Introduction to Core NFC: Building Near Field Communication Apps
In today’s interconnected world, technology is constantly evolving to make our lives more convenient and efficient. One such technology that has gained prominence is Near Field Communication (NFC). NFC enables devices to communicate wirelessly when they are brought into close proximity, opening up a world of possibilities for various applications. From contactless payments and access control to data exchange between devices, NFC’s capabilities are vast. In this blog post, we’ll delve into the basics of Core NFC, Apple’s framework for integrating NFC capabilities into iOS apps. We’ll explore its features, benefits, and walk through code samples to understand how to build NFC-enabled applications.
Table of Contents
1. Understanding NFC Technology
Near Field Communication (NFC) is a short-range wireless technology that allows communication between devices when they are within a few centimeters of each other. It operates on the principles of radio-frequency identification (RFID) and is a subset of RFID technology. NFC technology is widely used in contactless payments, access control systems, public transportation cards, smart posters, and more.
NFC has two operating modes: active and passive. In active mode, both devices generate their own radio frequency to communicate. In passive mode, one device generates the radio frequency while the other device responds to it. This mode is often used in scenarios like tapping your phone on a payment terminal or scanning an NFC-enabled product.
2. Core NFC: An Overview
Core NFC is Apple’s framework that allows developers to integrate NFC capabilities into their iOS applications. It was introduced with iOS 11 and has since provided developers with the tools to interact with various types of NFC tags and devices. Core NFC supports reading NFC Data Exchange Format (NDEF) messages from compatible NFC tags. NDEF is a standardized format that defines how data should be stored on NFC tags and exchanged between devices.
3. Benefits of Core NFC Integration
Integrating Core NFC into your iOS app offers several benefits:
- Seamless User Experience: NFC enables quick and effortless interactions between devices, enhancing the overall user experience.
- Versatility: Core NFC supports a wide range of applications, including reading NFC tags embedded in products, posters, and more.
- Security: NFC communication can be secured using encryption and authentication, making it suitable for applications that require data privacy.
- Contactless Payments: Core NFC can be utilized for contactless payments, allowing users to make transactions by simply tapping their devices on payment terminals.
- Efficient Data Exchange: NFC provides a fast and efficient way to exchange data between devices, eliminating the need for complex setup processes.
4. Getting Started with Core NFC
To begin working with Core NFC, you’ll need a device with NFC capabilities and Xcode, Apple’s integrated development environment. Core NFC is available on iPhone 7 and later models. Here’s a step-by-step guide to get you started:
Step 1: Set Up Your Xcode Project
- Create a new Xcode project or open an existing one.
- In the project navigator, right-click on the folder where you want to add NFC functionality.
- Choose “Add Files to [Your Project Name]” and select the “NearField.framework” from the list.
Step 2: Request NFC Privileges
Since NFC involves interactions with user data, you need to request permission to access NFC capabilities. Open your app’s Info.plist file and add the “NFCReaderUsageDescription” key with a brief description of why your app needs NFC access.
xml <key>NFCReaderUsageDescription</key> <string>We need NFC access to read NFC tags for product information.</string>
Step 3: Import Core NFC
In your Swift code, import the Core NFC framework at the beginning of the file where you intend to work with NFC functionality.
swift import CoreNFC
5. Reading NFC Tags
Now that you’ve set up your Xcode project and imported Core NFC, let’s dive into reading NFC tags. The basic process involves setting up an NFC session, detecting NFC tags, and processing the data from the detected tags.
Step 1: Create an NFC Session
In your view controller or wherever you want to handle NFC interactions, create an instance of NFCTagReaderSession:
swift class NFCViewController: UIViewController, NFCTagReaderSessionDelegate { var nfcSession: NFCTagReaderSession? override func viewDidLoad() { super.viewDidLoad() // Do any additional setup after loading the view. } // Function to start NFC session func startNFCTagSession() { nfcSession = NFCTagReaderSession(pollingOption: .iso14443, delegate: self) nfcSession?.begin() } // Implement NFCTagReaderSessionDelegate methods here }
Step 2: Implement NFCTagReaderSessionDelegate
Conform to the NFCTagReaderSessionDelegate protocol to handle the detected NFC tags and their data:
swift extension NFCViewController: NFCTagReaderSessionDelegate { func tagReaderSessionDidBecomeActive(_ session: NFCTagReaderSession) { // Session started, handle UI updates if needed } func tagReaderSession(_ session: NFCTagReaderSession, didInvalidateWithError error: Error) { // Session invalidated, handle error } func tagReaderSession(_ session: NFCTagReaderSession, didDetect tags: [NFCTag]) { guard let tag = tags.first else { session.invalidate(errorMessage: "No NFC tag found.") return } // Handle the detected NFC tag session.connect(to: tag) { (error: Error?) in if error != nil { session.invalidate(errorMessage: "Error connecting to NFC tag.") return } // Read NFC tag data // Example: if let nfcNdefTag = tag as? NFCNDEFTag { ... } } } }
6. Processing NFC Tag Data
After detecting and connecting to an NFC tag, you can start processing its data. NFC tags often store data in NDEF message format. Here’s a basic example of how you can read and process NDEF data:
swift if let nfcNdefTag = tag as? NFCNDEFTag { nfcNdefTag.readNDEF { (ndefMessage: NFCNDEFMessage?, error: Error?) in if let error = error { print("Error reading NFC data: \(error.localizedDescription)") session.invalidate(errorMessage: "Error reading NFC data.") return } if let message = ndefMessage { for record in message.records { let payload = record.payload // Process the payload data } } // Finish processing NFC data session.invalidate() } }
Conclusion
Near Field Communication (NFC) technology has revolutionized the way we interact with devices and data. With Core NFC, Apple has provided iOS developers with the tools to harness the power of NFC and create innovative applications that enhance user experiences. From reading NFC tags to processing data, the possibilities are vast. By integrating Core NFC into your apps, you can unlock new opportunities for contactless payments, access control, and seamless data exchange.
In this blog post, we’ve only scratched the surface of what Core NFC can do. As you delve deeper into NFC app development, you’ll discover advanced features and use cases that can further elevate your applications. Whether you’re building an app for business, education, or entertainment, Core NFC opens doors to a world of possibilities at the tap of a screen. So, take the plunge into the realm of NFC and explore the endless opportunities it brings. Your next groundbreaking NFC-enabled app could be just a tap away!
Table of Contents
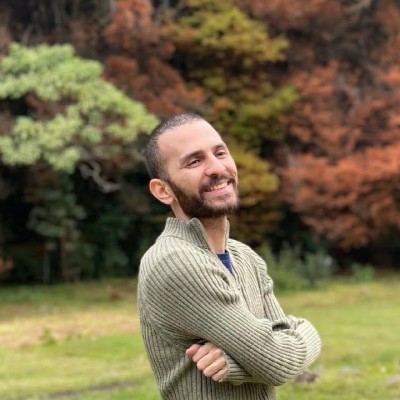
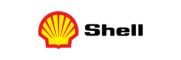