Exploring Core Video in iOS: Manipulating and Analyzing Video Content
Video content has become an integral part of our digital experience, from social media sharing to multimedia-rich apps. As an iOS developer, you may find yourself needing to manipulate and analyze video content in your applications. This is where Core Video, a powerful framework provided by Apple, comes into play. In this comprehensive guide, we will delve deep into Core Video, exploring its features and capabilities for handling video content in your iOS apps.
Table of Contents
1. Introduction to Core Video
1.1. What is Core Video?
Core Video is a framework provided by Apple that allows developers to manipulate, process, and analyze video content on iOS and macOS platforms. It’s part of the Core Media framework family and provides essential tools and APIs for working with video data efficiently. Core Video offers features like video frame rendering, pixel buffer manipulation, video composition, and video analysis.
1.2. Why Core Video?
As an iOS developer, you might wonder why you should bother with Core Video when there are high-level frameworks like AVFoundation available. The answer lies in the level of control and performance that Core Video offers. While AVFoundation is great for basic video playback and recording, Core Video empowers you to dig deeper, enabling you to process video frames at a granular level, apply custom video effects, and perform real-time video analysis.
1.3. Prerequisites
Before diving into Core Video, make sure you have the following prerequisites in place:
- Xcode installed on your macOS system.
- Basic knowledge of Swift programming.
- A development environment set up for iOS app development.
Now that you have a brief understanding of Core Video’s importance let’s move on to the practical aspects of using this powerful framework.
2. Getting Started
2.1. Creating a New Project
To get started with Core Video, create a new Xcode project or open an existing one where you want to integrate video manipulation or analysis features. Select the “App” template that best fits your project requirements, ensuring you choose the Swift programming language.
2.2. Importing Core Video
Core Video is part of the Core Media framework, so you’ll need to import it at the beginning of your Swift file where you plan to use it. Add the following import statement:
swift import CoreVideo
2.3. Basic Setup
Before you start manipulating or analyzing video content, you need to set up your project to handle video assets. Here are some essential steps:
- Import Video Assets: Add the video files you intend to work with to your Xcode project. Make sure they are included in the target you are building.
- Create a Video Player: If you want to display video content, you can use AVPlayer or AVPlayerViewController for playback. For more advanced video manipulation and analysis, continue with Core Video.
Now that you have set up your project, it’s time to dive into Core Video’s features.
3. Working with Video Buffers
Video processing in Core Video primarily revolves around the concept of a CVPixelBuffer, which represents the individual frames of a video. Understanding how to work with pixel buffers is fundamental to manipulating video content.
3.1. Understanding CVPixelBuffer
A CVPixelBuffer is a low-level representation of an image or video frame. It contains pixel data and information about the image format, such as color space, width, and height. Core Video uses pixel buffers to efficiently handle and process video frames.
To create a CVPixelBuffer from an image or video frame, you can use the following code snippet:
swift import CoreVideo func createPixelBuffer(from image: CGImage) -> CVPixelBuffer? { let width = image.width let height = image.height var pixelBuffer: CVPixelBuffer? let options: [String: Any] = [ kCVPixelBufferCGImageCompatibilityKey as String: true, kCVPixelBufferCGBitmapContextCompatibilityKey as String: true ] let status = CVPixelBufferCreate( kCFAllocatorDefault, width, height, kCVPixelFormatType_32ARGB, options as CFDictionary, &pixelBuffer ) guard status == kCVReturnSuccess, let buffer = pixelBuffer else { return nil } CVPixelBufferLockBaseAddress(buffer, []) let pixelData = CVPixelBufferGetBaseAddress(buffer) let colorSpace = CGColorSpaceCreateDeviceRGB() let context = CGContext( data: pixelData, width: width, height: height, bitsPerComponent: 8, bytesPerRow: CVPixelBufferGetBytesPerRow(buffer), space: colorSpace, bitmapInfo: CGImageAlphaInfo.noneSkipFirst.rawValue ) context?.draw(image, in: CGRect(x: 0, y: 0, width: width, height: height)) CVPixelBufferUnlockBaseAddress(buffer, []) return buffer }
This function takes a CGImage and creates a corresponding CVPixelBuffer. You can then use this pixel buffer for further processing.
3.2. Creating and Manipulating Pixel Buffers
Once you have a CVPixelBuffer, you can perform various operations on it, such as applying filters, resizing, or extracting pixel data. Here’s an example of how to resize a pixel buffer:
swift import CoreVideo import CoreImage func resizePixelBuffer(_ pixelBuffer: CVPixelBuffer, to size: CGSize) -> CVPixelBuffer? { let options: [String: Any] = [ kCVPixelBufferCGImageCompatibilityKey as String: true, kCVPixelBufferCGBitmapContextCompatibilityKey as String: true ] var resizedPixelBuffer: CVPixelBuffer? let status = CVPixelBufferCreate( kCFAllocatorDefault, Int(size.width), Int(size.height), kCVPixelFormatType_32ARGB, options as CFDictionary, &resizedPixelBuffer ) guard status == kCVReturnSuccess, let buffer = resizedPixelBuffer else { return nil } CVPixelBufferLockBaseAddress(buffer, []) let pixelData = CVPixelBufferGetBaseAddress(buffer) let context = CIContext() let image = CIImage(cvPixelBuffer: pixelBuffer) let scaledImage = image.transformed(by: CGAffineTransform(scaleX: size.width, y: size.height)) context.render( scaledImage, to: buffer, bounds: CGRect(origin: .zero, size: size), colorSpace: nil ) CVPixelBufferUnlockBaseAddress(buffer, []) return buffer }
In this example, we create a function that takes a pixel buffer and resizes it to a specified size using Core Image.
3.3. Converting Between Pixel Formats
Core Video provides functions to convert pixel buffers between different pixel formats. This can be useful when working with pixel data in various color spaces. Here’s a simple example of converting a pixel buffer to a different pixel format:
swift import CoreVideo func convertPixelBuffer(_ pixelBuffer: CVPixelBuffer, to pixelFormat: OSType) -> CVPixelBuffer? { let options: [String: Any] = [ kCVPixelBufferCGImageCompatibilityKey as String: true, kCVPixelBufferCGBitmapContextCompatibilityKey as String: true ] var convertedPixelBuffer: CVPixelBuffer? let status = CVPixelBufferCreate( kCFAllocatorDefault, CVPixelBufferGetWidth(pixelBuffer), CVPixelBufferGetHeight(pixelBuffer), pixelFormat, options as CFDictionary, &convertedPixelBuffer ) guard status == kCVReturnSuccess, let buffer = convertedPixelBuffer else { return nil } CVPixelBufferLockBaseAddress(buffer, []) let pixelData = CVPixelBufferGetBaseAddress(buffer) // Perform the conversion here, e.g., using Core Image or custom logic. CVPixelBufferUnlockBaseAddress(buffer, []) return buffer }
In this function, you can specify the desired pixel format (e.g., kCVPixelFormatType_32BGRA or kCVPixelFormatType_420YpCbCr8BiPlanarVideoRange) and perform the conversion accordingly.
These are just some of the basic operations you can perform on CVPixelBuffers. Depending on your specific use case, you can explore more advanced techniques, such as applying filters or transformations.
4. Video Composition
Video composition is the process of combining multiple video clips, adding overlays, effects, and creating a single output video. Core Video provides tools to achieve this efficiently.
4.1. Combining Multiple Videos
To combine multiple videos into one, you can use the AVMutableComposition class from AVFoundation in conjunction with Core Video. Here’s a high-level overview of the process:
- Create instances of AVAsset for each video clip you want to combine.
- Create an AVMutableComposition to represent the composition.
- Add the video tracks of each asset to the composition.
- Set the desired time range for each video track to determine when it appears in the final composition.
- Export the composition to a new video file.
Here’s an example of how you can combine two videos using Core Video:
swift import AVFoundation import CoreVideo func combineVideos(videoURLs: [URL], outputURL: URL) { // Create AVAssets for each video clip. let assets = videoURLs.map { AVAsset(url: $0) } // Create an AVMutableComposition for the composition. let composition = AVMutableComposition() // Add video tracks to the composition. for asset in assets { guard let videoTrack = asset.tracks(withMediaType: .video).first else { continue } let compositionTrack = composition.addMutableTrack( withMediaType: .video, preferredTrackID: kCMPersistentTrackID_Invalid ) do { try compositionTrack?.insertTimeRange( CMTimeRangeMake(start: .zero, duration: asset.duration), of: videoTrack, at: composition.duration ) } catch { print("Error inserting video track: \(error)") } } // Export the composition to a new video file. let exportSession = AVAssetExportSession( asset: composition, presetName: AVAssetExportPresetHighestQuality ) exportSession?.outputURL = outputURL exportSession?.outputFileType = .mp4 exportSession?.exportAsynchronously { if exportSession?.status == .completed { print("Video composition completed.") } else if let error = exportSession?.error { print("Video composition error: \(error)") } } }
In this example, we take an array of video URLs, combine them into a single composition, and export the result to a new video file.
4.2. Adding Overlays and Effects
Core Video allows you to add overlays and effects to video frames. You can create custom filters and apply them to individual frames or video tracks. One way to achieve this is by using the Core Image framework in combination with Core Video.
Here’s a simple example of adding a filter to a video using Core Image:
swift import AVFoundation import CoreImage import CoreVideo func applyFilterToVideo(inputURL: URL, outputURL: URL, filterName: String) { let asset = AVAsset(url: inputURL) let composition = AVMutableComposition() guard let videoTrack = asset.tracks(withMediaType: .video).first, let compositionTrack = composition.addMutableTrack( withMediaType: .video, preferredTrackID: kCMPersistentTrackID_Invalid ) else { return } do { try compositionTrack.insertTimeRange( CMTimeRangeMake(start: .zero, duration: asset.duration), of: videoTrack, at: .zero ) } catch { print("Error inserting video track: \(error)") return } // Create a CIFilter with the specified name. guard let filter = CIFilter(name: filterName) else { print("Filter not found.") return } // Apply the filter to the composition. compositionTrack.preferredTransform = videoTrack.preferredTransform compositionTrack.filterWith(filterName: filterName) // Export the composition with the filter applied. let exportSession = AVAssetExportSession( asset: composition, presetName: AVAssetExportPresetHighestQuality ) exportSession?.outputURL = outputURL exportSession?.outputFileType = .mp4 exportSession?.exportAsynchronously { if exportSession?.status == .completed { print("Filter applied and video exported.") } else if let error = exportSession?.error { print("Video composition error: \(error)") } } }
In this example, we create a function that applies a Core Image filter to a video and exports the result.
4.3. Exporting the Composite Video
After combining videos and applying any desired overlays or effects, you can export the composite video using an AVAssetExportSession. Set the output file URL and export options, then start the export session asynchronously. Monitor the export progress and handle any errors as needed.
5. Video Analysis
Core Video is not limited to video manipulation; it also enables you to analyze video content in various ways. Let’s explore some common video analysis tasks using Core Video.
5.1. Frame Extraction
Frame extraction involves capturing individual frames from a video for further analysis or processing. Core Video provides a straightforward way to accomplish this by using an AVAssetImageGenerator.
Here’s an example of how to extract frames from a video:
swift import AVFoundation import CoreVideo func extractFrames(from videoURL: URL, at intervals: [Double]) -> [CVPixelBuffer] { let asset = AVAsset(url: videoURL) let imageGenerator = AVAssetImageGenerator(asset: asset) var frames: [CVPixelBuffer] = [] for interval in intervals { let time = CMTime(seconds: interval, preferredTimescale: 600) do { let cgImage = try imageGenerator.copyCGImage(at: time, actualTime: nil) if let pixelBuffer = createPixelBuffer(from: cgImage) { frames.append(pixelBuffer) } } catch { print("Error extracting frame: \(error)") } } return frames }
In this example, we define a function that extracts frames from a video at specified time intervals and returns them as an array of CVPixelBuffers.
5.2. Motion Detection
Motion detection is a common video analysis task used in security systems, surveillance, and more. You can implement a simple motion detection algorithm using Core Video to compare consecutive frames and detect changes.
Here’s a basic example of motion detection:
swift import CoreVideo func detectMotion(between frame1: CVPixelBuffer, and frame2: CVPixelBuffer) -> Bool { // Implement your motion detection logic here. // Compare pixel data between the two frames and determine if there is motion. // For demonstration purposes, let's assume motion is always detected. return true }
In a real-world scenario, you would need to compare pixel data, possibly using techniques like frame differencing, thresholding, and image processing algorithms.
5.3. Face Detection
Face detection is a powerful video analysis task that can be implemented using Core Video in conjunction with other libraries like Vision or Core Image.
Here’s a high-level example of face detection using Core Video and Vision:
swift import AVFoundation import CoreVideo import Vision func detectFaces(in videoURL: URL) { let asset = AVAsset(url: videoURL) let videoTrack = asset.tracks(withMediaType: .video).first let request = VNDetectFaceRectanglesRequest() let handler = VNImageRequestHandler( cmSampleBuffer: createSampleBuffer(from: videoURL), orientation: CGImagePropertyOrientation.up, options: [:] ) do { try handler.perform([request]) guard let observations = request.results as? [VNFaceObservation] else { return } for observation in observations { // Process detected faces. // You can extract face coordinates, landmarks, and more. } } catch { print("Face detection error: \(error)") } }
In this example, we create a function that uses Vision to detect faces in a video. The detected face observations can then be processed further, such as drawing bounding boxes or extracting facial landmarks.
6. Performance Optimization
When working with video content, especially high-resolution videos, performance optimization is crucial to ensure smooth user experiences. Core Video offers several techniques for optimizing video processing tasks.
6.1. GPU Acceleration
Core Video takes full advantage of the GPU (Graphics Processing Unit) on iOS devices, which is well-suited for parallelizable tasks like video processing. When implementing video effects or analysis algorithms, consider using GPU-accelerated frameworks like Metal or Core Image to leverage the GPU’s processing power.
6.2. Memory Management
Efficient memory management is essential when working with video buffers. Always release pixel buffers and other resources when they are no longer needed to avoid memory leaks. You can use Swift’s automatic reference counting (ARC) to help manage memory, but be mindful of strong reference cycles in your code.
6.3. Real-time Processing
Real-time video processing often requires careful optimization to maintain a smooth frame rate. To achieve real-time performance, consider the following:
- Use background queues to perform time-consuming tasks like video analysis, so they don’t block the main thread.
- Profile and optimize your code to identify performance bottlenecks.
- Experiment with different video resolutions and frame rates to find the right balance between quality and performance for your application.
Conclusion
In this extensive guide, we’ve explored the capabilities of Core Video in iOS for manipulating and analyzing video content. We started with the basics, including setting up your project and working with video buffers. Then, we delved into video composition, adding overlays and effects, and exporting the resulting videos.
Furthermore, we ventured into video analysis tasks such as frame extraction, motion detection, and face detection, showcasing the versatility of Core Video in various applications. We concluded by emphasizing the importance of performance optimization when dealing with video content in your iOS applications.
Core Video opens up a world of possibilities for creating interactive and engaging video applications on iOS. As you continue to explore and experiment with Core Video, you’ll find endless opportunities to enhance user experiences and bring your creative video ideas to life.
Table of Contents
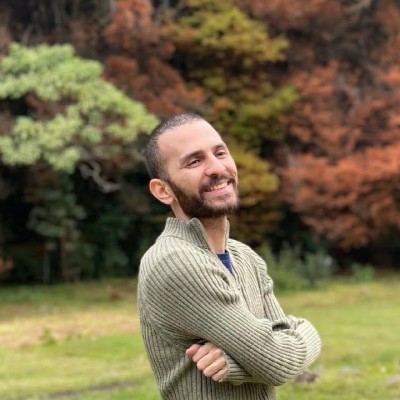
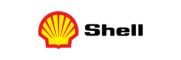