straightforward. You start by creating an instance of CLGeocoder, Apple’s class for converting between geographic coordinates and placemark information. Then, you use the geocodeAddressString(_:completionHandler:) method to perform the conversion asynchronously.
Here’s a basic example:
import CoreLocation let geocoder = CLGeocoder() geocoder.geocodeAddressString("1600 Amphitheatre Parkway, Mountain View, CA") { placemarks, error in guard let placemark = placemarks?.first else { print("Error: \(error?.localizedDescription ?? "Unknown error")") return } let location = placemark.location print("Latitude: \(location?.coordinate.latitude ?? 0), Longitude: \(location?.coordinate.longitude ?? 0)") }
In this example, we’re converting the address “1600 Amphitheatre Parkway, Mountain View, CA” into geographic coordinates. Once the geocoding operation completes, we extract the location from the resulting placemark and print out its latitude and longitude.
Reverse Geocoding: From Coordinates to Addresses
Reverse geocoding, on the other hand, involves converting geographic coordinates into human-readable addresses. This functionality is useful for applications that need to display location information to users or provide context based on their current position.
Core Location makes reverse geocoding just as easy as geocoding. You use the reverseGeocodeLocation(_:completionHandler:) method of CLGeocoder to perform the conversion asynchronously.
Here’s how you can perform reverse geocoding:
import CoreLocation let geocoder = CLGeocoder() let location = CLLocation(latitude: 37.7749, longitude: -122.4194) geocoder.reverseGeocodeLocation(location) { placemarks, error in guard let placemark = placemarks?.first else { print("Error: \(error?.localizedDescription ?? "Unknown error")") return } print("Address: \(placemark)") }
In this example, we’re converting the coordinates (latitude: 37.7749, longitude: -122.4194) into a human-readable address. Once the reverse geocoding operation completes, we extract the address information from the resulting placemark and print it out.
Real-World Applications
Now that you understand the basics of geocoding and reverse geocoding with Core Location, let’s explore some real-world applications:
- Mapping Apps: Geocoding allows mapping applications to translate addresses into map coordinates for display, while reverse geocoding enables them to provide detailed address information based on the user’s current location.
- Location-Based Services: Many apps utilize geocoding and reverse geocoding to offer location-based services, such as finding nearby restaurants, stores, or services.
- Weather Apps: Weather applications often use reverse geocoding to provide users with localized weather forecasts based on their current location.
Conclusion
Core Location’s geocoding and reverse geocoding capabilities open up a world of possibilities for iOS developers. Whether you’re building mapping applications, location-based services, or anything in between, understanding how to leverage these features will enhance the functionality and user experience of your app.
Start integrating Core Location into your iOS projects today and take advantage of its powerful location-based services.
External Resources:
- Apple Developer Documentation – Core Location
- Ray Wenderlich – Core Location Tutorial for iOS: Tracking Visited Locations
- Hacking with Swift – How to request a user’s location only when your app is running
By mastering Core Location, you’ll be able to create apps that navigate the world with ease and provide users with the seamless, location-aware experiences they crave. Happy coding!
Table of Contents
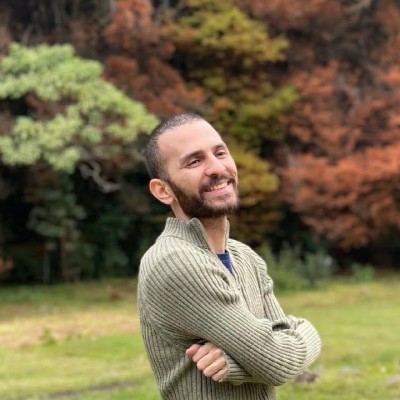
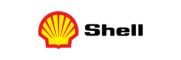