Implementing Dynamic Type in iOS: Adapting Text Size to User Preferences
In the realm of user experience, one size does not fit all. Every individual has unique preferences, especially when it comes to readability. Recognizing this, Apple introduced Dynamic Type, a feature that allows users to adjust the text size across iOS applications according to their comfort level. This not only enhances accessibility but also provides a more personalized experience for users. In this guide, we’ll delve into implementing Dynamic Type in iOS applications, exploring its benefits and providing practical examples.
Understanding Dynamic Type
Dynamic Type is a system-wide feature introduced by Apple that enables users to adjust the size of text displayed in supported iOS applications. It leverages the preferred text size specified in the device’s settings, allowing users to increase or decrease text size based on their comfort and accessibility needs. This feature is particularly beneficial for individuals with visual impairments or those who simply prefer larger or smaller text for better readability.
Benefits of Dynamic Type
- Enhanced Accessibility: By accommodating users with varying visual abilities, Dynamic Type ensures that your app is accessible to a wider audience. This inclusivity aligns with Apple’s commitment to creating products that are usable by everyone, regardless of their physical capabilities.
- Improved User Experience: Personalization is key to providing a superior user experience. With Dynamic Type, users can tailor the text size to their liking, resulting in a more comfortable and enjoyable interaction with your app. This customization fosters a sense of ownership and satisfaction among users.
- Compliance with Accessibility Standards: Adhering to accessibility standards not only makes your app more user-friendly but also helps you comply with regulations and guidelines such as the Web Content Accessibility Guidelines (WCAG). Implementing Dynamic Type demonstrates your commitment to accessibility and inclusivity.
Implementing Dynamic Type in iOS Applications
Implementing Dynamic Type in your iOS application is relatively straightforward and involves the following steps:
- Adopting Dynamic Type-Supported Text Styles: Utilize system-defined text styles such as UIFontTextStyle.body and UIFontTextStyle.headline for your text elements. These styles automatically adjust to the user’s preferred text size, ensuring consistency and compatibility with Dynamic Type.
- Setting Content Size Categories: Assign appropriate content size categories to your text elements based on their importance and relevance within your app’s interface. Apple provides predefined content size categories such as .large, .medium, and .small, allowing you to specify the scalability of text based on its context.
- Testing and Iteration: Thoroughly test your app’s text scalability across different content size categories and device configurations using the iOS Simulator and physical devices. Solicit feedback from users, particularly those with diverse accessibility needs, and iterate on your implementation to address any issues or concerns.
Example Implementation
import UIKit class ViewController: UIViewController { let titleLabel: UILabel = { let label = UILabel() label.font = UIFont.preferredFont(forTextStyle: .headline) label.text = "Welcome to Dynamic Type" label.translatesAutoresizingMaskIntoConstraints = false return label }() override func viewDidLoad() { super.viewDidLoad() view.addSubview(titleLabel) NSLayoutConstraint.activate([ titleLabel.centerXAnchor.constraint(equalTo: view.centerXAnchor), titleLabel.centerYAnchor.constraint(equalTo: view.centerYAnchor) ]) } override func traitCollectionDidChange(_ previousTraitCollection: UITraitCollection?) { super.traitCollectionDidChange(previousTraitCollection) // Update text styles based on dynamic type settings titleLabel.font = UIFont.preferredFont(forTextStyle: .headline) } }
Conclusion
Dynamic Type empowers users to customize their reading experience within iOS applications, fostering inclusivity and personalization. By implementing Dynamic Type support in your app, you not only enhance accessibility but also elevate the overall user experience. Embrace Dynamic Type as a fundamental aspect of iOS app development and prioritize the needs of all users, regardless of their visual abilities or preferences.
Further Reading:
Table of Contents
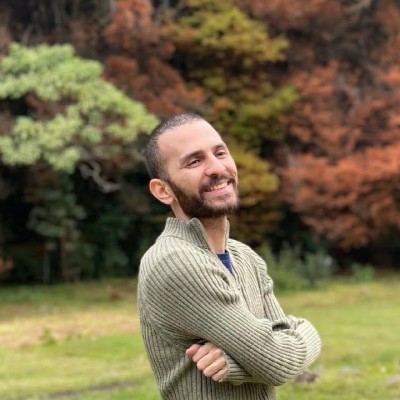
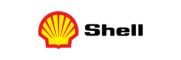