Getting Hands-On with Custom User Interface Development in iOS using Swift
Creating a custom user interface in iOS can be a delightful experience for both the developer and the end-user. Whether you’re an individual developer or a company looking to hire iOS developers, with the powerful Swift programming language and Apple’s frameworks such as UIKit and SwiftUI, you can design and build highly customized and interactive UI components. In this blog post, we’ll explore the basics of creating custom user interfaces in iOS with Swift, and we’ll also walk you through some practical examples. This guide will be invaluable to iOS developers or firms aiming to hire iOS developers, enhancing their understanding of UI customization.
Introduction to Swift and User Interface Frameworks
Swift, developed by Apple Inc., is a powerful and intuitive programming language for iOS, macOS, watchOS, and tvOS app development. It integrates seamlessly with Cocoa Touch, which is a UI framework for building software programs to run on iOS.
UIKit, a pivotal part of Cocoa Touch, provides the crucial infrastructure needed to construct and manage iOS apps. It includes a collection of user interface components like buttons, text fields, sliders, etc., which developers can use and customize.
SwiftUI, a more modern UI toolkit introduced in 2019, allows developers to design apps in a declarative way. With SwiftUI, you can create complex and custom UIs with less code. However, for the purpose of this blog, we’ll stick to UIKit, which offers a wider scope for customization.
Building a Custom UIButton
Let’s start with a fundamental example of customizing a UIButton. UIKit provides a simple way to create buttons, but often we need more than what the default UIButton offers. Let’s create a round button with a custom gradient background.
Here’s how to go about it:
```swift class GradientButton: UIButton { override init(frame: CGRect) { super.init(frame: frame) setupButton() } required init?(coder: NSCoder) { super.init(coder: coder) setupButton() } private func setupButton() { layer.cornerRadius = frame.size.height / 2 layer.masksToBounds = true setBackgroundColor() } private func setBackgroundColor() { let gradientLayer = CAGradientLayer() gradientLayer.frame = bounds gradientLayer.colors = [UIColor.red.cgColor, UIColor.blue.cgColor] gradientLayer.startPoint = CGPoint(x: 0, y: 0) gradientLayer.endPoint = CGPoint(x: 1, y: 1) layer.insertSublayer(gradientLayer, at: 0) } } ```
In the above code, we create a new class `GradientButton` that inherits from `UIButton`. In the `setupButton()` method, we set the `cornerRadius` to half of the button’s height to create a circular shape. We then call the `setBackgroundColor()` method to apply a gradient background color.
Custom UIView
Now, let’s create a custom UIView. Assume we need a view that shows a profile picture with a badge indicating online status. We’ll create a custom UIView that includes an UIImageView and a smaller UIView that will serve as the online status badge.
```swift class ProfileImageView: UIView { let imageView: UIImageView = { let iv = UIImageView() iv.translatesAutoresizingMaskIntoConstraints = false iv.contentMode = .scaleAspectFill iv.clipsToBounds = true return iv }() let statusView: UIView = { let view = UIView() view.translatesAutoresizingMaskIntoConstraints = false view.backgroundColor = UIColor.green return view }() override init(frame: CGRect) { super.init(frame: frame) setupView() } required init?(coder: NSCoder) { super.init(coder: coder) setupView() } private func setupView() { addSubview(imageView) addSubview(statusView) imageView.topAnchor.constraint(equalTo: topAnchor).isActive = true imageView.leadingAnchor.constraint(equalTo: leadingAnchor).isActive = true imageView.trailingAnchor.constraint(equalTo: trailingAnchor).isActive = true imageView.bottomAnchor.constraint(equalTo: bottomAnchor).isActive = true statusView.widthAnchor.constraint(equalToConstant: 20).isActive = true statusView.heightAnchor.constraint(equalToConstant: 20).isActive = true statusView.trailingAnchor.constraint(equalTo: trailingAnchor, constant: -10).isActive = true statusView.bottomAnchor.constraint(equalTo: bottomAnchor, constant: -10).isActive = true statusView.layer.cornerRadius = 10 statusView.clipsToBounds = true } } ```
In this example, we define an `UIImageView` and a `UIView`. We add them to the main view and set their constraints to position them appropriately. We also round the corners of the status view to make it circular.
Custom UITableView Cell
UITableView is a fundamental part of iOS development. Let’s say we need a custom cell for a chat application that shows the sender’s name, profile picture, message, and timestamp. Here’s how we can achieve this:
```swift class ChatTableViewCell: UITableViewCell { // Define UI components here let profileImageView: UIImageView = { // ... code }() let nameLabel: UILabel = { // ... code }() let messageLabel: UILabel = { // ... code }() let timestampLabel: UILabel = { // ... code }() override init(style: UITableViewCell.CellStyle, reuseIdentifier: String?) { super.init(style: style, reuseIdentifier: reuseIdentifier) setupCell() } required init?(coder: NSCoder) { super.init(coder: coder) setupCell() } private func setupCell() { // Add the UI components to the cell's contentView // Set constraints for positioning } } ```
We define the necessary UI components – an `UIImageView` and three `UILabels`. We add these to the cell’s `contentView` in the `setupCell()` method and set up their positioning using constraints.
Wrapping Up
In this blog post, we’ve delved into creating custom UI components in iOS using Swift and UIKit. These examples, while fundamental, form the basis of any custom UI development in iOS. Understanding these examples equips you, or the iOS developers you hire, with the skills to design and implement a broad array of custom user interfaces for your iOS applications.
While UIKit offers a rich set of tools for customization, SwiftUI, another toolkit, greatly simplifies the process of building UIs in a declarative way. Make sure to explore SwiftUI once you or your team of iOS developers are comfortable with UIKit and Swift.
The key to mastering UI customization, whether you’re an individual developer or a business looking to hire iOS developers, is practice. Attempt to recreate existing UIs or bring your unique designs to life, and never hesitate to experiment and explore. Happy coding!
Table of Contents
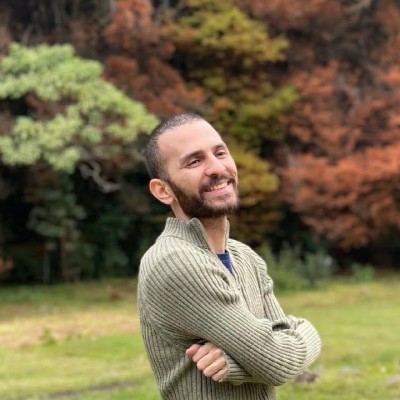
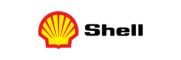