Working with Core Spotlight in iOS: Indexing User Activity for Search
In today’s digital age, where information overload is a common challenge, efficient search functionality within mobile applications is crucial. As an iOS developer, integrating robust search capabilities into your app not only enhances user experience but also enables users to quickly access the content they seek. One powerful tool for achieving this is Core Spotlight, a framework provided by Apple that allows you to index user activity and make it searchable within iOS.
What is Core Spotlight?
Core Spotlight is part of the Core Services framework in iOS and provides APIs for indexing and searching content within your app. It enables you to make specific items, such as documents, user activities, or other data, searchable by the system’s Spotlight search feature. This means that users can find content from your app directly through the iOS home screen search, even if they haven’t opened your app recently.
Indexing User Activity
One of the key features of Core Spotlight is its ability to index user activity within your app. This means that you can make user-generated content, interactions, or specific actions searchable through Spotlight. For example, if your app allows users to create notes, upload photos, or perform other activities, you can index these actions so that users can easily find them later.
Example 1: Note-Taking App
Imagine you’re developing a note-taking app where users can jot down ideas, to-dos, and reminders. By integrating Core Spotlight, you can index each note created by the user, along with relevant metadata such as title, content, and creation date. This allows users to search for specific notes directly from the iOS home screen, even if they have thousands of notes stored in your app.
Example 2: Fitness Tracking App
In a fitness tracking app, users may log their workouts, meals, and progress over time. By leveraging Core Spotlight, you can index each workout session or meal entry, making it easy for users to find past activities. Users can simply enter keywords related to their workouts, such as “morning run” or “leg day,” and relevant entries from your app will appear in the search results.
Integrating Core Spotlight into Your App
Now that you understand the concept of indexing user activity with Core Spotlight, let’s dive into how you can integrate it into your iOS app.
Step 1: Import the Core Spotlight Framework
Start by importing the Core Spotlight framework into your Xcode project. You can do this by adding the following import statement to your source files:
import CoreSpotlight
Step 2: Create CSSearchableItem Objects
Next, identify the user activities or content within your app that you want to make searchable. For each item, create a CSSearchableItem object and specify its attributes, such as title, content description, and unique identifier.
let item = CSSearchableItem(uniqueIdentifier: "note_\(note.id)", domainIdentifier: "com.example.notes", attributeSet: attributeSet)
Step 3: Index the Items
Once you’ve created the CSSearchableItem objects, you need to index them using the CSSearchableIndex class. This class provides methods for adding, updating, and removing items from the search index.
let index = CSSearchableIndex.default() index.indexSearchableItems([item]) { error in if let error = error { print("Error indexing item: \(error.localizedDescription)") } else { print("Item indexed successfully!") } }
Conclusion
Incorporating Core Spotlight into your iOS app can significantly improve the search experience for your users. By indexing user activity and making it searchable through Spotlight, you empower users to quickly find the content they need, whether it’s notes, workouts, or other interactions within your app.
External Resources:
Table of Contents
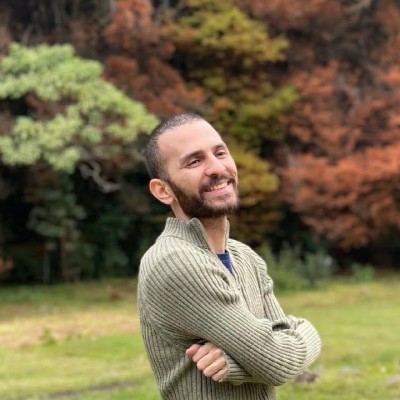
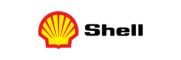