Creating Custom Transitions in iOS: UIViewControllerTransitioningDelegate
Are you tired of the same old transition animations in your iOS apps? Want to add a touch of creativity and uniqueness to your user experience? Look no further! In this guide, we’ll delve into the world of custom transitions in iOS using the powerful UIViewControllerTransitioningDelegate protocol. Buckle up as we explore how to take your app’s transitions from basic to breathtaking.
Understanding UIViewControllerTransitioningDelegate
Before we dive into creating custom transitions, let’s understand the role of UIViewControllerTransitioningDelegate. This protocol allows you to manage transitions between view controllers, giving you full control over the presentation and dismissal animations.
Getting Started
To begin, let’s create a custom transition class that conforms to the UIViewControllerAnimatedTransitioning protocol. This class will define the animation duration, as well as the animations themselves. Here’s a basic example:
class CustomTransition: NSObject, UIViewControllerAnimatedTransitioning { func transitionDuration(using transitionContext: UIViewControllerContextTransitioning?) -> TimeInterval { return 0.5 // Animation duration } func animateTransition(using transitionContext: UIViewControllerContextTransitioning) { // Implement your animation logic here } }
Implementing Custom Transitions
Now that we have our custom transition class, let’s integrate it into our view controller using the UIViewControllerTransitioningDelegate protocol. Here’s how you can do it:
class ViewController: UIViewController, UIViewControllerTransitioningDelegate { let customTransition = CustomTransition() override func viewDidLoad() { super.viewDidLoad() // Set transitioning delegate self.transitioningDelegate = self } // MARK: - UIViewControllerTransitioningDelegate func animationController(forPresented presented: UIViewController, presenting: UIViewController, source: UIViewController) -> UIViewControllerAnimatedTransitioning? { return customTransition } func animationController(forDismissed dismissed: UIViewController) -> UIViewControllerAnimatedTransitioning? { return customTransition } }
Adding Custom Animations
Now comes the fun part – adding your custom animations! You can manipulate view properties within the animateTransition(using:) method of your custom transition class to create stunning effects. Here’s a simple example of animating a view’s scale during presentation:
func animateTransition(using transitionContext: UIViewControllerContextTransitioning) { guard let fromView = transitionContext.view(forKey: .from), let toView = transitionContext.view(forKey: .to) else { return } let containerView = transitionContext.containerView containerView.addSubview(toView) toView.transform = CGAffineTransform(scaleX: 0.1, y: 0.1) UIView.animate(withDuration: transitionDuration(using: transitionContext), animations: { toView.transform = .identity }) { _ in transitionContext.completeTransition(!transitionContext.transitionWasCancelled) } }
Conclusion
Congratulations! You’ve just unlocked the power of custom transitions in iOS. With UIViewControllerTransitioningDelegate, you can elevate your app’s user experience to new heights. Experiment with different animations, get creative, and make your app stand out from the crowd.
External Resources
Ready to dive deeper into custom transitions? Check out these resources for further reading:
- Official Apple Documentation on Custom View Controller Presentations and Transitions
- Ray Wenderlich’s Tutorial on Custom View Controller Transitions
- Medium Article on Advanced iOS Animations: Custom View Controller Transitions
Now go forth and animate with confidence!
Happy coding!
Table of Contents
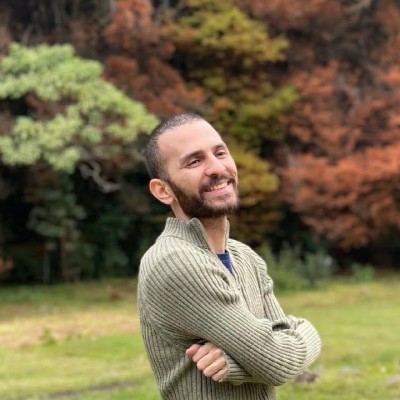
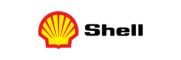