Essential Guide to Enhancing Performance in iOS Apps
As the iOS platform continues to evolve and mature, the performance optimization of iOS apps has become an imperative skill for every iOS developer. In today’s era of rapid technological advancement, users have little patience for slow, sluggish applications. Thus, it’s critical to ensure that your iOS applications deliver optimal performance.
Should you need assistance in enhancing your app performance, remember that there are professionals who can help. Hiring iOS developers with deep knowledge and expertise in performance optimization can be a great step forward.
This blog post will guide you through some of the best practices and techniques to optimize performance in iOS apps, complete with practical examples. These insights will be valuable whether you’re a seasoned iOS developer looking to fine-tune your skills, considering hiring iOS developers for additional support, or a novice eager to learn more about the field.
1. Efficient Use of Resources
Efficient resource management is a fundamental aspect of performance optimization. The first step in achieving this is minimizing the use of system resources. This means understanding how to work with data and ensuring that your app is not utilizing more memory than necessary.
For instance, if you’re using images in your app, it’s important to use them efficiently. Instead of loading high-resolution images that consume more memory, consider using optimized or compressed versions.
Example: Consider using the `UIImage` method `imageNamed:` that caches images and releases them when the system is running low on memory, rather than `imageWithContentsOfFile:` that doesn’t cache images and could lead to memory bloat.
```swift // Good let goodImage = UIImage(named: "example") // Bad let badImage = UIImage(contentsOfFile: "example") ```
2. Leverage Multithreading
iOS provides several technologies that allow you to harness the power of multi-core processors. This includes Grand Central Dispatch (GCD) and Operation Queues, which are high-level APIs for managing concurrent operations.
By using these technologies effectively, you can ensure that complex operations or tasks that don’t need to be completed immediately don’t block the main thread and cause your app to become unresponsive.
Example: Here is an example of using GCD for a time-consuming task such as downloading an image from the web.
```swift DispatchQueue.global(qos: .background).async { if let url = URL(string: "https://example.com/image.jpg"), let data = try? Data(contentsOf: url), let image = UIImage(data: data) { DispatchQueue.main.async { imageView.image = image } } } ```
In this example, the image download happens in the background, and the UI update happens on the main thread, preventing the UI from freezing during the download.
3. Efficient Networking
Networking operations often play a big role in the performance of iOS applications. It is vital to ensure the efficient use of network resources.
For example, consider using the HTTP/2 protocol, if possible, as it allows multiple simultaneous exchanges on the same connection, reducing latency. Also, cache network requests when possible to prevent unnecessary network calls.
Example: Using the `URLCache` class in iOS to cache network requests.
```swift let urlRequest = URLRequest(url: URL(string: "https://example.com")!) let config = URLSessionConfiguration.default config.urlCache = URLCache.shared config.requestCachePolicy = .returnCacheDataElseLoad let session = URLSession(configuration: config) ```
In this example, `URLSession` is configured to use a shared `URLCache` and a cache policy that returns cached data if available.
4. Use Instruments for Performance Analysis
Instruments is a powerful tool provided by Apple for performance analysis and visualization. It can help you understand where your app is spending time and resources.
Instruments can be used to track CPU usage, memory leaks, disk activity, and much more, enabling you to find bottlenecks and performance issues in your app.
Example: To use Instruments, follow these steps:
- Open Xcode, then choose “Product” from the menu bar, then “Profile.”
- Select the profiling template you need (e.g., Leaks, Time Profiler, etc.)
- Run your app within Instruments and interact with it to generate profiling data.
- Analyze the generated data to identify potential bottlenecks and optimize accordingly.
5. Avoid Blocking the Main Thread
The main thread is responsible for handling all user interface events in an iOS app. If the main thread gets blocked for any reason (like a lengthy task), the app’s UI will become unresponsive.
Thus, ensure that any time-consuming tasks such as network calls, database operations, image processing, etc., are performed in a background thread.
Example: Let’s say you need to perform a computationally heavy task such as filtering an array of large data. You can use GCD to move this operation off the main thread.
```swift DispatchQueue.global(qos: .userInitiated).async { let filteredData = heavyData.filter { ... } DispatchQueue.main.async { // Use filteredData to update UI } } ```
In this example, the heavy data filtering operation is performed in the background, and the main thread is only used when the operation is finished and the UI needs to be updated.
Conclusion
The optimization of iOS applications is a complex but essential aspect of development. Should you need professional assistance, consider the option to hire iOS developers who are well-versed in these best practices and techniques. They can provide a solid foundation for your project, focusing on the ultimate goal: creating a smooth, seamless user experience through an optimized, well-performing app. Happy coding!
Table of Contents
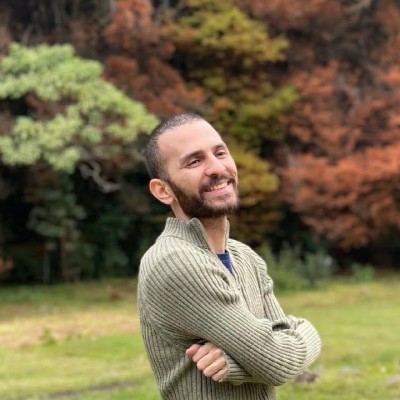
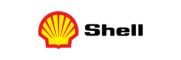