Exploring Core Motion in iOS: Accessing Device Motion and Sensor Data
In the dynamic world of iOS app development, understanding and harnessing the capabilities of device motion and sensor data is crucial for creating immersive and interactive experiences. With Core Motion, a powerful framework provided by Apple, developers can access a wealth of information about a device’s movement and orientation, enabling them to build innovative features and functionalities. In this guide, we’ll delve into the fundamentals of Core Motion and explore how to leverage it in your iOS applications.
Understanding Core Motion
At its core, Core Motion provides access to various motion-related data from the device’s hardware sensors, including accelerometer, gyroscope, and magnetometer. This framework offers a unified interface for accessing these sensors, simplifying the process of gathering motion data and integrating it into your app’s logic.
Accessing Device Motion Data
One of the key functionalities of Core Motion is the ability to retrieve real-time motion data from the device. By utilizing the CMMotionManager class, developers can easily access information such as acceleration, rotation rate, and orientation. Here’s a simple example demonstrating how to retrieve accelerometer data:
import CoreMotion let motionManager = CMMotionManager() if motionManager.isAccelerometerAvailable { motionManager.startAccelerometerUpdates(to: .main) { (data, error) in guard let acceleration = data?.acceleration else { return } // Process accelerometer data } }
In this code snippet, we create an instance of CMMotionManager and check if the accelerometer is available on the device. If it is, we start accelerometer updates and receive the data asynchronously in the closure.
Utilizing Sensor Fusion
Core Motion also supports sensor fusion, which combines data from multiple sensors to provide more accurate and robust motion information. By fusing data from the accelerometer, gyroscope, and magnetometer, developers can enhance the precision of motion tracking and enable features such as augmented reality and gesture recognition.
Implementing Device Orientation
Another useful aspect of Core Motion is its ability to determine the device’s orientation in space. By analyzing data from the accelerometer and gyroscope, developers can detect changes in orientation, including portrait, landscape, and face-up/face-down orientations. This information can be leveraged to adjust the app’s user interface or trigger specific actions based on device orientation changes.
Practical Applications
The capabilities of Core Motion open up a wide range of possibilities for iOS app development. From fitness and health tracking apps that monitor user movements to immersive gaming experiences that respond to device orientation, the potential applications are limitless. Here are a few examples of how Core Motion can be used in real-world scenarios:
- Fitness Tracking: Utilize accelerometer data to track steps, detect motion patterns, and provide personalized fitness insights to users.
- Augmented Reality: Combine sensor fusion techniques to create immersive AR experiences that respond to real-world movements and interactions.
- Gaming: Implement gesture recognition using gyroscopic data to enable intuitive controls and gameplay mechanics in mobile games.
External Resources
To further explore Core Motion and its capabilities, check out the following external resources:
- Apple Developer Documentation – Core Motion
- Ray Wenderlich – Core Motion Tutorial for iOS
- Hacking with Swift – Core Motion
Conclusion
In conclusion, Core Motion is a powerful framework for accessing device motion and sensor data in iOS development. By understanding its fundamentals and leveraging its features, developers can create immersive, interactive, and responsive experiences that delight users and push the boundaries of mobile app innovation. Whether you’re building fitness apps, AR experiences, or games, Core Motion provides the tools you need to bring your ideas to life on the iOS platform.
Table of Contents
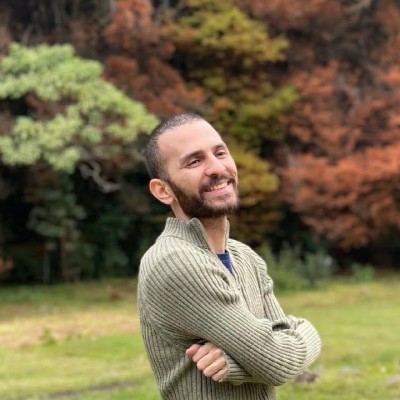
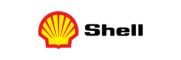