Creating Dynamic Type in iOS: Building Accessible Apps
In today’s digital world, accessibility is not just a nice-to-have feature; it’s a fundamental requirement for any app developer. As we strive to make our apps inclusive and usable by all, one crucial aspect to consider is text size. iOS offers a powerful feature called Dynamic Type that allows users to adjust text sizes according to their preferences. In this blog, we’ll delve into the world of Dynamic Type and learn how to create accessible iOS apps that adapt seamlessly to users’ text size preferences.
Table of Contents
1. Understanding Dynamic Type
Dynamic Type is an iOS feature that enables users to customize the size of text within apps. It’s a critical accessibility feature as it caters to users with varying visual abilities, making your app more inclusive and user-friendly. Users can adjust their preferred text size in the iOS Settings app under “Display & Brightness” > “Text Size.”
2. Advantages of Using Dynamic Type
Before we dive into the technical aspects of implementing Dynamic Type, let’s take a moment to understand why it’s essential and its benefits:
- Improved Accessibility: Dynamic Type ensures that all users, including those with visual impairments, can comfortably read text within your app.
- Enhanced User Experience: By allowing users to customize text size, you empower them to have a more personalized and enjoyable experience with your app.
- Future-Proofing: As users age or their visual needs change, Dynamic Type ensures your app remains usable and relevant to them.
- Compliance with Accessibility Standards: Adhering to accessibility standards is not only a moral imperative but also a legal requirement in many regions.
Now, let’s roll up our sleeves and explore how to implement Dynamic Type in your iOS app.
3. Implementing Dynamic Type
To implement Dynamic Type effectively, you’ll need to consider various elements within your app’s user interface, such as labels, buttons, and text views. Let’s break down the steps to make your app’s text adaptive to Dynamic Type changes.
3.1. Use Dynamic Type Fonts
Start by using Dynamic Type fonts for all textual content in your app. Dynamic Type fonts are system fonts that automatically adjust their size based on the user’s preferred text size setting. You can specify a Dynamic Type font by setting the UIFontTextStyle for your text.
swift let bodyFont = UIFont.preferredFont(forTextStyle: .body) label.font = bodyFont
In the above code snippet, we’re setting the label’s font to the system’s preferred font for body text. This ensures that the text size adapts as the user adjusts their Dynamic Type setting.
3.2. Set Font Scaling
While using Dynamic Type fonts is essential, it’s equally important to control how your app scales text when the user changes their text size preference. You can achieve this by setting the adjustsFontForContentSizeCategory property to true on your UI elements. This property allows the text to scale according to the user’s chosen text size while maintaining proper layout and spacing.
swift label.adjustsFontForContentSizeCategory = true
By enabling this property for all your text elements, you ensure that your app’s text remains legible and aesthetically pleasing at any size.
3.3. Test and Adjust
Once you’ve implemented Dynamic Type fonts and enabled font scaling, it’s crucial to test your app thoroughly. Try different text size settings in the iOS Settings app to ensure that your app’s text remains accessible and looks good at all sizes.
3.4. Custom Styles with Dynamic Type
In some cases, you may want to apply custom styling to your text while still supporting Dynamic Type. You can achieve this by using UIFontMetrics, which allows you to create a font with custom style traits that adapt to the user’s chosen text size.
swift let headlineFont = UIFont(name: "CustomFont-Bold", size: 24) let metrics = UIFontMetrics(forTextStyle: .headline) let scaledFont = metrics.scaledFont(for: headlineFont) label.font = scaledFont
In the code above, we’re creating a custom font for headlines and using UIFontMetrics to scale it based on the user’s Dynamic Type preference.
4. Best Practices for Dynamic Type
Implementing Dynamic Type is a significant step toward creating accessible iOS apps, but it’s essential to follow some best practices to ensure your app meets the highest standards of accessibility.
4.1. Avoid Hard-Coded Sizes
Never hard-code text sizes in your app. Always use system fonts and let Dynamic Type handle the sizing.
4.2. Maintain Adequate Spacing
Ensure that text elements have enough padding and spacing around them to accommodate larger text sizes without causing layout issues or overlapping.
4.3. Test with Real Users
Whenever possible, involve users with varying visual abilities to test your app’s accessibility. Their feedback can be invaluable in making improvements.
4.4. Consider Accessibility Labels
For non-textual elements like images and buttons, provide meaningful accessibility labels to assist users who rely on screen readers.
4.5. Stay Updated with Apple’s Guidelines
Keep an eye on Apple’s accessibility guidelines and updates to ensure your app remains compliant with the latest standards.
Conclusion
Creating Dynamic Type in iOS apps is a powerful way to make your applications more accessible and user-friendly. By implementing Dynamic Type fonts, enabling font scaling, and following best practices, you can ensure that users of all visual abilities can comfortably interact with your app.
Remember that accessibility is an ongoing effort. Continuously test and refine your app’s accessibility features, and always be open to user feedback. By prioritizing accessibility, you not only create a more inclusive app but also demonstrate your commitment to serving a diverse user base.
So, as you embark on your journey to build accessible iOS apps, embrace Dynamic Type, and help make the digital world a more inclusive place for everyone. Your efforts can make a significant difference in the lives of your users, and that’s something to be proud of. Happy coding!
Table of Contents
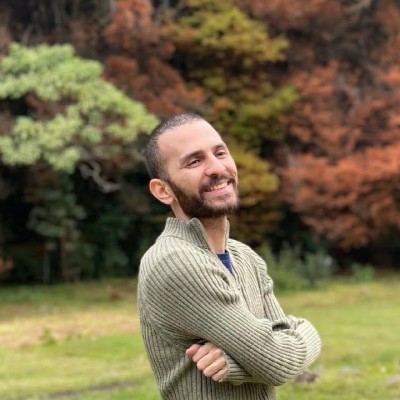
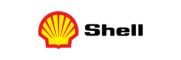